Cracking Java Interviews: Mastering Reference Guide Pt.1
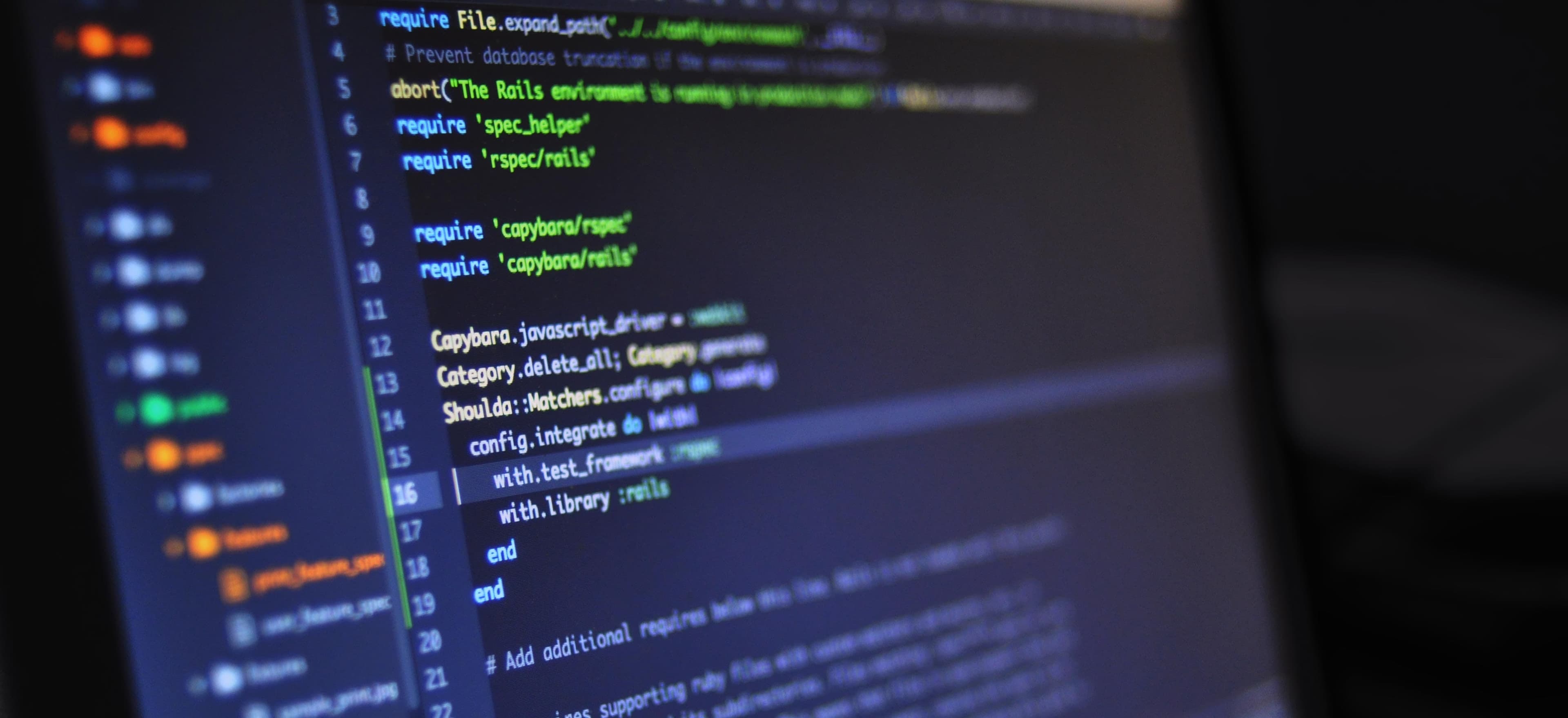
- Published on
Cracking Java Interviews: Mastering Reference Guide Pt.1
If you're a Java developer preparing for an interview, it's essential to have a strong command over references in Java. Understanding references is crucial for memory management and is often a topic of interest in interviews. This guide is designed to help you master Java references and give you an edge in your next interview.
Understanding Java References
In Java, when you create an object using the new
keyword, memory is allocated from the heap, and a reference to that memory location is returned. Understanding the different types of references in Java is vital. Let's delve into the various reference types in Java and their applications.
1. Strong References
Strong references are the default type of reference in Java. When an object has one or more strong references pointing to it, the garbage collector does not reclaim the memory occupied by the object. This is the simplest and most common type of reference.
// Strong reference
MyClass obj = new MyClass();
2. Soft References
Soft references, introduced in Java 2, are less prioritized for garbage collection compared to weak references. They are ideal for creating memory-sensitive caches. When the garbage collector runs out of memory, it clears out soft references.
// Soft reference
SoftReference<MyClass> softRef = new SoftReference<>(new MyClass());
3. Weak References
Weak references are used to avoid memory leaks. They are useful for implementing memory-sensitive data structures. Unlike soft references, weak references are cleared out during the next garbage collection cycle when they are not actively used.
// Weak reference
WeakReference<MyClass> weakRef = new WeakReference<>(new MyClass());
4. Phantom References
Phantom references are unlike the other three types of references. They are enqueued by the garbage collector after the referent has been finalized, but before its memory is reclaimed. Phantom references are often used for cleanup operations after an object is collected.
// Phantom reference
ReferenceQueue<MyClass> queue = new ReferenceQueue<>();
PhantomReference<MyClass> phantomRef = new PhantomReference<>(new MyClass(), queue);
Common Interview Questions on References
Here are some frequently asked interview questions related to Java references:
- What is the difference between strong, soft, weak, and phantom references?
- When would you use a soft reference instead of a weak reference?
- Can you give an example of a scenario where using weak references is beneficial?
- Why would you use phantom references?
- How do you prevent memory leaks when using references in Java?
Preparing for these questions is crucial for performing well in an interview.
Best Practices for Working with References
When working with references in Java, it's important to follow best practices to ensure efficient memory management and avoid memory leaks.
1. Use References Wisely
Avoid over-reliance on strong references, especially in scenarios where memory management is critical. Be mindful of the appropriate use cases for soft, weak, and phantom references to optimize memory usage.
2. Implement Clearing Strategies
For soft and weak references, it's essential to have a proper strategy for re-populating or re-creating the referenced objects if they get cleared. Understanding the implications of reference clearing is key to efficient memory handling.
3. Employ Phantom References for Resource Cleanup
When dealing with resources that require explicit cleanup, such as file handles or network connections, phantom references provide a way to perform cleanup actions before the object is reclaimed by the garbage collector.
Closing Remarks
Mastering Java references is essential for any serious Java developer, especially when preparing for interviews. Understanding the different types of references, their applications, and best practices for working with them gives you a significant advantage. Remember to practice writing code using different types of references and familiarize yourself with common interview questions related to references.
In the next part of this series, we will dive deeper into advanced concepts related to Java references, so stay tuned for more insights.
Start honing your Java skills and impress your next interviewer with your expert knowledge of Java references!
Checkout our other articles