Trim the Fat: Streamlining Code with Allocation Analysis
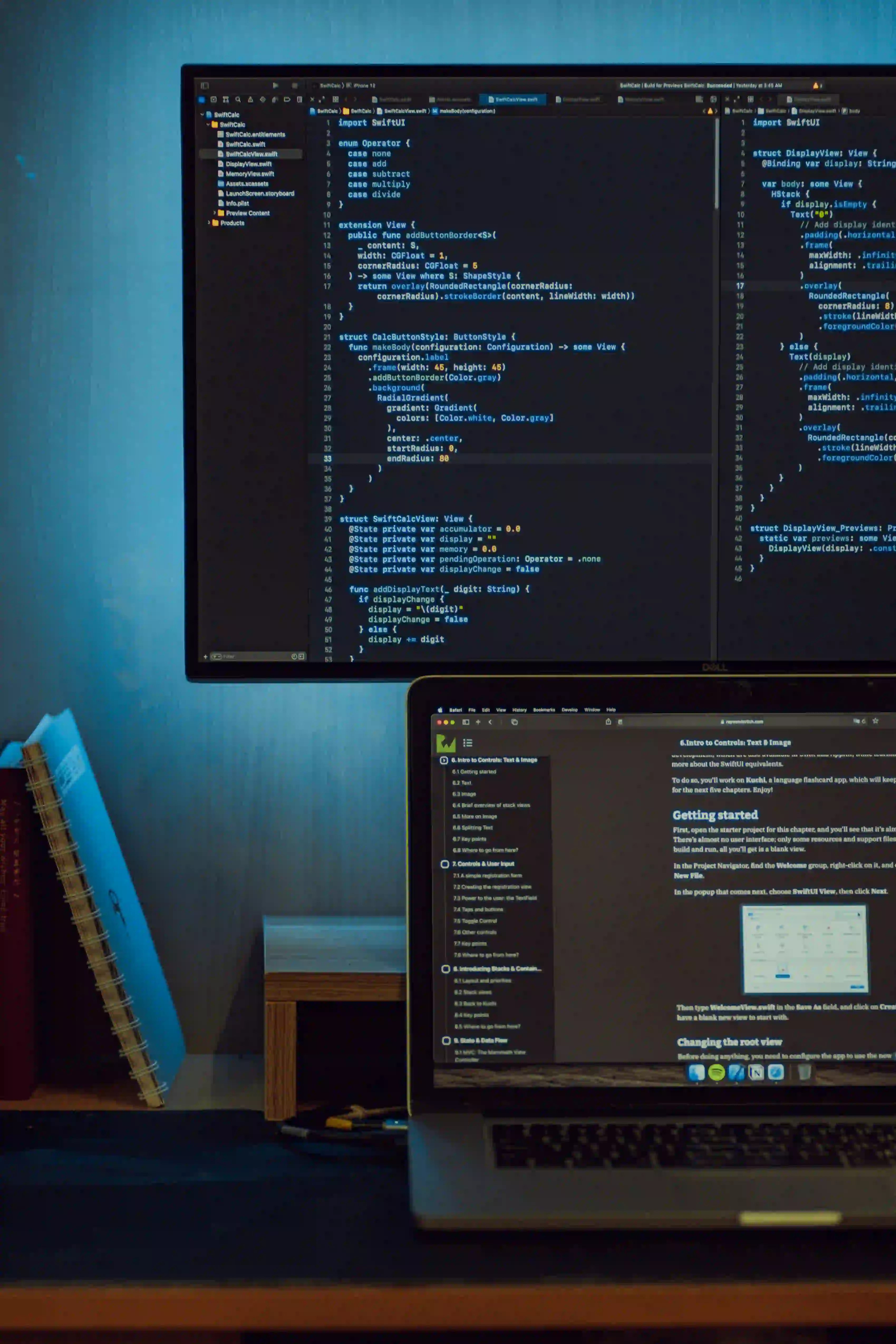
Trim the Fat: Streamlining Code with Allocation Analysis
In the world of Java programming, the quest for optimized and efficient code is an ongoing endeavor. One of the crucial aspects of this is reducing memory allocations and garbage collection overhead. This can significantly impact the performance of your applications, especially in resource-constrained environments. In this blog post, we will delve into the concept of allocation analysis in Java and explore how it can help streamline code for better memory management and improved performance.
Understanding Allocation Analysis
Allocation analysis involves the examination of memory allocations made by an application during its execution. It helps in identifying unnecessary object creations, excessive memory consumption, and potential memory leaks. By analyzing the allocation patterns, developers can gain insights into how memory is being utilized and pinpoint areas where optimizations can be made.
In Java, allocation analysis can be performed using various tools and techniques. One commonly used approach is to leverage profilers such as VisualVM or Java Mission Control to capture and analyze memory allocation data. These tools provide valuable information about object allocations, garbage collection events, and memory usage, enabling developers to identify allocation hotspots and areas for improvement.
The Impact of Excessive Allocations
Excessive memory allocations can have a detrimental impact on the performance of Java applications. When objects are frequently created and discarded, it puts a strain on the garbage collector, leading to increased overhead and potential performance bottlenecks. Moreover, excessive allocations can contribute to heap fragmentation, which can further degrade the overall memory management efficiency.
Consider the following code snippet:
List<String> names = new ArrayList<>();
for (int i = 0; i < 1000; i++) {
names.add("Name" + i);
}
In this example, a new String
object is created in each iteration of the loop, leading to a significant number of allocations. This can result in unnecessary memory overhead and increased garbage collection pressure.
Strategies for Allocation Optimization
To address excessive allocations and improve memory management, developers can employ several strategies and best practices. Let's explore some effective approaches to streamline code through allocation optimization.
Reusing Objects
One way to reduce allocations is by reusing objects wherever possible. This can be achieved by utilizing object pools or implementing object-reuse patterns. By reusing objects instead of creating new ones, unnecessary allocations can be minimized, thereby alleviating the burden on the garbage collector.
Consider the following example:
// Object pool for reusing StringBuilder instances
Deque<StringBuilder> stringBuilderPool = new ArrayDeque<>();
public StringBuilder obtainStringBuilder() {
if (stringBuilderPool.isEmpty()) {
return new StringBuilder();
} else {
return stringBuilderPool.poll();
}
}
public void releaseStringBuilder(StringBuilder sb) {
sb.setLength(0); // Clear the contents
stringBuilderPool.offer(sb);
}
In this example, a pool of StringBuilder
instances is maintained to facilitate object reuse. When a StringBuilder
is needed, it is obtained from the pool, and after its use, it is released back to the pool for future reuse. This can help minimize unnecessary allocations of StringBuilder
objects.
Using Immutable Objects
Immutable objects can also play a pivotal role in allocation optimization. By leveraging immutable objects, developers can eliminate the need for frequent object creations, as the state of an immutable object cannot be modified once it is created. This not only reduces memory allocations but also contributes to a more predictable and thread-safe programming model.
Consider the following immutable class:
public final class Point {
private final int x;
private final int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
}
In this example, the Point
class is immutable, making it suitable for scenarios where frequent object creation is not desirable. Immutable objects exhibit value stability, allowing for efficient memory usage and reduced allocation overhead.
Opting for Primitive Types
When working with numerical data, opting for primitive types instead of their object counterparts can lead to significant allocation savings. Primitive types such as int
, long
, double
, etc., do not require heap allocation and incur lower memory overhead compared to their respective object wrappers.
Consider the following code snippet:
// Using primitive type (int) instead of Integer object
int sum = 0;
for (int i = 1; i <= 1000; i++) {
sum += i;
}
In this example, the use of the int
primitive type for sum
avoids the overhead of creating Integer
objects, resulting in more efficient memory utilization.
Leveraging Allocation Analysis for Optimization
Allocation analysis serves as a valuable tool for identifying optimization opportunities within Java code. By analyzing memory allocation patterns and profiling object creations, developers can gain insights into where allocations are occurring and take targeted measures to optimize memory usage.
When conducting allocation analysis, developers can look for repetitive object creations, unnecessary temporary objects, and potential areas for object reuse. Through a careful examination of allocation hotspots, developers can apply targeted optimizations to streamline code and enhance memory management.
By integrating allocation analysis into the development workflow, developers can proactively identify and address allocation-related performance concerns, leading to more efficient and performant Java applications.
Final Considerations
In the pursuit of efficient and optimized Java code, allocation analysis plays a crucial role in identifying and mitigating excessive memory allocations. By leveraging tools, techniques, and best practices for allocation optimization, developers can streamline code, minimize unnecessary memory overhead, and improve the overall performance of Java applications.
As a Java developer, incorporating allocation analysis into your development process can lead to tangible benefits in terms of memory management, garbage collection efficiency, and overall application performance. By trimming the fat and optimizing memory allocations, you can ensure that your Java code runs lean and performs at its best.
In the ever-evolving landscape of Java development, allocation analysis stands as a fundamental practice for crafting high-performance, resource-efficient applications.
So, trim the fat, streamline your code, and harness the power of allocation analysis for optimized Java programming.
Happy coding!