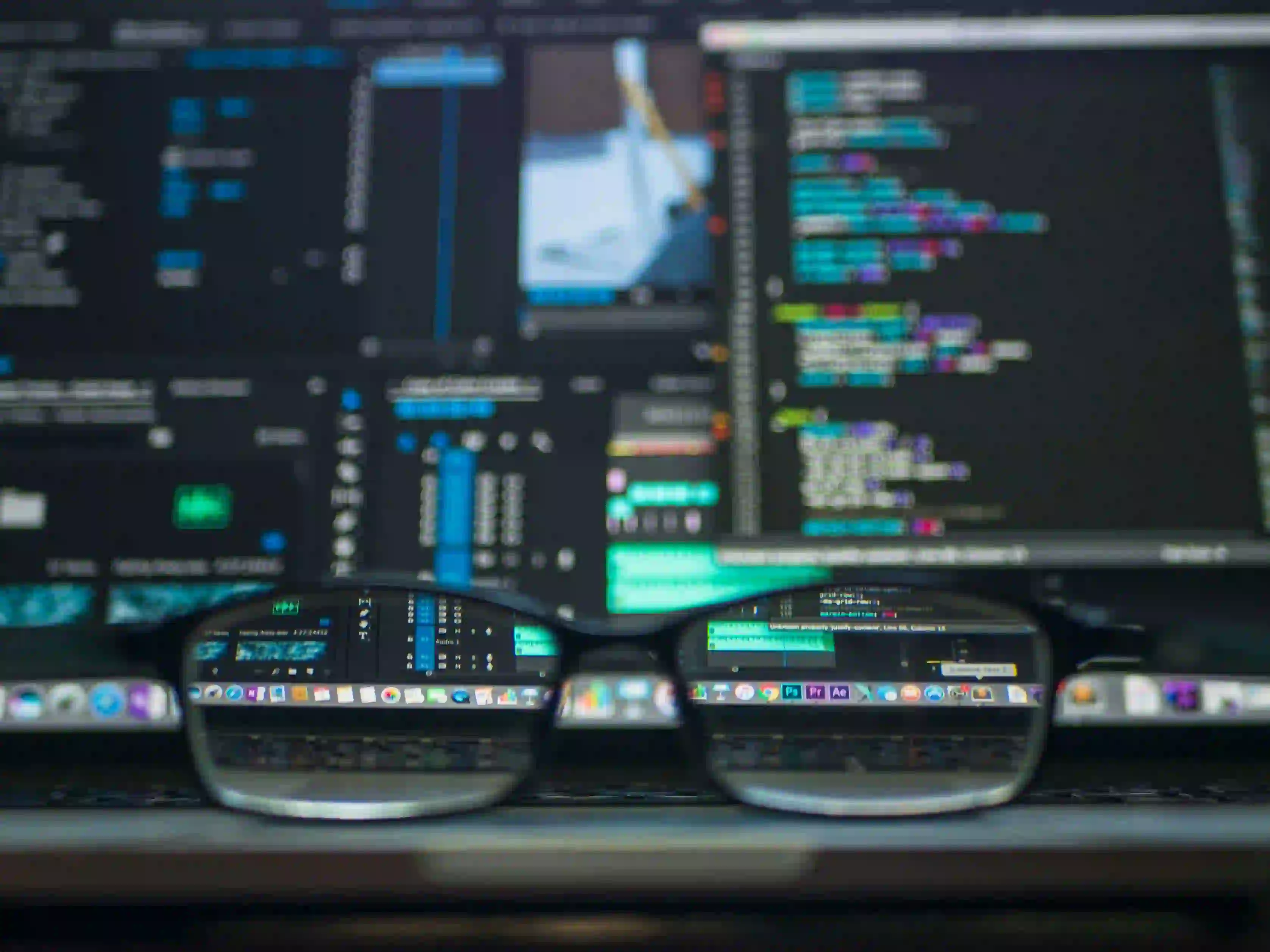
Overcoming Hurdles: Setting Up Zuul Gateway in Spring Boot
In the world of microservices, managing and routing traffic between different services can be quite challenging. Thankfully, with the help of Zuul, a powerful API gateway, this task becomes much more manageable. In this blog post, we'll dive into setting up Zuul Gateway in a Spring Boot application, and explore how it can help us overcome the hurdles of microservices architecture.
What is Zuul Gateway?
Zuul is a dynamic routing and filtering gateway that sits at the edge of your microservices ecosystem. It allows you to route requests to your services, apply cross-cutting concerns such as authentication and monitoring, and provides a unified interface for your clients.
Setting Up Zuul Gateway
Step 1: Create a Spring Boot Project
First, we need to create a new Spring Boot project. You can use Spring Initializr to quickly generate a new project with the necessary dependencies.
Step 2: Add Zuul Dependency
Once your project is set up, add the Zuul dependency to your pom.xml
or build.gradle
file.
For Maven:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-zuul</artifactId>
</dependency>
For Gradle:
implementation 'org.springframework.cloud:spring-cloud-starter-netflix-zuul'
Step 3: Enable Zuul Proxy
Next, you need to enable Zuul proxy by annotating your main application class with @EnableZuulProxy
.
@SpringBootApplication
@EnableZuulProxy
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
}
By adding @EnableZuulProxy
, you're telling Spring Boot to enable Zuul capabilities in your application.
Step 4: Configure Routes
Now, you can configure the routes for your services. You can do this in the application.properties
file.
zuul.routes.users.path=/users/**
zuul.routes.users.serviceId=user-service
In this example, any request to /users
will be routed to the user-service
.
Step 5: Run and Test
Finally, you can run your Zuul Gateway application and test the routing by sending requests to the defined routes.
Why Use Zuul Gateway?
Dynamic Routing
Zuul provides dynamic routing of requests, allowing you to easily add and remove services without impacting clients. This makes it incredibly flexible and scalable in a microservices environment.
Cross-Cutting Concerns
With Zuul, you can apply cross-cutting concerns such as authentication, rate limiting, and monitoring at the gateway level. This simplifies the implementation of these concerns across multiple services.
Simplified Client Interface
By providing a unified interface for clients, Zuul hides the complexities of your microservices architecture, making it easier for clients to consume your services.
Closing Remarks
Setting up Zuul Gateway in a Spring Boot application can greatly simplify the management of microservices architecture. Its dynamic routing, cross-cutting concerns, and simplified client interface make it a powerful tool in the world of microservices. By following the steps outlined in this post, you can easily overcome the hurdles of microservices architecture and set up a robust gateway for your services.
To dive deeper into Zuul Gateway and Spring Boot, check out the official documentation and start exploring the endless possibilities it offers. Happy coding!