Avoiding Memory Leaks with Java ByteBuffers Demystified
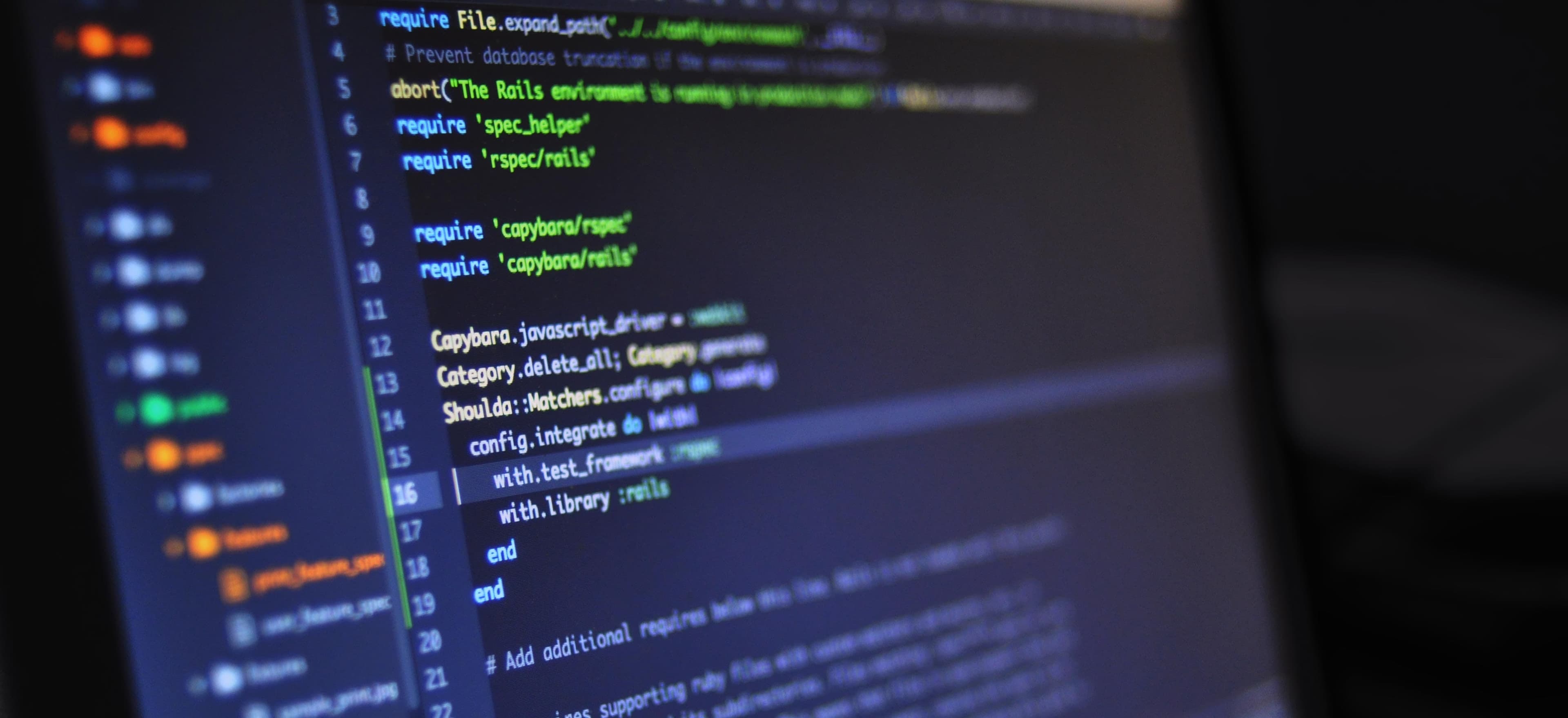
- Published on
Avoiding Memory Leaks with Java ByteBuffers Demystified
Memory leaks in Java can be a nightmare for developers. They can lead to performance issues, unexpected crashes, and in some cases, even compromise the stability of the entire system. In this blog post, we will delve into the world of memory management in Java and specifically focus on how to avoid memory leaks using Java ByteBuffers.
Understanding Memory Leaks in Java
Before we jump into Java ByteBuffers, it's important to understand what memory leaks are and how they occur in Java. In simple terms, a memory leak happens when an application allocates memory but fails to release it when it's no longer needed. This can happen due to various reasons, such as holding onto references longer than necessary or not releasing resources properly.
In Java, memory leaks are often caused by incorrect handling of native memory, particularly when dealing with I/O operations, networking, or direct memory access. This is where Java ByteBuffers come into play as a solution to manage native memory in a more efficient and controlled manner.
Introducing Java ByteBuffers
Java ByteBuffers provide a way to allocate and manage memory outside of the Java heap. They are part of the java.nio package and are especially useful when working with I/O operations, networking, and direct memory access. The ByteBuffer class allows direct access to native memory, which can be leveraged to improve performance and avoid certain types of memory leaks.
Let's take a closer look at how Java ByteBuffers can help us in avoiding memory leaks.
Managing Direct Memory with Java ByteBuffers
When working with I/O operations, it's common to use ByteBuffers to efficiently read from and write to channels. However, it's crucial to manage these ByteBuffers properly to prevent memory leaks.
One common mistake that leads to memory leaks is failing to release direct buffers after they are no longer needed. Unlike Java heap memory, which is managed by the garbage collector, direct memory allocated by ByteBuffers is not automatically released. This means that if you're not careful, you can easily end up with a pile of unreleased native memory, leading to a memory leak.
Let's consider an example where we allocate a direct ByteBuffer to read data from a file:
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
public class FileReadExample {
public static void main(String[] args) {
try (RandomAccessFile file = new RandomAccessFile("example.txt", "r");
FileChannel channel = file.getChannel()) {
ByteBuffer buffer = ByteBuffer.allocateDirect(1024);
// Read data into buffer
while (channel.read(buffer) > 0) {
buffer.flip();
// Process data
buffer.clear();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
In the above example, we allocate a direct ByteBuffer using allocateDirect
to read data from a file. However, once the operation is complete, we need to ensure that the buffer is properly released to avoid leaking native memory.
To release the direct buffer, we can explicitly call the clean
method on the buffer:
buffer.clear();
((sun.nio.ch.DirectBuffer) buffer).cleaner().clean();
By explicitly cleaning the direct buffer, we ensure that the native memory is properly released, thus avoiding a potential memory leak.
While this approach works, it's worth noting that calling clean
directly on the buffer is not generally considered good practice, as it relies on internal implementations and is not part of the public API. In Java 9 and later, the Cleaner API has been introduced to address this and provide a more standardized way of releasing direct buffers.
Using the Cleaner API, we can rewrite the cleanup process as follows:
buffer.clear();
Cleaner cleaner = ((sun.nio.ch.DirectBuffer) buffer).cleaner();
if (cleaner != null) {
cleaner.clean();
}
By leveraging the Cleaner API, we ensure a more robust and standardized approach to releasing direct buffers, thereby reducing the risk of memory leaks.
Closing Thoughts
Memory leaks in Java can be a challenging issue to tackle, especially when dealing with native memory. By understanding the intricacies of Java ByteBuffers and adopting best practices for managing direct memory, developers can mitigate the risk of memory leaks and ensure the stability and performance of their applications.
In this blog post, we've explored how Java ByteBuffers can be used to manage native memory and avoid memory leaks, particularly in the context of I/O operations. We've discussed the importance of properly releasing direct buffers and highlighted the Cleaner API as a more standardized approach to memory management.
By mastering the use of Java ByteBuffers and adhering to best practices for memory management, developers can bolster the robustness and reliability of their Java applications while minimizing the likelihood of memory leaks.
Remember, proactive memory management is key to a healthy and efficient Java application!
As memory management is crucial in Java development, understanding the effective use of Java ByteBuffers is essential. By taking the time to learn and implement best practices, developers can significantly reduce the risk of memory leaks, ensuring the stability and performance of their applications. If you are interested in diving deeper into memory management in Java, consider exploring Java's Memory Management Best Practices for additional insights.