Cracking the Code: Maximizing Your Java Bundle in 2020
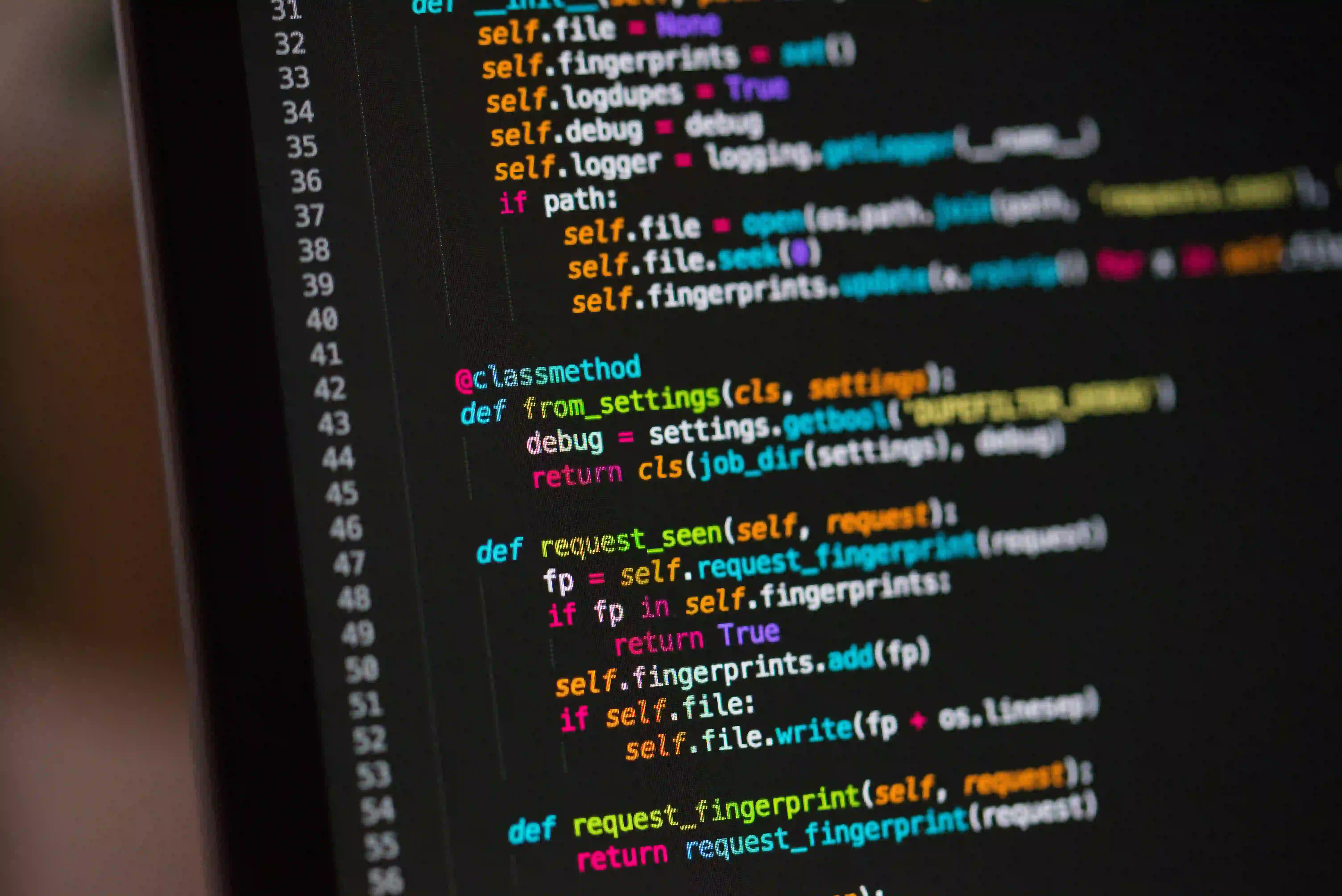
Maximizing Your Java Bundle in 2020
Java is a popular programming language renowned for its versatility and robustness, making it a prime choice for developing everything from mobile apps to enterprise systems. As we venture into 2020, it's crucial to explore strategies for optimizing our Java codebase to ensure peak performance and maintainability.
Understanding Java Bundles
In the context of Java, a bundle refers to packaging the code, resources, and configuration files into a single archive file. This bundle, commonly in the form of a JAR (Java ARchive), is integral for distributing and running Java applications.
Why Optimize Java Bundles?
Efficient bundling offers numerous benefits, including reduced load times, improved runtime performance, and simplified deployment. By optimizing our Java bundles, we can streamline the application's footprint, minimize startup overhead, and enhance the overall user experience.
Fundamental Techniques for Java Bundle Optimization
Let's delve into practical techniques to maximize our Java bundles, focusing on minimizing size, accelerating startup, and ensuring maintainability.
1. Minimizing Size with Code Obfuscation
Utilizing code obfuscation tools like ProGuard can significantly reduce the size of our Java bundles by renaming classes, fields, and methods to shorter names. This not only shrinks the bundle size but also hampers reverse engineering attempts.
Example:
// ProGuard configuration file
-optimizationpasses 5
-dontusemixedcaseclassnames
-dontskipnonpubliclibraryclasses
-dontpreverify
-verbose
-optimizations !code/simplification/arithmetic,!field/*,!class/merging/*
2. Accelerating Startup with Just-In-Time (JIT) Compilation
Employing JIT compilation can accelerate the startup time of Java applications. JIT compilers dynamically optimize bytecode to native machine code during runtime, reducing the initial overhead.
Example:
public class Application {
public static void main(String[] args) {
// Application logic
}
}
3. Ensuring Maintainability with Modularization
Adopting modularization through Java 9's module system fosters maintainability by encapsulating code into distinct modules. This promotes code organization and simplifies dependency management.
Example:
module com.example.app {
requires module1;
exports com.example.app.feature;
}
Advanced Approaches for Java Bundle Optimization
In addition to fundamental techniques, advanced strategies can further optimize Java bundles for superior performance and efficiency.
1. Embracing Ahead-Of-Time (AOT) Compilation
Integrating AOT compilation with tools like GraalVM ahead of application deployment can yield significant performance gains by pre-compiling Java code to native machine code.
2. Leveraging Tree Shaking for Dead Code Elimination
Utilizing tools such as ProGuard's tree shaking capabilities can eliminate dead code from our bundles, further reducing their size and enhancing runtime efficiency.
Example:
// ProGuard configuration for tree shaking
-keep public class com.example.app.Main {
public static void main(java.lang.String[]);
}
3. Employing Lazy Loading for On-Demand Class Initialization
Implementing lazy loading techniques enables classes to be loaded only when required, enhancing application startup times and memory utilization.
Example:
public class LazyInitialization {
private static HeavyResource resource;
public static HeavyResource getResource() {
if (resource == null) {
resource = new HeavyResource();
}
return resource;
}
}
Best Practices and Tools for Java Bundle Optimization
Adhering to best practices and leveraging specialized tools is vital for sustained Java bundle optimization.
Best Practices:
- Regularly perform code reviews and refactor redundant or bloated code to maintain a lean codebase.
- Employ efficient algorithms and data structures to optimize runtime performance and memory utilization.
- Continuously monitor bundle size and performance metrics, and iterate on optimization strategies.
Tools:
- ProGuard: An open-source tool for shrinking, optimizing, and obfuscating Java code.
- GraalVM: A high-performance runtime that provides AOT compilation and native image generation.
- JProfiler: A powerful Java profiler for identifying performance bottlenecks and memory leaks.
Closing Remarks
Optimizing Java bundles is a continuous endeavor that demands a blend of fundamental techniques, advanced strategies, best practices, and specialized tools. By embracing these optimization methodologies, developers can ensure that their Java applications deliver exceptional performance, maintainability, and user satisfaction in 2020 and beyond.