Spring Boot on Cloud Foundry: Solving Binding Hurdles
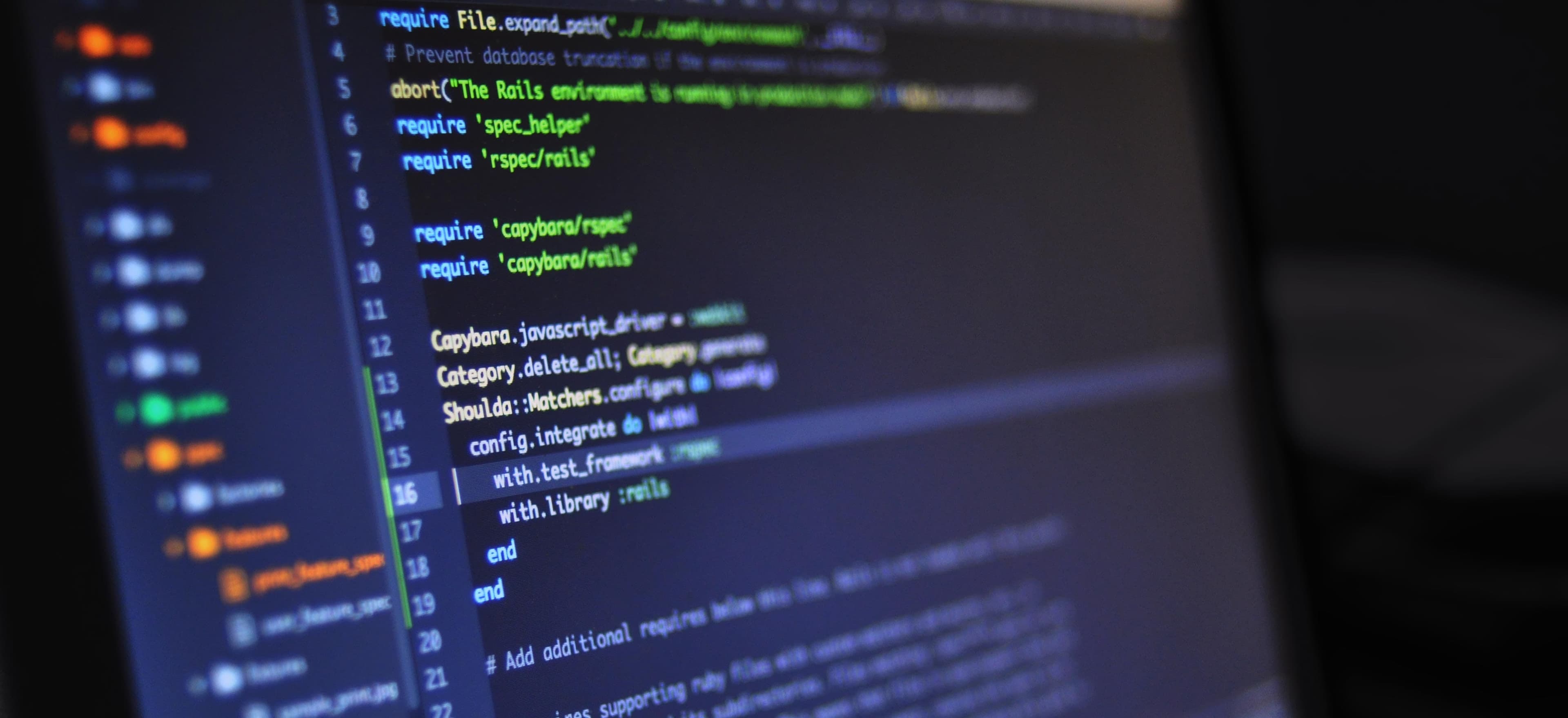
- Published on
Spring Boot on Cloud Foundry: Solving Binding Hurdles
In the age of microservices and cloud-native development, Spring Boot and Cloud Foundry have become indispensable tools for developers. However, deploying Spring Boot applications to Cloud Foundry, especially when it comes to service binding, presents a significant challenge. This article will delve into the concept of service binding in Cloud Foundry, the hurdles developers face with Spring Boot applications, and various solutions to overcome these obstacles.
The Approach
Spring Boot and Cloud Foundry are pivotal to modern microservices architecture and cloud-native application development. Spring Boot simplifies the creation of stand-alone, production-grade Spring-based applications, while Cloud Foundry is an open-source cloud app platform that provides a faster and easier way to build, test, deploy, and scale applications. Despite their advantages, one common challenge developers encounter when deploying Spring Boot applications to Cloud Foundry is service binding.
Service binding in Cloud Foundry allows applications to connect to services such as databases, message brokers, and external APIs. It simplifies application configuration and management by providing a central mechanism for securely and dynamically connecting services to applications. However, binding services to Spring Boot applications on Cloud Foundry presents specific challenges related to environment variables, service discovery, and configuration properties, which need to be resolved for seamless application deployment and operation.
What is Service Binding in Cloud Foundry?
In Cloud Foundry, service binding enables applications to access and use the services available in the platform. This includes databases, message brokers, and various other types of backing services that are provided as part of the platform or by third-party vendors. When a service is bound to an application, Cloud Foundry provides the application with all the necessary connection details and credentials required to communicate with the service.
One key benefit of service binding is that it simplifies application configuration and management. Developers don't need to explicitly define connection details within the application code or configuration files. Instead, the platform dynamically provides the necessary environment variables and connection information at runtime, making the process of adding, updating, or removing services seamless and straightforward for the deployed applications.
The Challenge with Spring Boot and Cloud Foundry Binding
When it comes to binding services to Spring Boot applications on Cloud Foundry, developers face several challenges. One major issue is related to accessing and utilizing environment variables specific to Cloud Foundry within the Spring Boot application. Additionally, service discovery and configuration properties often pose hurdles, requiring a deeper understanding of how Spring Boot applications interact with the Cloud Foundry platform.
This challenge is crucial to address as it directly impacts the ability to seamlessly deploy, connect, and manage Spring Boot applications in a Cloud Foundry environment. Without effectively resolving these binding issues, the deployment and operation of applications can become complex, error-prone, and challenging to maintain.
Solutions to Spring Boot and Cloud Foundry Binding Hurdles
Understanding Cloud Foundry Environment Variables
To access and utilize Cloud Foundry environment variables within a Spring Boot application, developers can leverage the VCAP_SERVICES environment variable. This variable contains the connection details for all bound services, including information required to connect to databases, message brokers, and more.
Here’s a sample code snippet demonstrating how to retrieve database credentials from Cloud Foundry's VCAP_SERVICES environment variable:
@Configuration
public class DatabaseConfig {
@Bean
public DataSource dataSource() {
// Access the VCAP_SERVICES environment variable to retrieve the database credentials
String vcapServices = System.getenv("VCAP_SERVICES");
// Extract the necessary database connection details
// ...
// Create and return the DataSource using the retrieved credentials
// ...
}
}
In this code snippet, the DatabaseConfig
class demonstrates how to access the VCAP_SERVICES environment variable to retrieve the required database credentials. This allows the Spring Boot application to dynamically obtain the database connection information at runtime, simplifying the process of service binding.
Utilizing Spring Cloud Connectors
Spring Cloud Connectors is a library that simplifies connecting to services, like databases and message brokers, in Cloud Foundry environments. It provides a convenient way to abstract away the complexity of service binding, making it easier for developers to integrate Cloud Foundry services with their Spring Boot applications.
Here’s an example of using Spring Cloud Connectors to connect to a database service in a Cloud Foundry environment:
@Configuration
public class DatabaseConfig {
@Bean
public DataSource dataSource(Cloud cloud) {
// Retrieve the bound database service using Spring Cloud Connectors
DataSource dataSource = cloud.getServiceConnector("my-db-service", DataSource.class, null);
// Return the DataSource for the bound database service
return dataSource;
}
}
In this code snippet, the DatabaseConfig
class demonstrates how to use Spring Cloud Connectors to retrieve and connect to a bound database service. By leveraging Spring Cloud Connectors, developers can simplify the process of connecting Spring Boot applications to Cloud Foundry services, reducing the complexity associated with service binding.
Custom Configuration for Complex Service Bindings
In cases where auto-configuration or Spring Cloud Connectors aren't sufficient, developers may need to create custom configuration classes within their Spring Boot applications to handle more complex service binding scenarios. This involves creating specific configuration classes tailored to the requirements of the bound services.
For example, when dealing with a complex message broker service, developers can create a custom configuration class to manage the intricacies of connecting to and interacting with the service:
@Configuration
public class MessageBrokerConfig {
@Bean
public MessageBrokerService messageBrokerService() {
// Custom logic to connect and interact with the complex message broker service
// ...
}
}
By creating custom configuration classes, developers can address complex service binding scenarios that aren't straightforwardly handled by Spring Boot auto-configuration or Spring Cloud Connectors. This approach provides the flexibility to tailor the service binding process based on specific requirements and interactions with the bound services.
Best Practices for Service Binding in Cloud Foundry
To ensure effective service binding when deploying Spring Boot applications on Cloud Foundry, here are some best practices to consider:
- Security: Always handle and store service credentials securely.
- Maintenance: Regularly review and update service bindings to ensure they align with the evolving application requirements and service offerings.
- Scalability: Design service bindings to be scalable, allowing applications to seamlessly connect to and utilize additional services as the demand grows.
By following these best practices, developers can enhance the security, maintainability, and scalability of their Spring Boot applications when integrating with services in a Cloud Foundry environment.
Final Considerations
Efficiently handling service bindings in Cloud Foundry is essential for seamlessly deploying and managing Spring Boot applications. By understanding the challenges, solutions, and best practices associated with service binding, developers can overcome the hurdles and make the most of the capabilities offered by Spring Boot and Cloud Foundry in the context of modern cloud-native application development.
As developers continue to explore the integration of Spring Boot applications with Cloud Foundry services, it's crucial to share experiences and insights. Whether it's addressing specific challenges or discovering innovative solutions, the community's collective knowledge can significantly benefit the development and deployment of Spring Boot applications on Cloud Foundry.
Additional Resources
For further exploration of Spring Boot, Cloud Foundry, and service binding, consider the following resources:
These resources offer in-depth information, tutorials, and community support to aid developers in their journey of deploying Spring Boot applications on Cloud Foundry and effectively managing service bindings.
In conclusion, the efficient integration of Spring Boot and Cloud Foundry presents developers with the opportunity to overcome service binding challenges and create robust, scalable applications in the cloud-native ecosystem. By embracing best practices and leveraging the solutions provided, developers can ensure the smooth operation and maintenance of their Spring Boot applications deployed on Cloud Foundry.
Call to Action: We encourage readers to share their experiences and insights regarding deploying Spring Boot applications on Cloud Foundry in the comments section below. Whether you've encountered specific challenges or discovered innovative solutions, your contributions are invaluable to the community.
Disclosure: This blog post may contain external affiliate links that can result in us receiving a commission if you choose to purchase mentioned products. However, our editorial content is not influenced by advertisers or affiliate partnerships.
SEO Tips for the Blog Post:
- Incorporate relevant keywords like "Spring Boot," "Cloud Foundry," and "service binding" naturally throughout the article to optimize search visibility.
- Ensure engaging titles and subtitles that include primary keywords for better SEO performance.
- Provide internal and external links to high-quality sources related to Spring Boot and Cloud Foundry to improve the post's authority and relevance.
- Optimize any included images with relevant alt text containing primary keywords to enhance SEO ranking.
- Encourage user engagement by ending with a strong call to action that prompts interaction and comments.
- Utilize structured formatting, bullet points, and numbered lists to enhance readability and SEO ranking.