Mastering Java 8 Streams: The Art of Predicate Negation
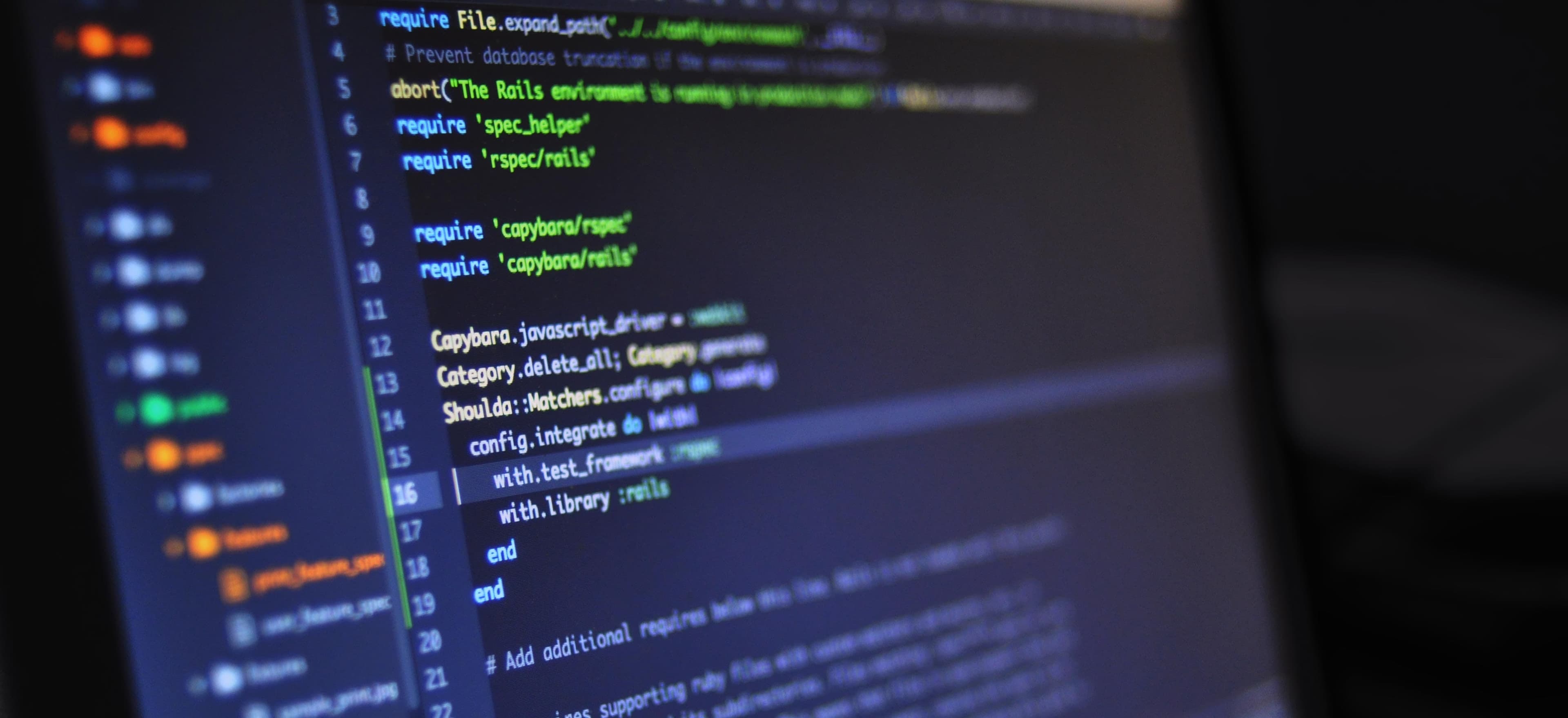
- Published on
Mastering Java 8 Streams: The Art of Predicate Negation
In Java 8, the introduction of streams revolutionized the way we work with collections. Leveraging lambda expressions and functional programming techniques, streams offer a concise and powerful way to process data. One important feature of streams is the ability to filter elements based on a predicate. However, there are scenarios where we need to negate a predicate to achieve our desired result. In this article, we will explore the art of predicate negation in Java 8 streams, delving into various techniques and best practices.
Understanding Predicates and Predicate Negation
In Java, a Predicate
is a functional interface that represents a boolean-valued function of one argument. It is commonly used with streams to filter elements based on certain criteria. For instance, suppose we have a collection of numbers and we want to filter out the even numbers. We can use a predicate to define this filtering condition and pass it to the filter
operation of a stream.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers); // Output: [2, 4, 6, 8, 10]
The predicate n -> n % 2 == 0
is used to filter out the even numbers from the stream. But what if we want to filter out the odd numbers instead? This is where predicate negation comes into play. By negating the original predicate, we can easily achieve our desired result.
Negating Predicates using negate()
In Java 8, the Predicate
interface provides the negate
method, which returns a predicate that represents the logical negation of the original predicate. Let's revisit our previous example and negate the predicate to filter out the odd numbers from the collection.
List<Integer> oddNumbers = numbers.stream()
.filter(((Predicate<Integer>) n -> n % 2 == 0).negate())
.collect(Collectors.toList());
System.out.println(oddNumbers); // Output: [1, 3, 5, 7, 9]
By using the negate
method, we inverted the filtering condition, effectively filtering out the odd numbers. This approach provides a clean and straightforward way to negate predicates in Java 8 streams.
Simplifying Predicate Negation with Predicate.not()
In Java 11, a new not
method was introduced in the Predicate
interface, providing a more intuitive and readable way to negate predicates. Let's refactor our previous example using the not
method.
List<Integer> oddNumbersJava11 = numbers.stream()
.filter(((Predicate<Integer>) n -> n % 2 == 0).not())
.collect(Collectors.toList());
System.out.println(oddNumbersJava11); // Output: [1, 3, 5, 7, 9]
The use of not
creates a more declarative and self-explanatory code, making the predicate negation process more intuitive and elegant.
Negating Predicates using De Morgan's Laws
In some cases, especially when dealing with complex predicates, applying De Morgan's Laws can simplify predicate negation. According to De Morgan's Laws, the negation of a conjunction (AND) is the disjunction (OR) of the negations, and vice versa. By decomposing the original predicate using these laws, we can effectively negate it.
Let's consider a scenario where we have a collection of strings and we want to filter out the elements that are neither starting with 'A' nor containing the letter 'i'. We can achieve this by decomposing the original predicate using De Morgan's Laws.
List<String> filteredStrings = someStrings.stream()
.filter(((Predicate<String>) s -> s.startsWith("A"))
.or(s -> !s.contains("i")))
.collect(Collectors.toList());
In this example, we first apply the negation to each individual condition and then combine them using the logical OR operator. This showcases the application of De Morgan's Laws to simplify predicate negation in Java 8 streams.
Lessons Learned
Predicate negation is a powerful concept when working with Java 8 streams. By understanding and utilizing the techniques discussed in this article, you can effectively negate predicates to filter elements based on your specific criteria. Whether using the negate
method, employing Predicate.not()
in Java 11, or applying De Morgan's Laws, mastering predicate negation will elevate your skills in stream processing and functional programming with Java.
Harness the power of predicate negation and take your Java 8 stream operations to the next level.
Remember, the art of predicate negation in Java is not only about achieving your desired result, but also about writing clear, maintainable, and readable code.
Happy Coding!