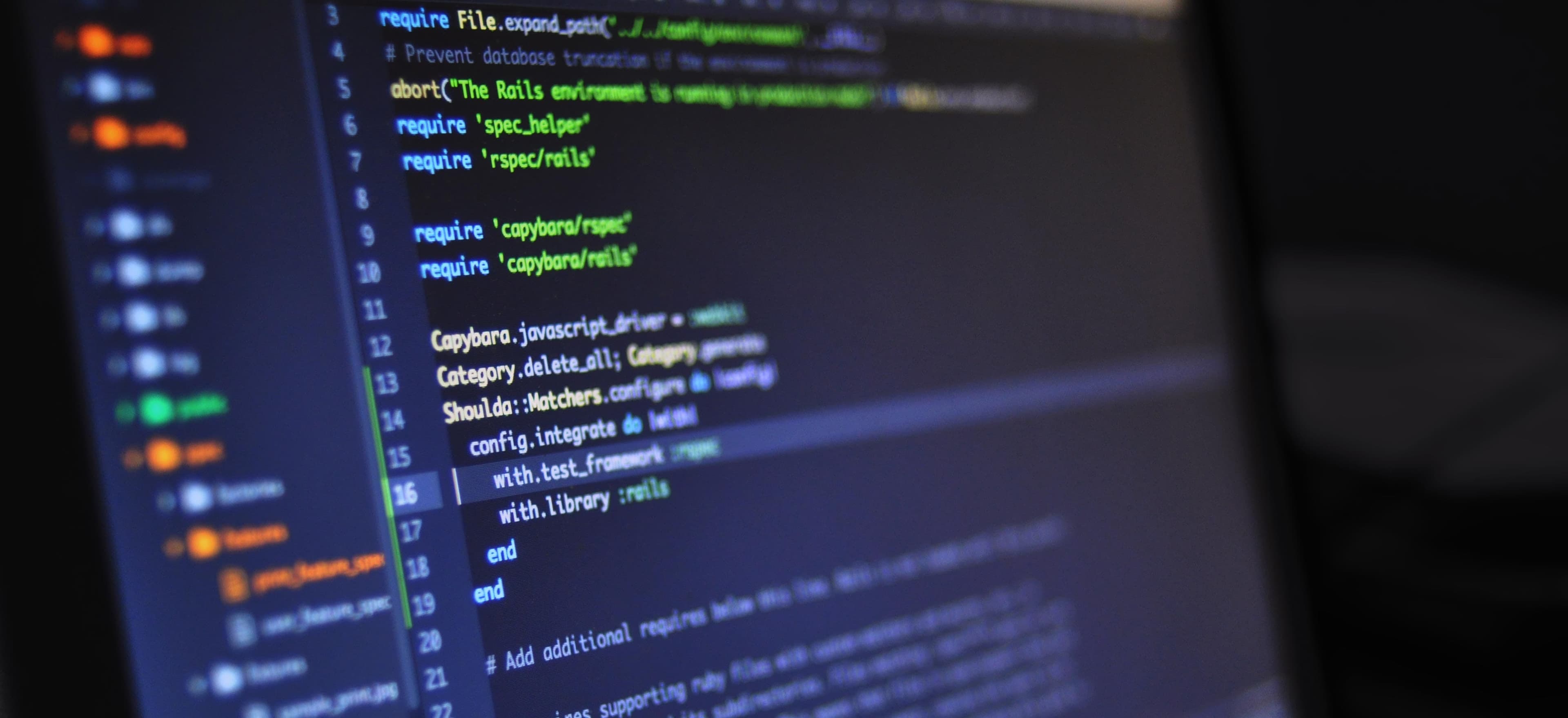
- Published on
Enhancing User Engagement with a Custom TaskProgressView
User engagement is a critical aspect of modern application development. Engaging users not only ensures a pleasant user experience but also contributes to user retention and overall success of the application. In this post, we will explore the creation of a custom TaskProgressView in Java, which can significantly enhance user engagement by providing visual feedback on the progress of background tasks.
Understanding the Importance of User Engagement
User engagement is vital for the success of any application. When users feel engaged, they are more likely to continue using the application, provide positive feedback, and even make in-app purchases. One effective way to boost user engagement is by providing visual feedback on the progress of tasks within the application.
The Challenges of Task Progress Visualization
In many applications, especially those that involve background tasks such as file downloads, data processing, or image loading, there is a lack of visual feedback to the user on the progress of these tasks. This can lead to a disengaged user experience, where the user is uncertain about the status of ongoing tasks.
One effective solution to this challenge is the implementation of a custom TaskProgressView, which provides a visually appealing and informative representation of the task progress.
Building a Custom TaskProgressView in Java
In this section, we will walk through the implementation of a custom TaskProgressView in Java. We will utilize the Swing library for creating the user interface components and update the progress visually.
Setting up the Project
To begin, let's set up a new Java project using an IDE such as IntelliJ IDEA or Eclipse. Once the project is created, we can proceed to create a new Java class for our custom TaskProgressView.
Creating the TaskProgressView Class
import javax.swing.*;
import java.awt.*;
public class TaskProgressView extends JPanel {
private int progress;
public TaskProgressView() {
this.progress = 0;
}
public void setProgress(int progress) {
this.progress = progress;
repaint(); // Trigger repaint when progress is updated
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
int width = (int) (getWidth() * ((double) progress / 100));
g.setColor(Color.BLUE);
g.fillRect(0, 0, width, getHeight());
}
}
In the above code, we define a TaskProgressView
class that extends JPanel
. We maintain the progress value and override the paintComponent
method to visually represent the progress using a blue-colored bar.
Implementing the TaskProgressView
Now, let's integrate the TaskProgressView
into an example application to demonstrate its usage. We will create a simple Swing application with a button that simulates a background task and updates the progress on the TaskProgressView
.
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TaskProgressDemo {
public static void main(String[] args) {
JFrame frame = new JFrame("Task Progress Demo");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(400, 200);
TaskProgressView progressView = new TaskProgressView();
JButton startButton = new JButton("Start Task");
startButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Simulate task progress
for (int i = 0; i <= 100; i++) {
try {
Thread.sleep(100); // Simulate task processing time
} catch (InterruptedException ex) {
ex.printStackTrace();
}
progressView.setProgress(i); // Update progress
}
}
});
frame.add(progressView, BorderLayout.CENTER);
frame.add(startButton, BorderLayout.SOUTH);
frame.setVisible(true);
}
}
In the above code, we create a simple Swing application with a TaskProgressView
and a "Start Task" button. When the button is clicked, it simulates a background task by iterating from 0 to 100 and updating the progress on the TaskProgressView
.
Closing Remarks
In this post, we explored the importance of user engagement in application development and addressed the challenges of visualizing task progress. By creating a custom TaskProgressView
in Java, we demonstrated a practical approach to enhance user engagement and provide visual feedback on background tasks.
With the implementation of a custom TaskProgressView, developers can significantly improve the user experience, leading to higher user engagement and overall satisfaction with the application.
By providing visual feedback on the progress of tasks, applications become more intuitive and user-friendly, ultimately resulting in a positive impact on user engagement and retention.
In conclusion, creating a custom TaskProgressView is a valuable addition to any Java application, contributing to a more engaging and user-friendly experience.
Start enhancing user engagement with your custom TaskProgressView now!
Checkout our other articles