Crafting Clickable Links Inside TextViews: A How-To Guide
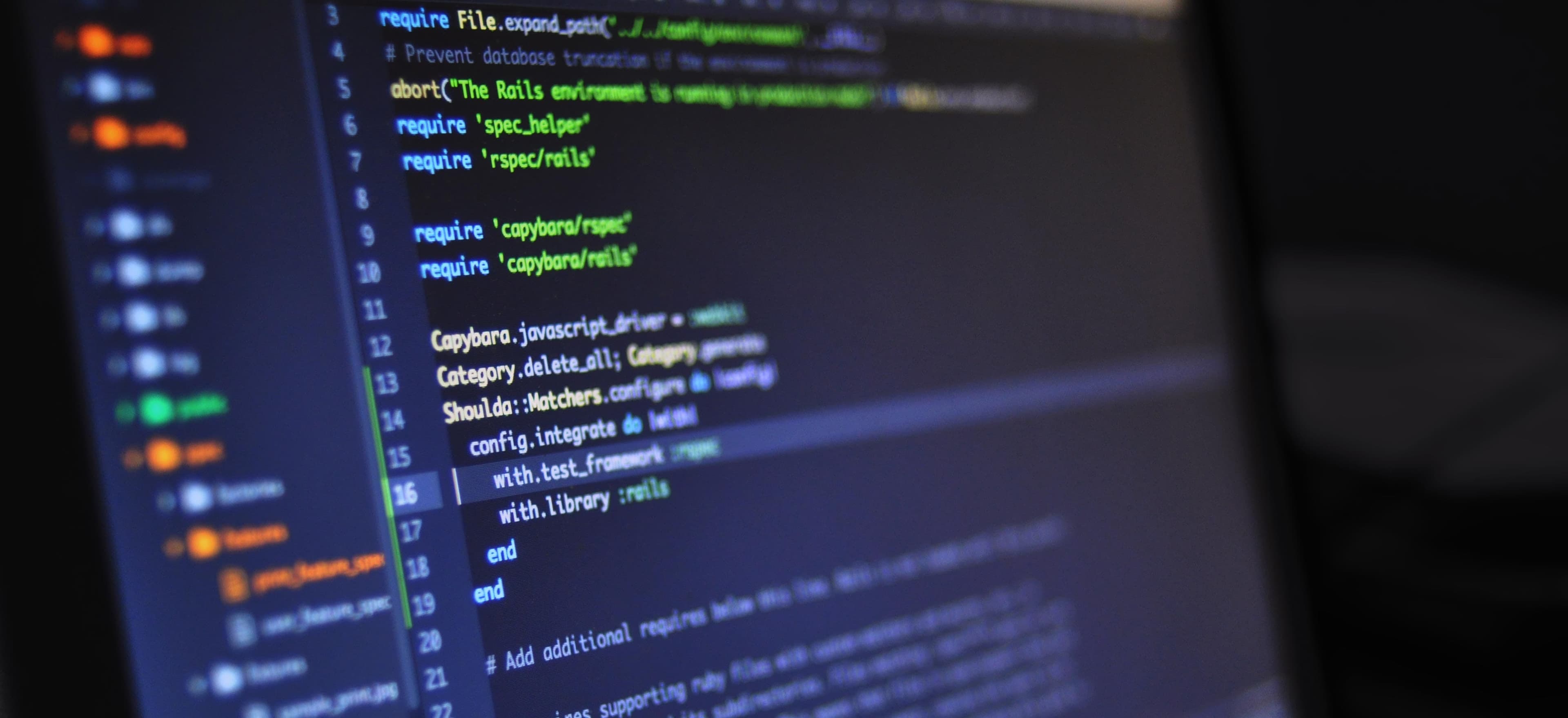
- Published on
Crafting Clickable Links Inside TextViews: A How-To Guide
In the world of Android development, working with TextViews to display text is a common task. Often, there's a need to make certain parts of the text clickable, leading to actions such as opening a web page, dialing a number, or triggering any custom action within the app. In this guide, we'll explore how to achieve this functionality by creating clickable links inside TextViews in a seamless manner.
The Basics of SpannableString
Before diving into clickable links, let's talk about SpannableString. A SpannableString is an immutable object that allows you to define a range of styles for a portion of the text. This can be useful for highlighting, styling, or in our case, making parts of the text clickable.
SpannableString spannableString = new SpannableString("Click here for more information.");
ClickableSpan clickableSpan = new ClickableSpan() {
@Override
public void onClick(View textView) {
// Handle click action here
}
};
spannableString.setSpan(clickableSpan, start, end, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
textView.setText(spannableString);
textView.setMovementMethod(LinkMovementMethod.getInstance());
In the above code, we create a SpannableString with the text "Click here for more information." We then define a ClickableSpan and set it to a specific portion of the text using the setSpan
method, denoted by the start
and end
parameters. This sets up the clickable behavior for that specific portion of text within the TextView. Finally, we set the MovementMethod to LinkMovementMethod, which allows the TextView to recognize and process clickable spans.
Creating Clickable Links
Now that we understand the basics of SpannableString and ClickableSpan, let's create a practical example of clickable links inside a TextView. Suppose we have a TextView displaying the following text: "For more information, visit our [website]." We want the word "website" to be clickable, leading the user to a specific URL when clicked.
SpannableString spannableString = new SpannableString("For more information, visit our website.");
ClickableSpan clickableSpan = new ClickableSpan() {
@Override
public void onClick(View textView) {
// Open the website link here
Uri uri = Uri.parse("https://example.com");
Intent intent = new Intent(Intent.ACTION_VIEW, uri);
startActivity(intent);
}
};
int start = spannableString.toString().indexOf("website");
int end = start + "website".length();
spannableString.setSpan(clickableSpan, start, end, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
textView.setText(spannableString);
textView.setMovementMethod(LinkMovementMethod.getInstance());
Code Explanation
In this code snippet, we create a SpannableString with the provided text. We then define a ClickableSpan to open the website link when clicked. Using indexOf
, we find the position of the word "website" in the text and set the ClickableSpan to that specific portion. Finally, we set the SpannableString to the TextView and enable the LinkMovementMethod to make the "website" clickable.
Handling Click Events
When a user clicks a clickable link inside a TextView, it triggers the onClick
method of the respective ClickableSpan. Inside this method, you can define the action to be performed when the link is clicked. This could be anything from opening a web page, making a phone call, sending an email, or triggering a custom in-app action.
ClickableSpan clickableSpan = new ClickableSpan() {
@Override
public void onClick(View textView) {
// Custom action when the clickable link is clicked
handleCustomAction();
}
};
Making Phone Numbers Clickable
In addition to web links, you may need to make phone numbers clickable, so users can directly initiate a call by clicking on them. This functionality can be achieved using the PhoneNumberUtils and Linkify classes.
TextView textView = findViewById(R.id.textView);
String phoneNumber = "123-456-7890";
Linkify.addLinks(textView, Linkify.PHONE_NUMBERS);
textView.setText(phoneNumber);
A Final Look
In this guide, we've explored the process of creating clickable links inside TextViews in Android. By utilizing SpannableString and ClickableSpan, we can seamlessly make specific parts of the text clickable and define custom actions for when they are clicked. Whether it's directing users to a website, initiating a phone call, or triggering in-app actions, the ability to create clickable links provides a more interactive and engaging user experience within your Android app.
By understanding the fundamentals of SpannableString and ClickableSpan, you can enhance your app's text display with interactive elements, opening up a world of possibilities for user interaction. So go ahead, enrich your TextViews with clickable links and elevate your app's user experience!
Checkout our other articles