Avoiding Java DateFormat Pitfalls: Best Practices Unveiled
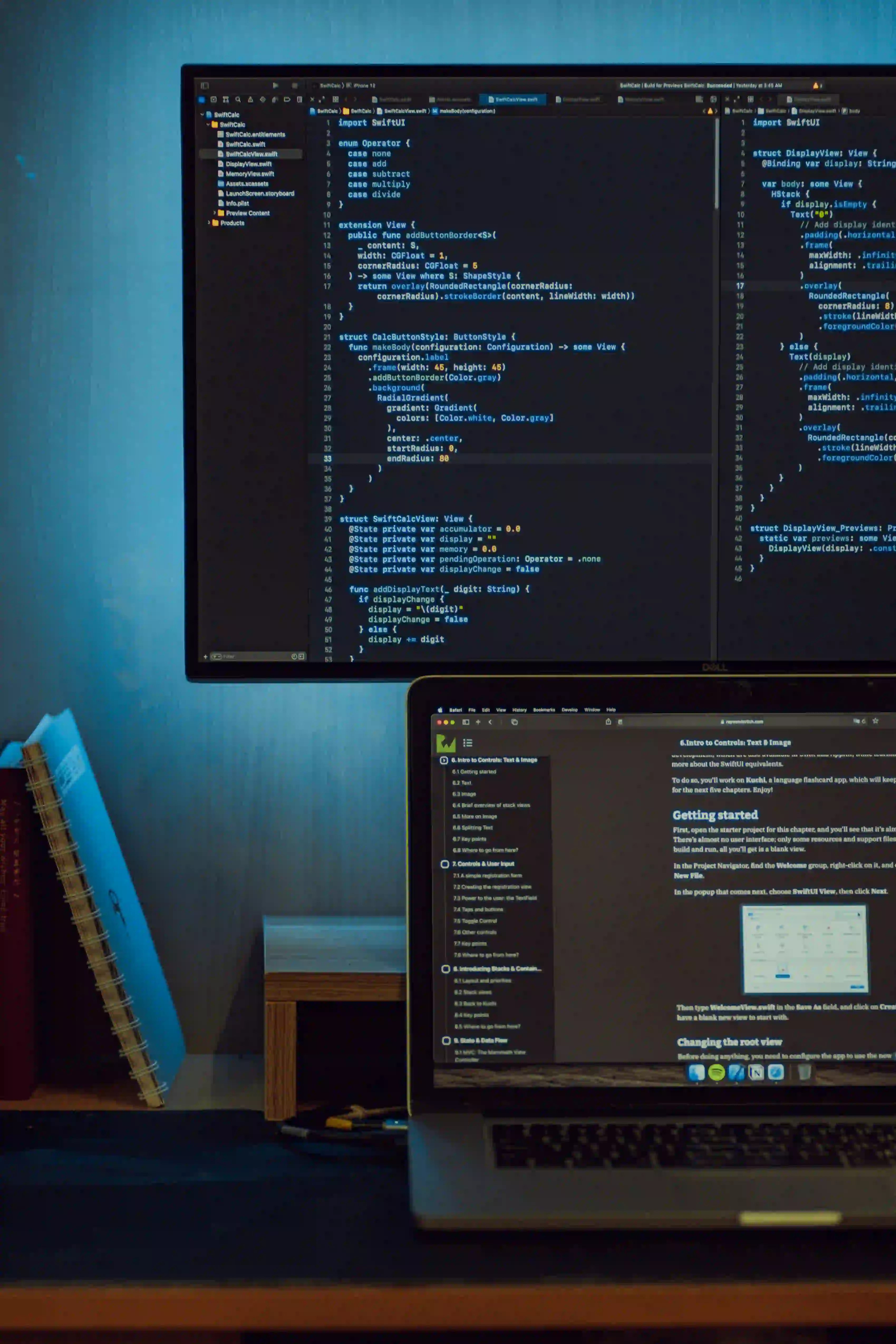
Avoiding Java DateFormat Pitfalls: Best Practices Unveiled
If you've ever worked with dates and times in Java, you know how tricky it can be to handle them correctly. The DateFormat
class in Java is a powerful tool for parsing and formatting dates, but it comes with its own set of pitfalls that can trip up even experienced developers. In this post, we'll explore some common DateFormat pitfalls and discuss best practices for avoiding them.
Pitfall 1: Thread Safety
One of the most common DateFormat pitfalls is the lack of thread safety. The DateFormat
class is not thread-safe, meaning that it can cause unexpected behavior when used in a multi-threaded environment. This is because the DateFormat
class stores internal state related to the date and time formatting, and when multiple threads are using the same DateFormat
instance, they can interfere with each other.
Best Practice: Use ThreadLocal or Create a New Instance
To avoid thread safety issues, it's best to use a new instance of SimpleDateFormat
for each thread, or utilize ThreadLocal
to provide a separate instance of SimpleDateFormat
for each thread. This ensures that each thread has its own copy of the DateFormat
and avoids any potential conflicts.
private static final ThreadLocal<DateFormat> threadLocalDateFormat = ThreadLocal.withInitial(() ->
new SimpleDateFormat("yyyy-MM-dd"));
This way, each thread gets its own DateFormat
instance, preventing any thread safety issues.
Pitfall 2: Locale Sensitivity
Another common pitfall when working with DateFormat
is the handling of different locales. When parsing or formatting dates, the default behavior of DateFormat
depends on the system's default locale, which may lead to inconsistencies when the code runs on different systems with different default locales.
Best Practice: Always Specify the Locale
To avoid issues related to locale sensitivity, it's crucial to always specify the locale when working with SimpleDateFormat
or any other date formatting classes. This ensures that the date parsing and formatting are consistent regardless of the system's default locale.
SimpleDateFormat sdf = new SimpleDateFormat("dd-MMM-yyyy", Locale.US);
By specifying the locale explicitly, you can ensure consistent behavior across different environments.
Pitfall 3: Date Parsing and Formatting Patterns
Incorrect date parsing and formatting patterns can lead to unexpected results or runtime errors. It's easy to make mistakes when defining the patterns for parsing or formatting dates, especially when dealing with custom date formats.
Best Practice: Understand Date Pattern Symbols
When working with date parsing and formatting, it's crucial to understand the pattern symbols used by SimpleDateFormat
. The symbols used to define date and time patterns are case-sensitive and have specific meanings. For example, "M" represents the month, "d" represents the day of the month, "y" represents the year, and so on.
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
By using the correct pattern symbols, you can ensure that the date parsing and formatting behaves as expected.
Pitfall 4: Handling Time Zones
Dealing with time zones can be a complex task when working with dates and times. Incorrectly handling time zones can lead to subtle bugs and inconsistencies in your date handling code.
Best Practice: Use Time Zone Aware Classes
When working with dates and times that involve time zones, it's best to use time zone aware classes such as ZonedDateTime
or OffsetDateTime
introduced in Java 8. These classes provide better support for time zone handling, daylight saving time, and other time-related adjustments.
ZonedDateTime now = ZonedDateTime.now(ZoneId.of("America/New_York"));
Using time zone aware classes can help you avoid common pitfalls related to time zone handling.
Pitfall 5: Deprecated Methods
With the evolution of Java and the introduction of newer APIs, some of the methods in the DateFormat
class have been deprecated. Using deprecated methods can lead to compatibility issues and reduced maintainability of your code.
Best Practice: Use Java Time API
To ensure future compatibility and better support for date and time handling, it's recommended to use the Java Time API introduced in Java 8 and later versions. The java.time
package provides a modern date and time API that resolves many issues found in the old java.util.Date
and SimpleDateFormat
.
LocalDate today = LocalDate.now();
By utilizing the Java Time API, you can future-proof your code and avoid using deprecated methods.
Key Takeaways
In conclusion, working with dates and times in Java requires careful attention to avoid common pitfalls related to DateFormat
. By following best practices such as ensuring thread safety, specifying locales, understanding date pattern symbols, handling time zones properly, and utilizing modern time-related APIs, you can write robust and reliable date handling code in Java.
Remember, mastering DateFormat
in Java is crucial for building dependable applications that handle date and time operations accurately. By acknowledging and understanding these best practices, you are taking a significant step towards writing well-crafted and bug-free Java code.
Don't let date handling trip you up - equip yourself with these best practices and ensure your Java applications handle dates and times with precision and finesse.
To delve deeper into modern date and time handling in Java, consider exploring the Oracle's Java Documentation. This resource provides comprehensive insights into the Java Time API, offering detailed information, examples, and best practices for effective date and time manipulation in Java.
For a practical application of Java date handling, you may also find the java.text.DateFormat
Class Documentation on Oracle's Official Website informative. This resource offers detailed explanations of the DateFormat
class and its methods, enabling you to deepen your understanding of its functionality.