Boosting Performance: Immutable vs Persistent Collections
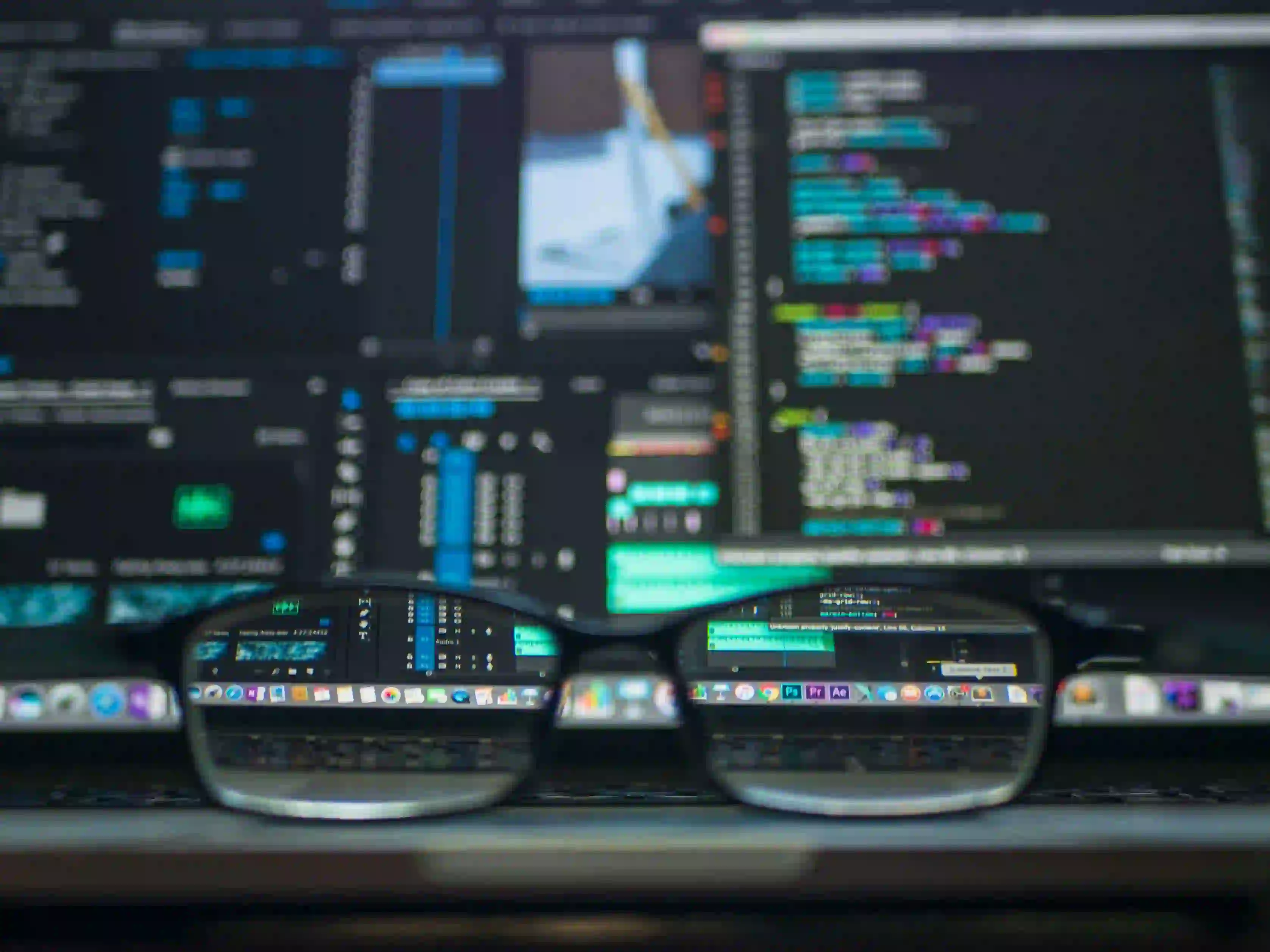
Boosting Performance: Immutable vs Persistent Collections
When it comes to improving the performance of your Java applications, choosing the right data structure can make a significant difference. Immutable and persistent collections are two popular options that offer benefits in terms of immutability, safety, and performance. In this post, we'll explore the differences between immutable and persistent collections, and discuss their impact on performance in Java applications.
What are Immutable Collections?
Immutable collections are data structures whose state cannot be modified after they are created. In Java, the Collections.unmodifiableXXX()
methods are used to create immutable versions of standard collections such as List
, Set
, and Map
. Once an immutable collection is created, attempts to modify it will result in UnsupportedOperationException
.
Example of creating an immutable list in Java:
List<String> mutableList = new ArrayList<>();
mutableList.add("Apple");
mutableList.add("Banana");
List<String> immutableList = Collections.unmodifiableList(mutableList);
In the example above, immutableList
cannot be modified, ensuring that its state remains consistent throughout the application. Immutability provides safety and thread-safety, as it eliminates the risk of concurrent modification exceptions and unintended changes.
What are Persistent Collections?
Persistent collections, on the other hand, are data structures that maintain previous versions of themselves when modified. In Java, libraries such as PCollections provide persistent collection implementations such as PVector
, PSet
, and PMap
. When new elements are added, removed, or updated in a persistent collection, a new version of the collection is created, while the original version remains unmodified.
Example of creating a persistent vector in Java using PCollections:
PVector<String> originalVector = TreePVector.empty();
PVector<String> newVector = originalVector.plus("Apple").plus("Banana");
In this example, originalVector
remains unchanged, while newVector
represents the updated version with "Apple" and "Banana" added.
Performance Considerations
Immutable Collections Performance
Immutable collections excel in scenarios where data consistency and safety are critical. However, their performance can be a concern when it comes to modifying large collections. In such cases, creating a new copy of the entire collection whenever a modification occurs can lead to memory and processing overhead.
Why It Matters:
- Immutable collections, by design, ensure data integrity and thread safety.
- Immutability simplifies multithreading, as there is no risk of concurrent modification.
Persistent Collections Performance
Persistent collections, with their structural sharing behavior, can offer better performance in scenarios involving frequent modifications. When elements are added, removed, or updated, persistent collections leverage sharing of unchanged portions with previous versions, reducing memory consumption and improving performance.
Why It Matters:
- Persistent collections allow efficient sharing of unchanged portions, minimizing memory overhead.
- Better suited for applications where frequent modifications are expected.
Choosing the Right Approach
Use Immutable Collections When:
- Data consistency and safety are top priorities.
- Concurrent access to collections is prevalent.
- The size of the collection is relatively small, and modifications are infrequent.
Use Persistent Collections When:
- Performance during modifications is crucial.
- Large collections are involved, and modifications are frequent.
- Efficient memory management is a priority.
Key Takeaways
In conclusion, the choice between immutable and persistent collections in Java should be driven by the specific requirements of your application. Immutable collections offer safety and consistency, making them suitable for scenarios where modifications are infrequent and data integrity is paramount. On the other hand, persistent collections provide efficient performance during frequent modifications and are better suited for handling large data sets.
Carefully evaluating the trade-offs between immutability and performance can lead to the selection of the most suitable data structure for your application, ultimately contributing to an optimized and well-performing system.
By understanding the nuances of immutable and persistent collections, Java developers can make informed decisions to enhance the performance and reliability of their applications.
For further exploration, consider diving into the complexities of Java Collections Framework. Additionally, you might want to explore PCollections or other persistent collection libraries to gain hands-on experience with persistent data structures in Java.