Mastering Data: Unlocking Hadoop HDFS Mysteries
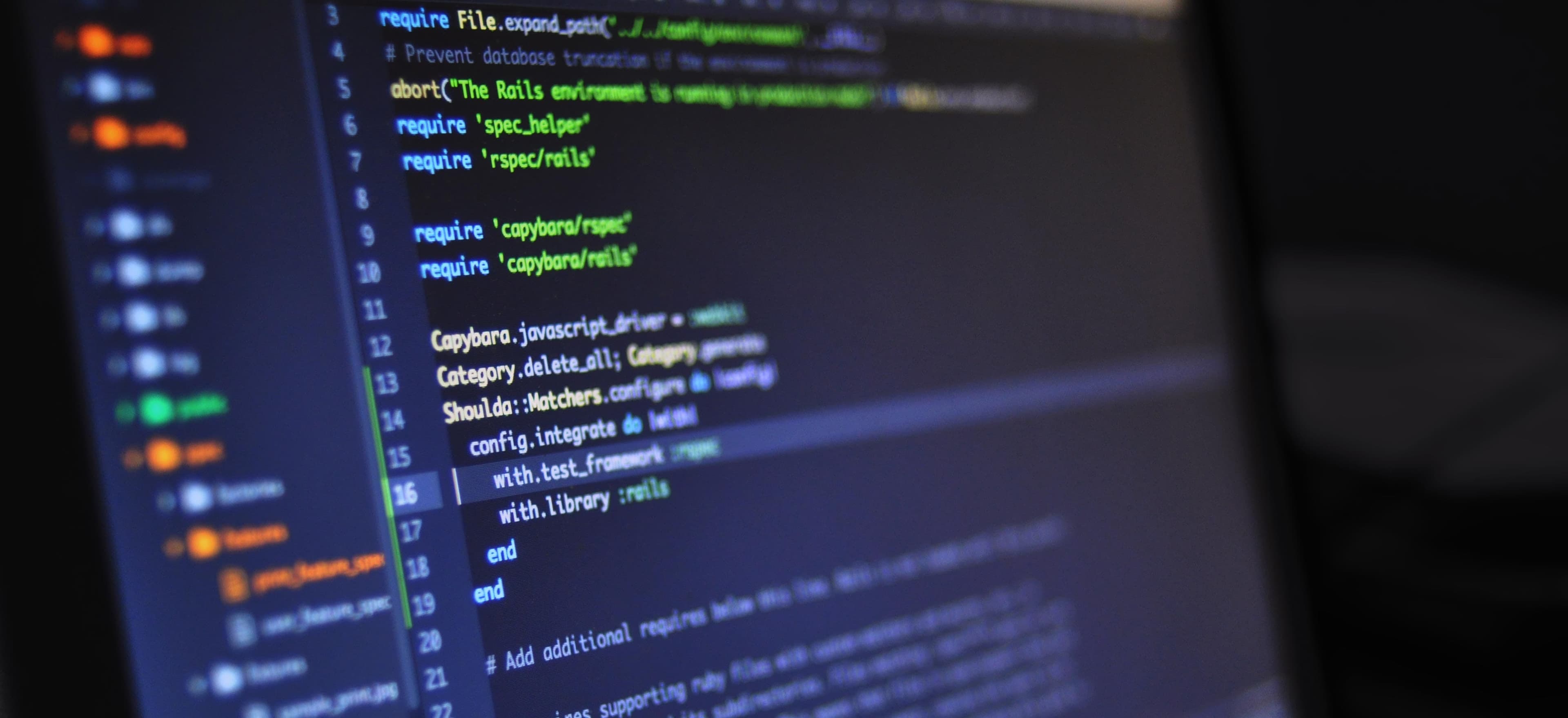
- Published on
Mastering Data: Unlocking Hadoop HDFS Mysteries
In the vast landscape of big data technologies, Hadoop stands as a giant. At the core of Hadoop lies the Hadoop Distributed File System (HDFS), a key component for storing and managing vast amounts of data across clusters of commodity hardware. In this article, we will delve into the enigmatic world of HDFS and explore how Java, with its robust features and vibrant ecosystem, can be harnessed to interact with and manipulate data within HDFS.
Understanding Hadoop HDFS
Before we embark on our Java-centric journey, it's imperative to grasp the fundamental principles of HDFS. HDFS is a distributed file system designed to run on commodity hardware. It boasts high fault tolerance and is well-equipped to handle large-scale data storage, making it a cornerstone of big data applications.
HDFS Architecture
HDFS comprises two primary components: the NameNode and DataNode. The NameNode is responsible for managing the metadata, while the DataNode stores the actual data. The architecture's distributed nature allows for reliability and scalability, thereby enabling HDFS to accommodate the exponential growth of data in modern enterprises.
Java and HDFS
Java, renowned for its portability and extensive libraries, seamlessly integrates with Hadoop to empower developers in building robust and scalable solutions. Leveraging the Hadoop HDFS Java API, developers can harness the power of HDFS to read, write, and manipulate data stored within the system.
Interacting with HDFS Using Java
Setting Up the Development Environment
To begin our journey with Java and HDFS, it's essential to set up a development environment that includes the Hadoop libraries. Maven, a popular build automation tool, simplifies this process by managing dependencies efficiently. Ensure that the following Maven dependency for Hadoop is included in the project's pom.xml
file:
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-client</artifactId>
<version>3.3.0</version>
</dependency>
Writing to HDFS
Let's kick off our exploration by writing a simple Java program to create a new file in HDFS. First, we need to establish a connection to the HDFS cluster and obtain an instance of the FileSystem
:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class HdfsWriter {
public static void main(String[] args) {
Configuration conf = new Configuration();
try {
FileSystem fs = FileSystem.get(conf);
String content = "Hello, HDFS!";
byte[] data = content.getBytes();
Path filePath = new Path("/user/hadoop/sample.txt");
fs.create(filePath).write(data);
fs.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this example, we create a FileSystem
instance using the Hadoop configuration. We then define the content to be written, convert it to bytes, specify the file path in HDFS, create the file, and finally close the file system.
Reading from HDFS
Next, let's dive into reading a file from HDFS using Java. Here's a simple program to achieve this:
import org.apache.hadoop.fs.FSDataInputStream;
import org.apache.hadoop.fs.Path;
public class HdfsReader {
public static void main(String[] args) {
Configuration conf = new Configuration();
try {
FileSystem fs = FileSystem.get(conf);
Path filePath = new Path("/user/hadoop/sample.txt");
FSDataInputStream inputStream = fs.open(filePath);
byte[] data = new byte[1024];
inputStream.read(data);
System.out.println(new String(data).trim());
inputStream.close();
fs.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this snippet, we retrieve an FSDataInputStream
for the file in HDFS, read its content into a byte array, convert it back to a string, and print it to the console.
Handling File Operations
Java offers a plethora of capabilities for handling file operations within HDFS. Whether it's deleting files, checking file existence, or even modifying file permissions, Java provides a robust interface to interact with HDFS programmatically.
import org.apache.hadoop.fs.Path;
public class HdfsFileOperations {
public static void main(String[] args) {
Configuration conf = new Configuration();
try {
FileSystem fs = FileSystem.get(conf);
Path filePath = new Path("/user/hadoop/sample.txt");
// Deleting a file
boolean isDeleted = fs.delete(filePath, false);
System.out.println("File deleted: " + isDeleted);
// Checking file existence
boolean exists = fs.exists(filePath);
System.out.println("File exists: " + exists);
// Modifying file permissions
fs.setPermission(filePath, new FsPermission(FsAction.READ_WRITE, FsAction.READ, FsAction.NONE));
fs.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this snippet, we illustrate deleting a file, checking file existence, and modifying file permissions in HDFS using Java.
My Closing Thoughts on the Matter
In conclusion, mastering Hadoop HDFS with Java opens a gateway to harness the full potential of big data. From writing and reading data to performing intricate file operations, Java equips developers with the tools to elegantly interface with HDFS. By seamlessly blending the robust features of Java and the scalability of HDFS, developers can conquer the challenges posed by colossal data volumes and unlock the mysteries of distributed data storage and processing.
Embark on your journey to conquer HDFS with Java, and witness how the fusion of two powerful technologies paves the way for unlocking the true potential of big data.
Remember, the key to mastery lies in relentless practice and a thirst for knowledge in the ever-evolving realm of big data technologies. Happy Hadooping with Java!