Maximizing Productivity with JShell: Common Pitfalls Explained
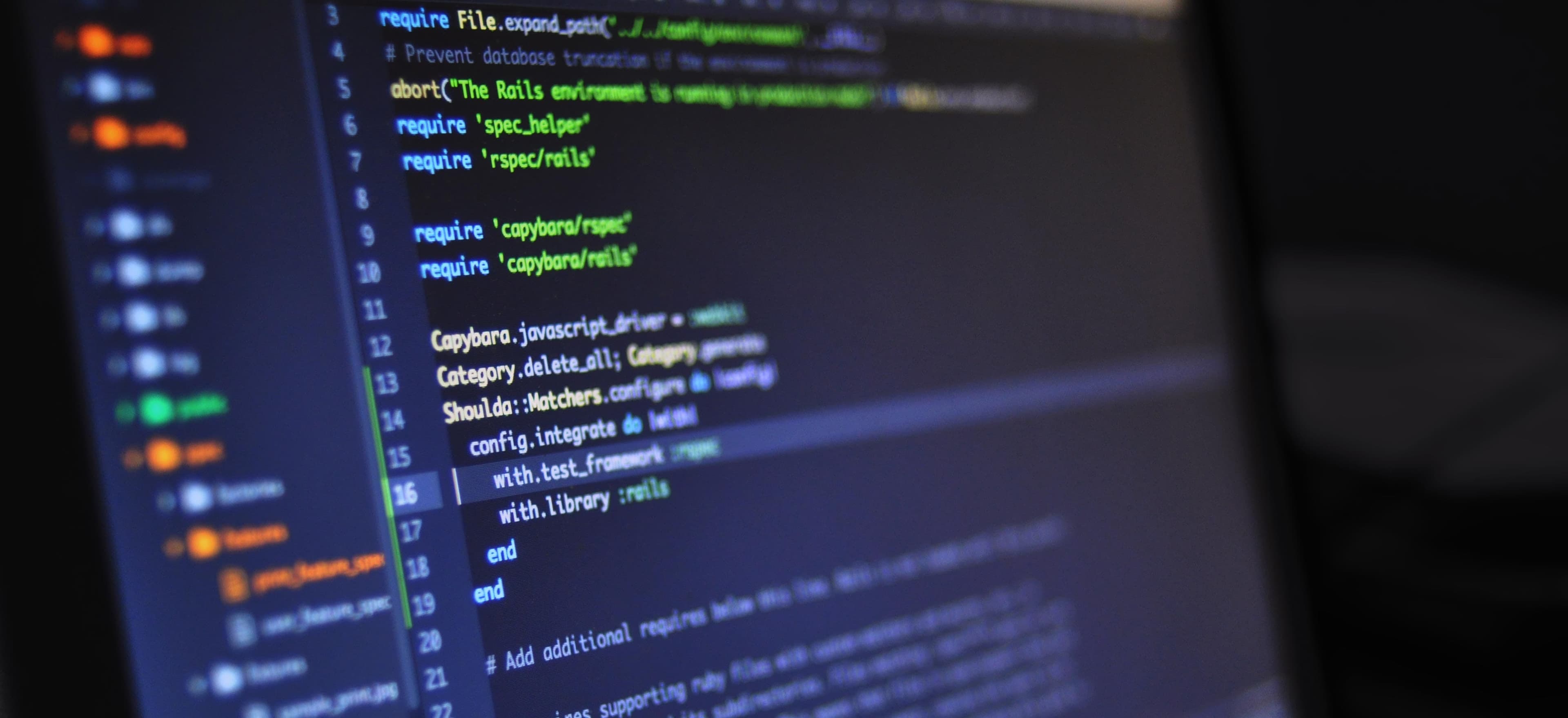
- Published on
Maximizing Productivity with JShell: Common Pitfalls Explained
In the ever-evolving landscape of Java development, JShell has emerged as a powerful tool for programmers seeking to enhance their productivity. This interactive Java REPL (Read-Eval-Print Loop) allows developers to execute code snippets on the fly—an invaluable asset when testing ideas or iterating over code. Despite its advantages, many developers, especially beginners, encounter common pitfalls that can hinder their productivity. In this blog post, we'll explore these pitfalls while providing actionable solutions to help you maximize your productivity using JShell.
What is JShell?
JShell, introduced in Java 9, serves as an interactive command-line tool that simplifies the process of testing Java snippets. Unlike traditional IDEs, JShell allows for rapid feedback and experimentation, enabling developers to try out code without the need for a full project setup.
A typical JShell session involves inputting a line of code, which JShell then evaluates and prints the result. This can significantly speed up learning and prototyping phases in Java development.
Getting Started with JShell
To start JShell, open your command-line interface and type:
jshell
Upon execution, you'll enter the JShell environment. Here, you can define variables, methods, or even classes interactively.
For example, if you want to define a simple variable:
int sum = 5 + 10;
JShell will immediately evaluate and display:
sum ==> 15
Common Pitfalls
Despite its benefits, many users still struggle with JShell. Let's discuss these pitfalls and how to mitigate them.
1. Forgetting to Declare Variables Properly
One of the primary pitfalls newcomers face is forgetting to declare variables in the correct scope. While it might be tempting to quickly type out code without declarations, it’s crucial to ensure your variables can be accessed properly later.
Example
int a = 10;
int b = a + 5;
If you forget to declare a
first and try using it in the next line, you'll receive an error indicating that a
cannot be found:
| b = a + 5;
| ^
Solution
Always declare your variables in the correct sequence. To check what variables you've declared so far, you can use:
/list
2. Missing Imports
Another common hurdle is neglecting to import necessary classes. While JShell imports some packages automatically (like java.lang
), you may run into issues when attempting to use classes like ArrayList
without importing them first.
Example
ArrayList<String> list = new ArrayList<>();
This will throw an error stating that ArrayList cannot be resolved to a type
.
Solution
Before using the class, remember to import it:
import java.util.ArrayList;
3. Misusing Semicolons
Java requires semicolons to terminate statements. In JShell, omitting them can lead to unexpected behavior or errors. This issue is particularly prevalent among developers transitioning from dynamically typed languages, such as Python or JavaScript, where semicolons are often optional.
Example
int c = 5 // Missing semicolon
int d = c + 10;
The above code will result in:
| int c = 5
| ^
Solution
Always end your statements with a semicolon:
int c = 5;
int d = c + 10;
4. Using Code Blocks Without Proper Syntax
In JShell, it can be tempting to write larger blocks of code or entire classes. However, failing to follow Java syntax for classes or methods can waste time troubleshooting unclear error messages.
Example
Trying to define a method:
void greet() {
System.out.println("Hello, world!")
}
This will produce an error related to the missing semicolon.
Solution
When writing methods, always ensure correct syntax. For example, you can modify it to:
void greet() {
System.out.println("Hello, world!");
}
For more complex structures, consider creating complete classes in an IDE where syntax feedback is more robust.
5. Inefficient Use of the Command Line
JShell allows you to run snippets quickly, but many users overlook its command structure which can significantly simplify their workflow. Frequent typing of lengthy code snippets can be tedious.
Solution
JShell provides several command shortcuts:
- /help: Displays available commands.
- /list: Lists all defined variables and methods.
- /exit: Exits the JShell session.
- /reset: Clears all defined variables and methods.
Leverage these commands to maintain an organized workspace and enhance your coding efficiency.
Enhancing Productivity with JShell
Using JShell for Testing and Prototyping
Making the most out of JShell means using it as a testing or prototyping tool rather than a complete development environment. Here’s how you can do this efficiently:
-
Experiment with APIs: Use JShell to explore Java APIs without the need for project setup. For example, try out string manipulation functions dynamically.
-
Test Small Pieces of Code: Instead of writing entire classes for testing, create small code snippets. If you’re unsure about a particular logic segment, break it down and test incrementally:
String name = "Java"; String greeting = "Hello, " + name; System.out.println(greeting);
A Final Look
JShell is a potent tool for enhancing productivity in Java development when used correctly. By avoiding these common pitfalls—like forgetting declarations or misusing command line syntax—developers can significantly improve their workflow.
For a comprehensive guide to mastering JShell, visit the official Oracle documentation.
As you navigate through your coding journey, remember that JRuby's key lies in practice. The more you use JShell, the more familiar you'll become with its workings, and soon it will become a staple in your Java development toolkit. Happy coding!
Checkout our other articles