Streamline Your Linux Dev Setup: Avoid Common Pitfalls
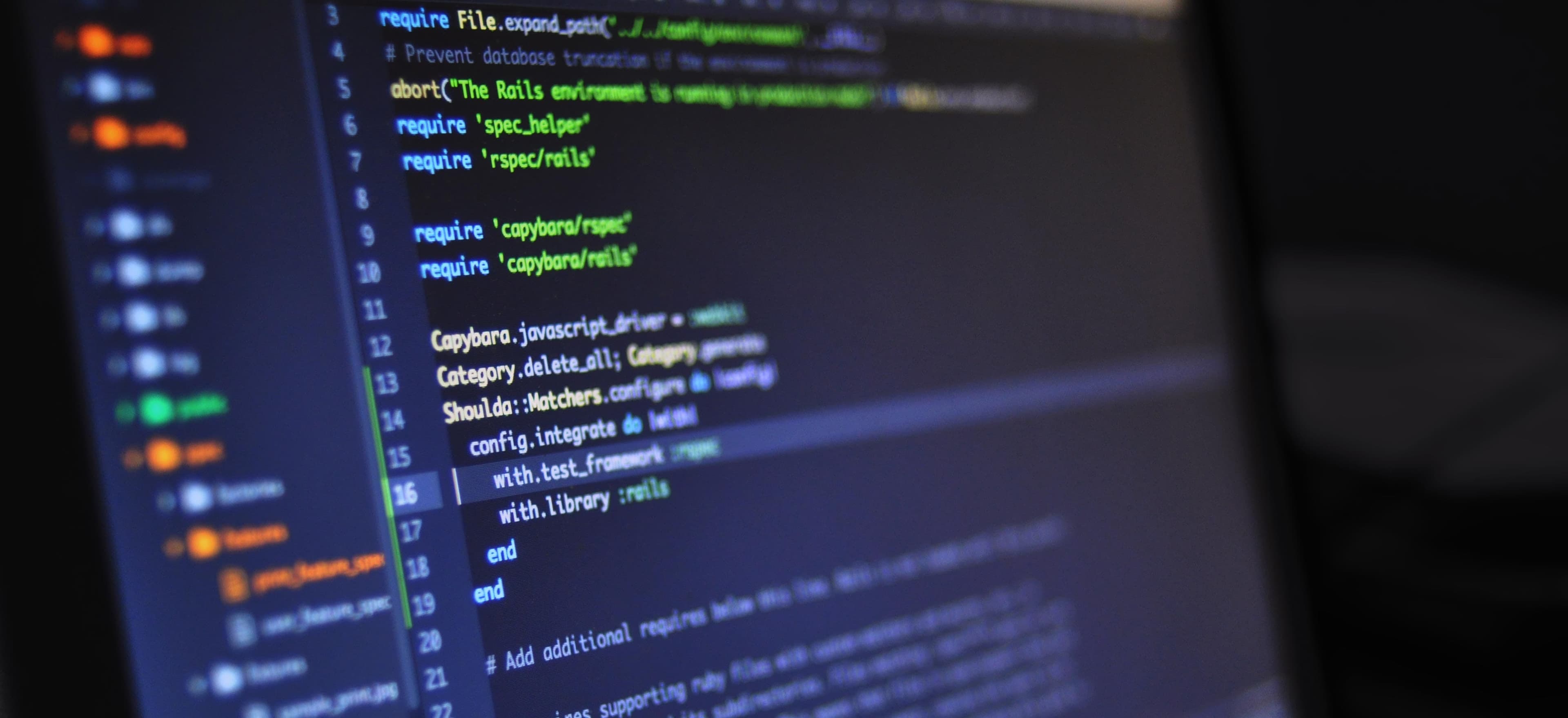
- Published on
Streamline Your Linux Dev Setup: Avoid Common Pitfalls
As a Java developer, setting up a productive and efficient development environment is crucial. It can significantly impact your coding speed, debugging capabilities, and overall satisfaction with the development process. In this post, we will discuss how to streamline your Linux development setup to avoid common pitfalls and maximize your productivity.
Choose the Right Integrated Development Environment (IDE)
One of the first decisions you'll make as a Java developer is choosing the right Integrated Development Environment (IDE). While there are several good options available, IntelliJ IDEA stands out as a powerful and feature-rich IDE for Java development. Its robust code analysis, refactoring tools, and seamless integration with build tools like Maven and Gradle make it an ideal choice for Linux-based Java development.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
The above code snippet demonstrates a simple "Hello, World" program in Java. Utilizing a robust IDE like IntelliJ IDEA can provide features such as auto-completion, code navigation, and intelligent refactoring, all of which contribute to a more streamlined development experience.
Embrace Build Automation Tools
In the realm of Java development, build automation tools play a crucial role in streamlining the development process. Tools like Apache Maven and Gradle automate the process of building, testing, and packaging Java applications, thereby reducing manual errors and enhancing reproducibility.
By utilizing a build automation tool, developers can define project structures, manage dependencies, and execute tasks effortlessly. The following snippet exemplifies a simple pom.xml
configuration file for a Maven project:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
</properties>
</project>
By integrating tools like Maven or Gradle into your Linux development environment, you can standardize the build process, manage dependencies effectively, and improve the overall project structure and organization.
Leverage Version Control Systems
Version control is a fundamental aspect of modern software development. Git, being one of the most widely used version control systems, is an essential tool for Java developers. Its distributed nature, branching and merging capabilities, and strong community support make it an ideal choice for managing source code.
By effectively utilizing Git within your Linux development environment, you can track changes, collaborate with team members, and ensure the integrity and consistency of your codebase.
Optimize Java Virtual Machine (JVM) Performance
The Java Virtual Machine (JVM) plays a vital role in the execution of Java applications. Optimizing the JVM for better performance is crucial for a streamlined development experience. Tools like VisualVM and JConsole provide insights into JVM performance, memory usage, and garbage collection metrics, enabling developers to identify and resolve performance bottlenecks.
By carefully tuning JVM parameters and monitoring application behavior, developers can ensure optimal performance and efficient memory utilization.
Ensure Effective Dependency Management
Effective dependency management is critical for Java projects. Tools like Apache Maven and Gradle, as mentioned earlier, facilitate dependency management by providing a structured approach to declaring, resolving, and managing dependencies.
By utilizing these tools, developers can ensure that their projects have well-defined dependencies, which are automatically resolved from repositories, thereby reducing the risk of dependency conflicts and ensuring a smooth and efficient build process.
Stay Updated with Java Language Features and Libraries
Keeping up with the latest Java language features and libraries is essential for enhancing productivity and staying competitive in the ever-evolving landscape of Java development.
Java's official website and resources like Baeldung and Vogella are valuable sources for staying updated with the latest Java language features, best practices, and emerging libraries. Incorporating new language features and leveraging modern libraries can significantly improve code quality, maintainability, and developer efficiency.
Wrapping Up
Optimizing your Linux development environment for Java involves making informed choices about the tools, frameworks, and processes that best suit your project requirements. By embracing powerful IDEs, efficient build automation, robust version control, and proactive performance optimization, you can streamline your development workflow and avoid common pitfalls.
With the right tools and practices in place, you can maximize your productivity as a Java developer and tackle complex challenges with confidence, all within a streamlined Linux development environment.