Choosing the Right HTTP Method for Your API: POST vs PUT vs PATCH
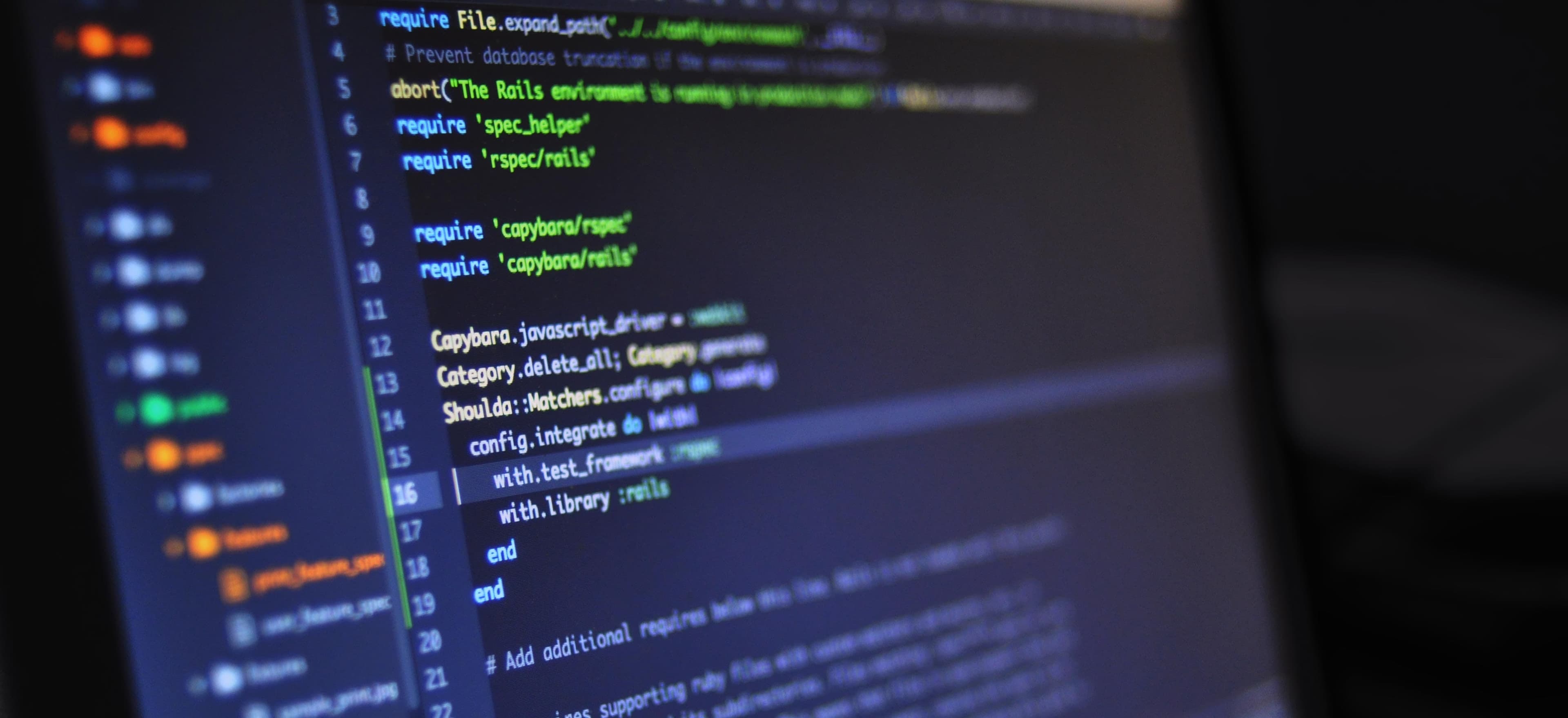
- Published on
Choosing the Right HTTP Method for Your API: POST vs PUT vs PATCH
When building an API, one of the essential decisions you'll face is choosing the appropriate HTTP method for your endpoints. In RESTful API design, we commonly use POST, PUT, and PATCH to create, update, and modify resources. Each of these methods has its own purpose, and understanding when to use each can greatly improve the design and functionality of your API.
Understanding the Basics of Each Method
POST
The POST method is used to submit an entity to the specified resource, often causing a change in state or side effects on the server. It is commonly used for creating new resources or submitting data to the server for processing.
PUT
The PUT method, on the other hand, is used to replace the entire entity at the specified resource or create it if it doesn't exist. When using PUT, the client specifies the entire resource, and any missing fields can be interpreted as null or default values.
PATCH
PATCH is used to apply partial modifications to a resource, providing a way to update specific fields without having to send the entire entity. This method is particularly useful when you only need to modify a few fields in a resource without affecting the rest of the data.
Understanding When to Use POST
POST is commonly used for creating new resources. For example, when you're adding a new item to a collection, such as creating a new user account or submitting a new blog post, POST is the appropriate choice. It's also suitable for operations that trigger side effects, like sending an email notification or processing payment.
In Java, you can use the POST method in your API using a framework like Spring Boot. Here's an example of a POST endpoint using Spring's @RestController
:
@RestController
public class UserController {
@PostMapping("/users")
public ResponseEntity<User> createUser(@RequestBody User user) {
// Logic to create a new user
return ResponseEntity.status(HttpStatus.CREATED).body(createdUser);
}
}
In this example, the @PostMapping
annotation is used to map the createUser
method to the /users
endpoint, allowing clients to create a new user by submitting the user data in the request body.
Understanding When to Use PUT
PUT is best suited for updating existing resources or creating a new resource if it doesn't exist. When you have the entire representation of the resource available and want to store it at the specified URI, PUT is the right choice. It's important to note that using PUT to update a resource requires the client to send the entire updated entity, as any fields not provided will be overwritten with null or default values.
Let's consider an example of using PUT in a Java API. Suppose we have a Product
resource, and we want to update it with new information. Here's how we can implement a PUT endpoint using Spring:
@RestController
public class ProductController {
@PutMapping("/products/{id}")
public ResponseEntity<Product> updateProduct(@PathVariable Long id, @RequestBody Product updatedProduct) {
// Logic to update the product with the provided id
return ResponseEntity.ok(updatedProduct);
}
}
In this case, the @PutMapping
annotation maps the updateProduct
method to the /products/{id}
endpoint, allowing clients to update a product by providing the updated product representation in the request body.
Understanding When to Use PATCH
PATCH is ideal for making partial updates to existing resources. When you only need to modify specific fields of a resource without sending the entire entity, PATCH is the most suitable method to use. This can be especially helpful when dealing with large resources where sending the entire entity for a small change is unnecessary.
In a Java API, you can implement a PATCH endpoint using a library like Spring Boot. Let's consider an example using the User
resource again:
@RestController
public class UserController {
@PatchMapping("/users/{id}")
public ResponseEntity<User> updateUser(@PathVariable Long id, @RequestBody Map<String, Object> updates) {
// Logic to apply partial updates to the user with the provided id
return ResponseEntity.ok(updatedUser);
}
}
In this example, the @PatchMapping
annotation maps the updateUser
method to the /users/{id}
endpoint, allowing clients to send a JSON object with the fields to be updated in the request body, and the server applies those changes to the user with the specified id.
Making the Right Choice for Your API
In conclusion, choosing the right HTTP method for your API endpoints is crucial for maintaining a well-designed and functional API. Understanding the differences between POST, PUT, and PATCH allows you to make informed decisions based on the specific requirements of your resources and operations.
When designing your API in Java, it's essential to leverage the capabilities of frameworks like Spring Boot to easily implement and handle these HTTP methods. By using annotations such as @PostMapping
, @PutMapping
, and @PatchMapping
, you can clearly define the intent and functionality of your API endpoints, providing a clear and intuitive interface for clients to interact with your API.
Ultimately, your choice of HTTP method should align with the principles of RESTful API design and the specific actions your endpoints are expected to perform. With a solid understanding of when to use POST, PUT, and PATCH, you can confidently build APIs that are robust, efficient, and maintainable.
Incorporating the appropriate HTTP methods in your API design is fundamental to achieving a well-organized and purposeful system. Whether you're creating, updating, or modifying resources, selecting the suitable method ensures that your API operates seamlessly, distinctly serving the unique needs of your project.
When developing a Java API, making informed choices regarding HTTP methods allows you to enhance the functionality of your endpoints, contributing to a cohesive and effective system. By leveraging the features of frameworks such as Spring Boot, you can efficiently implement and manage these methods, paving the way for an intuitive and streamlined interaction with your API.
To further delve into the depths of RESTful API design and HTTP methods, consider exploring in-depth resources such as RESTful API Design: Best Practices, where you can gain comprehensive insights and best practices for creating robust and efficient APIs.
Finally, by honing your understanding of when to use POST, PUT, and PATCH, you can construct APIs that are resilient, performant, and tailored to meet the specific requirements of your applications. Embracing the principles of RESTful design and selecting the appropriate HTTP methods ensures that your Java API thrives as a flexible, scalable, and well-structured system.