5 Tricky Pitfalls When Upgrading to Java 7 Explained
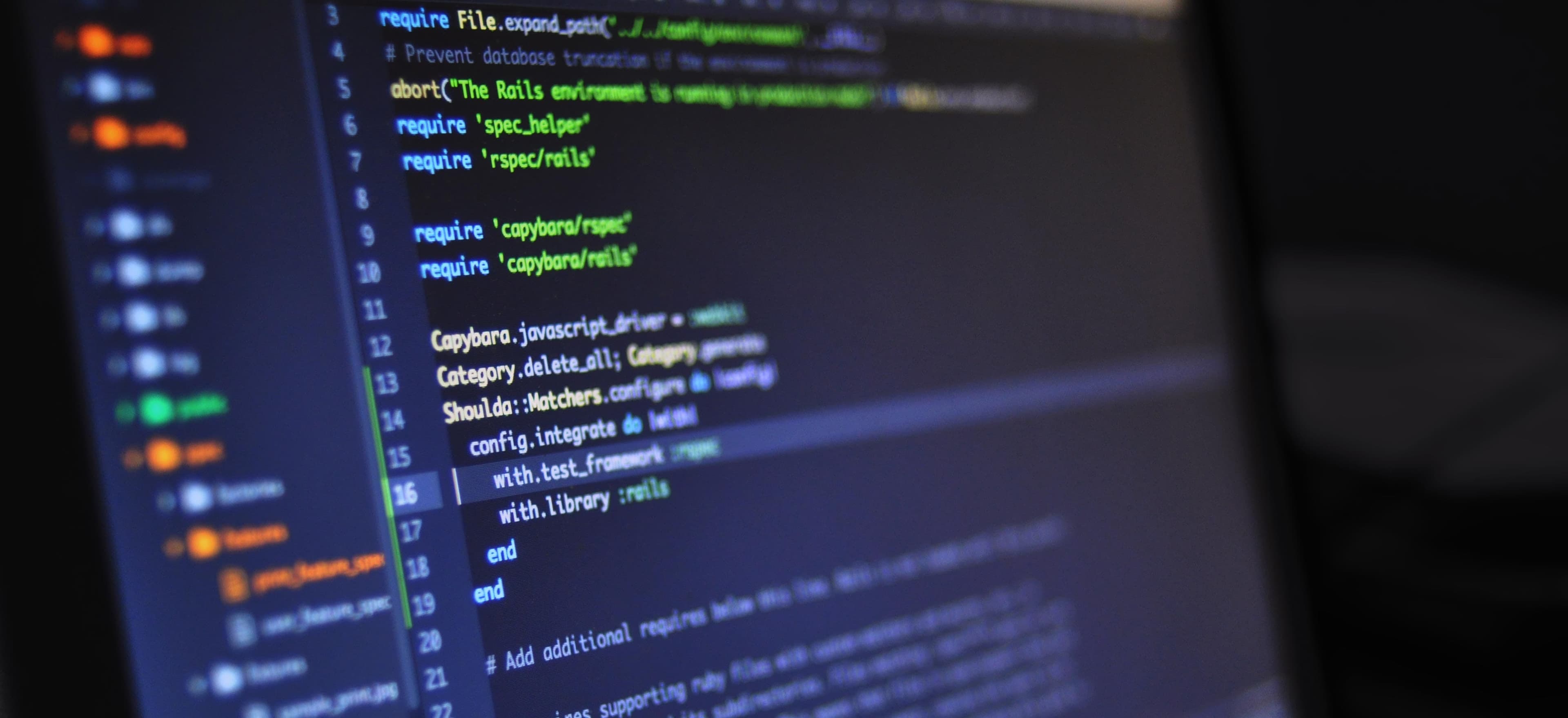
- Published on
5 Tricky Pitfalls When Upgrading to Java 7 Explained
Upgrading to a new version of Java can be an exciting but challenging endeavor. Java 7, released in 2011, introduced several new features and improvements over its predecessor. However, this upgrade can be fraught with pitfalls for the unwary. In this post, we'll delve into five tricky pitfalls that you may encounter when upgrading to Java 7, and how to overcome them.
Pitfall 1: Binary Compatibility
When transitioning to Java 7, it's crucial to ensure binary compatibility with existing libraries and dependencies. Java 7 brought language enhancements and API changes that could potentially break compatibility with older code. One of the main culprits is the introduction of the diamond operator (<>
) for type inference in generics.
For instance, consider the following Java 6 code:
List<String> list = new ArrayList<String>();
In Java 7 and later, this can be simplified as:
List<String> list = new ArrayList<>();
The diamond operator improves code readability, but if your existing codebase uses libraries that rely on the explicit type declaration, this change can lead to incompatibility issues.
To address this, it's essential to thoroughly test all existing code with the new Java version, ensuring that no compatibility issues arise from these language enhancements.
Pitfall 2: Unintended Consequences of Automatic Resource Management
Java 7 introduced the try-with-resources statement, a feature aimed at simplifying resource management by automatically closing resources at the end of the block. While this is a powerful addition, it comes with its own set of pitfalls.
Consider the following code snippet:
try (FileInputStream fis = new FileInputStream("input.txt");
FileOutputStream fos = new FileOutputStream("output.txt")) {
// Process the files
} catch (IOException e) {
// Handle the exception
}
The try-with-resources statement will automatically close both FileInputStream
and FileOutputStream
at the end of the block. However, this may lead to unexpected behavior if the close()
method of a resource throws an exception. In such cases, any exception thrown during the resource cleanup could potentially mask the original exception thrown within the block.
To mitigate this, it's crucial to handle exceptions thrown during resource cleanup appropriately. In Java 7, the Throwable
class introduced the addSuppressed()
method, which can be used to attach suppressed exceptions to the primary exception.
Pitfall 3: Changes to the Fork/Join Framework
Java 7 introduced the Fork/Join framework, a powerful addition for parallel programming. However, migrating code that uses traditional thread-based concurrency to the Fork/Join framework can be challenging.
One of the key differences is that tasks in the Fork/Join framework are not executed in separate threads from the common pool. Instead, they are split into subtasks, which are then executed in a recursive manner. This fundamental difference can lead to unexpected behavior and performance issues if not handled carefully during the migration process.
It's important to thoroughly review and refactor the existing concurrency code to leverage the Fork/Join framework effectively, taking into account its unique execution model and limitations.
Pitfall 4: Behavioral Changes in Libraries and APIs
Java 7 introduced several behavioral changes in libraries and APIs that could impact existing code. For example, changes to the ConcurrentHashMap
class resulted in modifications to the way it handles concurrent updates, potentially affecting the behavior of applications relying on its previous behavior.
Additionally, improvements to the java.nio.file
package brought changes to file I/O operations and error handling. Code that relies on these APIs must be carefully reviewed and adapted to accommodate the behavioral changes introduced in Java 7.
Furthermore, the introduction of the java.util.Objects
class brought in new utility methods, such as requireNonNull()
, which can have subtle yet substantial impacts on existing code that leverages null checks and exception handling.
To address these pitfalls, thorough testing and refactoring are necessary to ensure that the behavioral changes in libraries and APIs do not adversely affect the functionality of the existing codebase.
Pitfall 5: Legacy Code Adaptation
Lastly, a common pitfall when upgrading to Java 7 is the adaptation of legacy code that relies on deprecated features. Java 7 deprecated several classes, methods, and features, necessitating adjustments to the existing codebase.
For example, the Thread.stop()
and Runtime.runFinalizersOnExit()
methods were deprecated, requiring alternative approaches to achieve the same functionality. Legacy code that depends on these deprecated features must be carefully refactored to align with the new standards and best practices introduced in Java 7.
Additionally, the removal of the permanent generation in the HotSpot JVM introduced changes to memory management and garbage collection. Code relying on specific behavior of the permanent generation must be updated to accommodate these changes.
Thorough code analysis, refactoring, and adherence to the Java 7 migration guide are essential to successfully adapt legacy code to the new standards and best practices.
In conclusion, while the upgrade to Java 7 brings forth numerous benefits and new features, it's not without its share of pitfalls. By understanding, anticipating, and addressing these tricky hurdles, developers can ensure a smooth transition to Java 7 while reaping the rewards of enhanced performance, improved language features, and expanded APIs.
Remember, awareness and preparation are the keys to overcoming the potential pitfalls of upgrading to Java 7. With careful planning, thorough testing, and vigilant adaptation of existing code, the transition can be navigated successfully, paving the way for leveraging the full potential of Java 7.
For more in-depth guidance on upgrading to Java 7, refer to the official Java SE 7 documentation and the comprehensive migration resources provided by the Java community.
Checkout our other articles