Debugging Quartz Schedule: Solving Cron Expression Errors
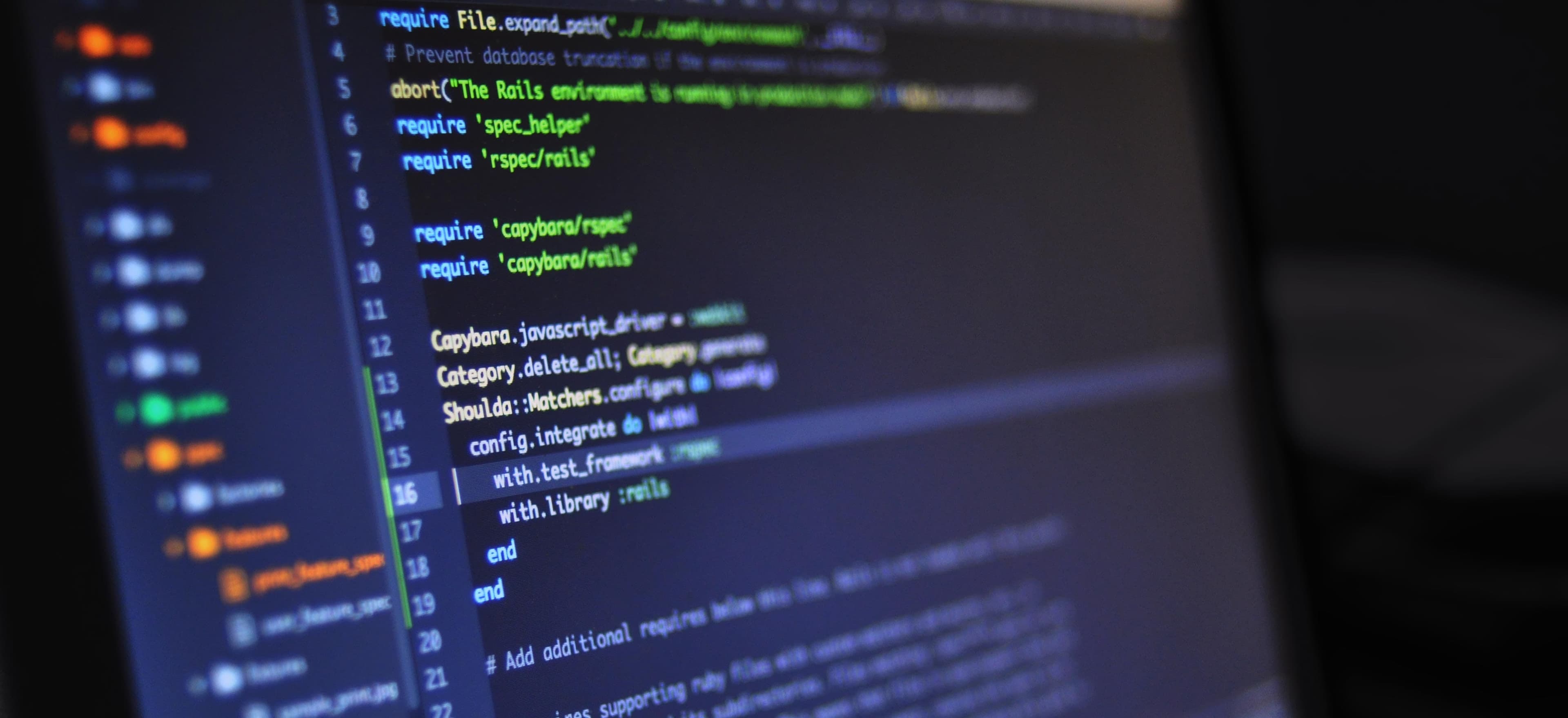
- Published on
Debugging Quartz Schedule: Solving Cron Expression Errors
Quartz is a powerful job scheduling library for Java applications. It allows developers to set up jobs that execute at pre-defined schedules using cron expressions. However, working with cron expressions can sometimes lead to errors that can be challenging to debug. In this post, we'll explore common cron expression errors in Quartz and how to effectively debug and solve them.
Understanding Cron Expressions
Before diving into debugging cron expression errors in Quartz, let's quickly review what cron expressions are and how they work.
A cron expression is a string representing a schedule. It consists of six or seven fields that represent various time units such as seconds, minutes, hours, day of month, month, day of week, and an optional year field. These fields are separated by white space, and a cron expression looks something like this:
0 0/5 * 1/1 * ? *
This example cron expression represents a schedule that runs every 5 minutes.
Common Errors with Cron Expressions
When working with Quartz, you may encounter several common errors related to cron expressions. Some of these errors include:
-
Incorrect Field Order: The order of fields in a cron expression is crucial. Placing the fields in the wrong order will result in a parsing error.
-
Syntax Errors: Typos or incorrect syntax within a cron expression can lead to parsing failures.
-
Invalid Values: Providing invalid values for time units within the cron expression, such as specifying 60 for seconds, can cause parsing errors.
-
Misunderstanding Special Characters: Misinterpreting special characters like "/" or "-" can lead to unexpected behavior in the cron expression.
Debugging Cron Expression Errors in Quartz
When faced with cron expression errors in Quartz, it's essential to have a structured approach to debugging. Here are some steps to effectively debug and solve cron expression errors:
-
Validate Cron Expressions: Use a cron expression validator tool or an online cron expression parser to validate the syntax and field values of your cron expression. Ensure that the expression is correctly formatted and does not contain any syntax errors.
-
Check Field Order: Double-check the order of fields in your cron expression to ensure they align with the expected sequence of seconds, minutes, hours, day of month, month, and day of week.
-
Review Special Characters: Understand the meaning of special characters within cron expressions and ensure they are being used correctly. For example, "/" is used to specify increments, while "-" is used to specify ranges.
-
Test Incrementally: When debugging a complex cron expression, consider starting with a simple expression representing a narrow time window and gradually expanding it to the desired schedule. This incremental approach can help pinpoint where the issue lies.
Example: Debugging a Cron Expression Error
Let's consider an example where we encounter a cron expression error in Quartz. Suppose we have the following cron expression:
0/60 * * 1/1 * ? *
This expression is intended to represent a schedule that runs every 60 seconds. However, it contains a critical error in the first field. The use of "0/60" suggests running the job every 0 seconds within a minute, which is invalid.
To debug this error, we can first validate the expression using a cron expression validator. Upon validation, we'd identify the incorrect use of "0/60" and replace it with a simple "*" to represent every second within a minute:
* * * 1/1 * ? *
By following the steps outlined earlier, we've successfully debugged and corrected the cron expression to achieve the intended schedule.
The Last Word
Debugging cron expression errors in Quartz is a crucial skill for Java developers working with job scheduling. By understanding the common errors and following a structured debugging approach, developers can effectively identify and solve cron expression issues in their applications. Remember to validate expressions, check field order, review special characters, and test incrementally to pinpoint and resolve errors.
By mastering the art of debugging cron expressions in Quartz, developers can ensure their scheduled jobs run smoothly and reliably, contributing to the overall robustness of their Java applications.
In summary, dealing with cron expression errors in Quartz involves a meticulous process of validation, understanding, and correction, ultimately leading to optimized job scheduling and enhanced application functionality.
For further insights into Quartz and job scheduling in Java, I recommend exploring the official Quartz Scheduler Documentation and the Quartz GitHub Repository for in-depth resources and community support.
Happy debugging!
Checkout our other articles