Simplifying Complex Systems: The Power of Facade Pattern
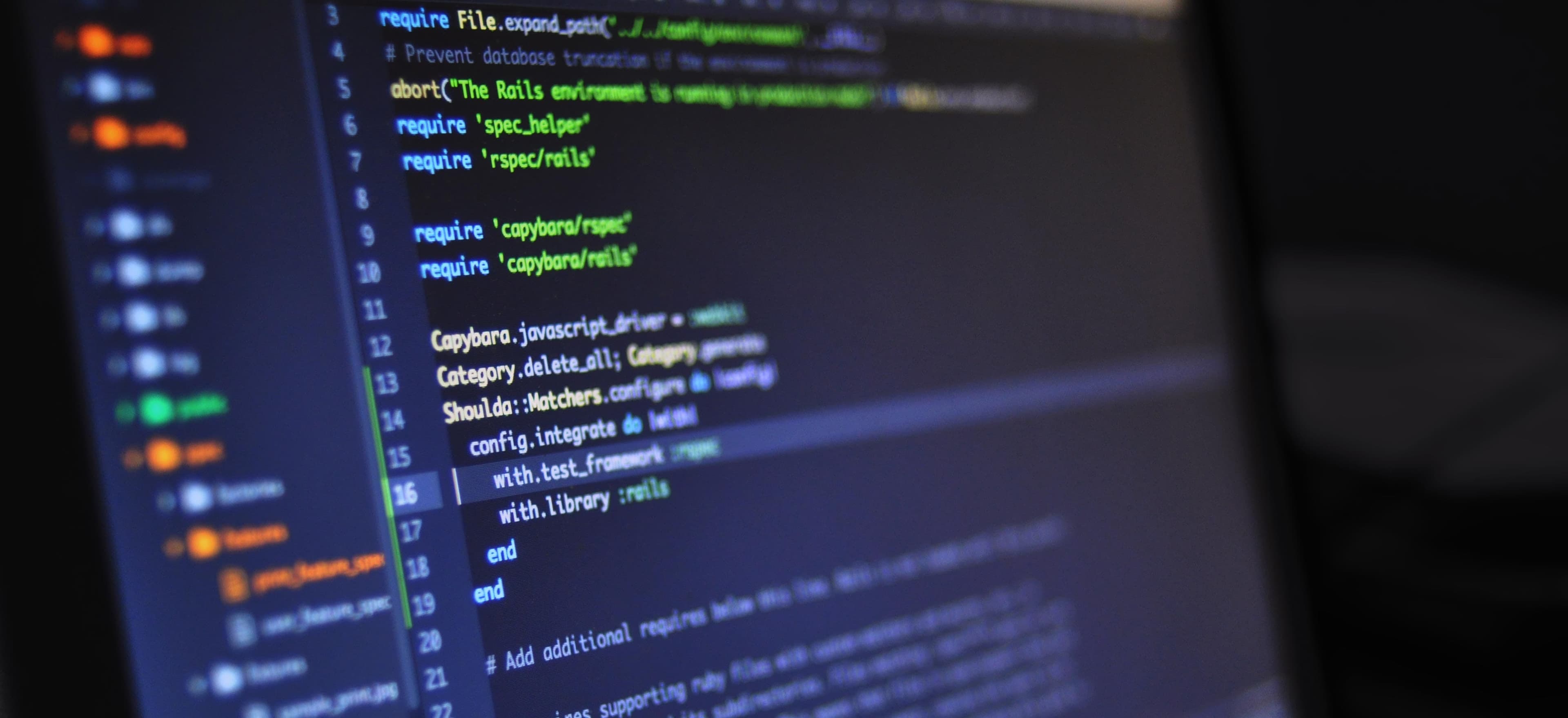
- Published on
Simplifying Complex Systems: The Power of Facade Pattern
In the ever-evolving world of software development, complexity is a double-edged sword. On one hand, complex systems offer powerful, flexible solutions to a wide array of problems. On the other, they can become a tangled web for developers and end-users alike. This is where design patterns come into play, serving as a beacon for navigating through the complexity. Among these patterns, the Facade Pattern stands out for its simplicity and efficacy. Today, we're diving into the intricacies of the Facade Pattern, unveiling its power to simplify complex systems, and providing Java code snippets to help you implement it in your projects.
Understanding the Facade Pattern
At its core, the Facade Pattern is structurally simple yet profoundly impactful. It provides a unified, higher-level interface to a set of interfaces in a subsystem, making the subsystem easier to use. Think of it as an abstraction layer that wraps a complicated subsystem with a simpler interface. By doing so, it decreases the system's overall complexity and increases its usability and maintainability.
Why Facade Pattern?
- Simplicity: It simplifies the interface to complex systems.
- Separation of Concerns: It decouples the system from clients and other subsystems, promoting loose coupling.
- Ease of Use: It makes the system easier for beginners and external systems to use.
Implementing Facade Pattern in Java
To illustrate the practicality and usage of the Facade Pattern, let's consider an example of a home entertainment system. This system includes a television, a sound system, and a gaming console. Each component has its own complex interface, but we want to simplify turning the entire system on and off with a single button press.
Step 1: Define Subsystem Interfaces
First, we define the complex interfaces of our subsystems.
// Television.java
public class Television {
public void turnOn() {
System.out.println("Television is turning on...");
}
public void turnOff() {
System.out.println("Television is turning off...");
}
}
// SoundSystem.java
public class SoundSystem {
public void turnOn() {
System.out.println("Sound system is turning on...");
}
public void turnOff() {
System.out.println("Sound system is turning off...");
}
}
// GamingConsole.java
public class GamingConsole {
public void turnOn() {
System.out.println("Gaming console is turning on...");
}
public void turnOff() {
System.out.println("Gaming console is turning off...");
}
}
Step 2: Implement the Facade
Now, we encapsulate these subsystems within a Facade class, providing a simplified interface for turning the entire system on and off.
// HomeEntertainmentFacade.java
public class HomeEntertainmentFacade {
private Television tv;
private SoundSystem soundSystem;
private GamingConsole gamingConsole;
public HomeEntertainmentFacade(Television tv, SoundSystem soundSystem, GamingConsole gamingConsole) {
this.tv = tv;
this.soundSystem = soundSystem;
this.gamingConsole = gamingConsole;
}
public void turnOnEverything() {
tv.turnOn();
soundSystem.turnOn();
gamingConsole.turnOn();
System.out.println("Everything is turned on. Enjoy!");
}
public void turnOffEverything() {
tv.turnOff();
soundSystem.turnOff();
gamingConsole.turnOff();
System.out.println("Everything is turned off. Goodbye!");
}
}
Step 3: Utilize the Facade
Finally, clients can interact with the complex subsystems through the simplified interface provided by the Facade.
public class Main {
public static void main(String[] args) {
Television tv = new Television();
SoundSystem soundSystem = new SoundSystem();
GamingConsole gamingConsole = new GamingConsole();
HomeEntertainmentFacade homeEntertainment = new HomeEntertainmentFacade(tv, soundSystem, gamingConsole);
homeEntertainment.turnOnEverything(); // Turns on all components
// Do something enjoyable...
homeEntertainment.turnOffEverything(); // Turns off all components
}
}
Why This Matters
By abstracting the complexity of the subsystems into a unified interface, the Facade Pattern significantly simplifies client interactions. Clients need not concern themselves with the inner workings of the subsystems, leading to cleaner, more readable, and maintainable code.
Moreover, the Facade Pattern is not limited to software architecture. Its principles are widely applicable in various fields, including web development, where frameworks and libraries act as facades over complex underlying technologies. For more insights into design patterns and their application in software development, consider visiting Refactoring.Guru and the Gang of Four’s seminal work.
Key Takeaways
The Facade Pattern is a potent tool in a developer's arsenal for battling complexity. It excels in the following areas:
- Providing a simple interface to complex systems.
- Enhancing system legibility and maintainability.
- Promoting loose coupling between subsystems and their clients.
Remember, the ultimate goal of using the Facade Pattern, or any design pattern for that matter, is to write code that’s not just functional but also clean, understandable, and easy to manage. Whether you’re a seasoned developer or new to the game, mastering the Facade Pattern is a step towards achieving that goal.
Happy coding, and may your systems always be effortlessly manageable!
By demystifying the Facade Pattern and demonstrating its implementation in Java, this blog post aims to empower developers to harness its simplicity and power. Through strategic abstraction, the Facade Pattern enables efficient interaction with complex systems, ensuring code remains both robust and accessible.
Checkout our other articles