Key Tricks to Running Methods on Spring Boot Startup!
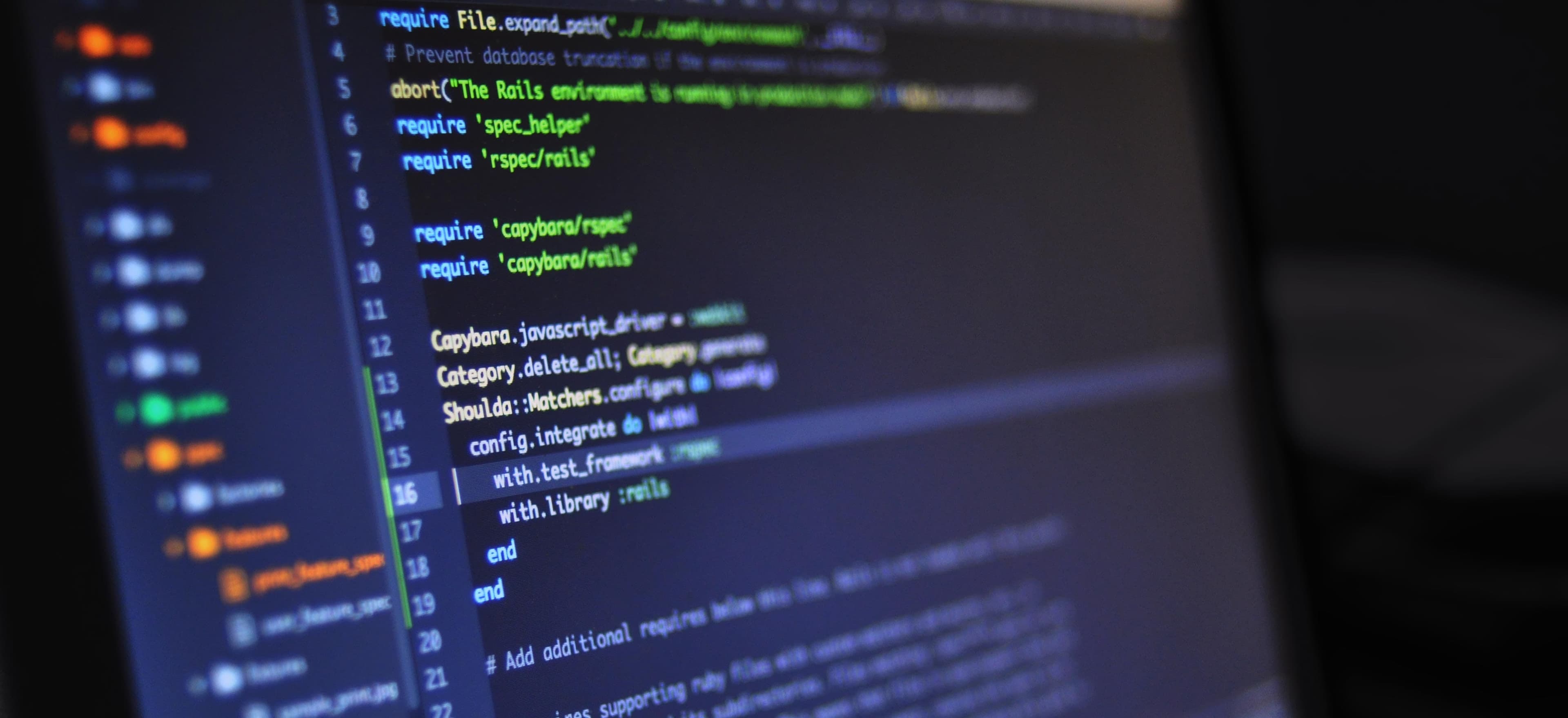
- Published on
Automating Method Execution on Spring Boot Startup
Spring Boot provides flexible and convenient ways to execute methods during its startup process. This capability is particularly useful for performing tasks such as initializing data, setting up configurations, or triggering any process that needs to be done as the application starts up. In this article, we'll explore various approaches to run methods on Spring Boot startup.
Using CommandLineRunner Interface
One of the simplest ways to execute code on Spring Boot startup is by implementing the CommandLineRunner
interface. This interface contains a single method run
, which will be invoked by the Spring Boot application once it has started.
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class MyStartupRunner implements CommandLineRunner {
@Override
public void run(String... args) throws Exception {
// Your startup logic goes here
}
}
By annotating the class with @Component
, we ensure that Spring Boot will automatically detect and run the run
method during startup. This approach is suitable for scenarios where the startup logic is relatively simple and does not require any external dependencies.
Leveraging ApplicationRunner Interface
Similar to CommandLineRunner
, the ApplicationRunner
interface provides a way to execute code upon the startup of a Spring Boot application. The main difference is that ApplicationRunner
gives access to the application arguments as a list of strings, providing more flexibility in processing and utilizing the startup parameters.
import org.springframework.boot.ApplicationArguments;
import org.springframework.boot.ApplicationRunner;
import org.springframework.stereotype.Component;
@Component
public class MyApplicationRunner implements ApplicationRunner {
@Override
public void run(ApplicationArguments args) throws Exception {
// Your startup logic using application arguments
}
}
Utilizing ApplicationRunner
is beneficial when the startup logic requires access to application arguments or when the initialization process is dependent on the provided parameters.
Initializing Bean with CommandLineRunner
Another powerful way to execute methods on Spring Boot startup is by initializing a bean that implements the CommandLineRunner
interface. This method allows for more fine-grained control over the dependencies required for the startup logic.
import org.springframework.boot.CommandLineRunner;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class MyConfiguration {
@Bean
public CommandLineRunner myStartupRunner() {
return args -> {
// Your startup logic goes here
};
}
}
By using the @Configuration
annotation along with @Bean
, we can define a custom method to return an instance of CommandLineRunner
. This approach is beneficial when the startup logic depends on specific dependencies that need to be managed by Spring's inversion of control.
Running Methods with ApplicationEvent
In some cases, it may be necessary to execute methods based on specific application events rather than just during the application startup. Spring Boot provides the ApplicationEvent
and ApplicationListener
interfaces for this purpose.
import org.springframework.context.ApplicationListener;
import org.springframework.context.event.ContextRefreshedEvent;
import org.springframework.stereotype.Component;
@Component
public class MyApplicationListener implements ApplicationListener<ContextRefreshedEvent> {
@Override
public void onApplicationEvent(ContextRefreshedEvent event) {
// Your logic to run on application startup event
}
}
By implementing the ApplicationListener
interface with the appropriate event type, we can specify the exact application event that should trigger the execution of our logic. This method is useful when the startup logic is tied to a specific phase of the application lifecycle rather than the overall startup process.
The Bottom Line
Startling methods on Spring Boot startup is a powerful feature that allows for seamless integration of custom logic into the application initialization process. Whether it's simple one-time initialization or complex event-driven tasks, Spring Boot provides multiple avenues to achieve this.
By leveraging interfaces like CommandLineRunner
and ApplicationRunner
, utilizing application events, or defining custom beans, developers can ensure that their application starts up with the necessary setup and configurations in place.
In summary, understanding the various methods to run code on Spring Boot startup provides a valuable toolset for optimizing the application's initialization process and managing essential tasks from the get-go.
To delve deeper into Spring Boot and its versatile capabilities, check out the official Spring Boot documentation and explore the extensive resources available to enrich your development skills.
Checkout our other articles