Avoiding Common JUnit Pitfalls: A Programming Guide
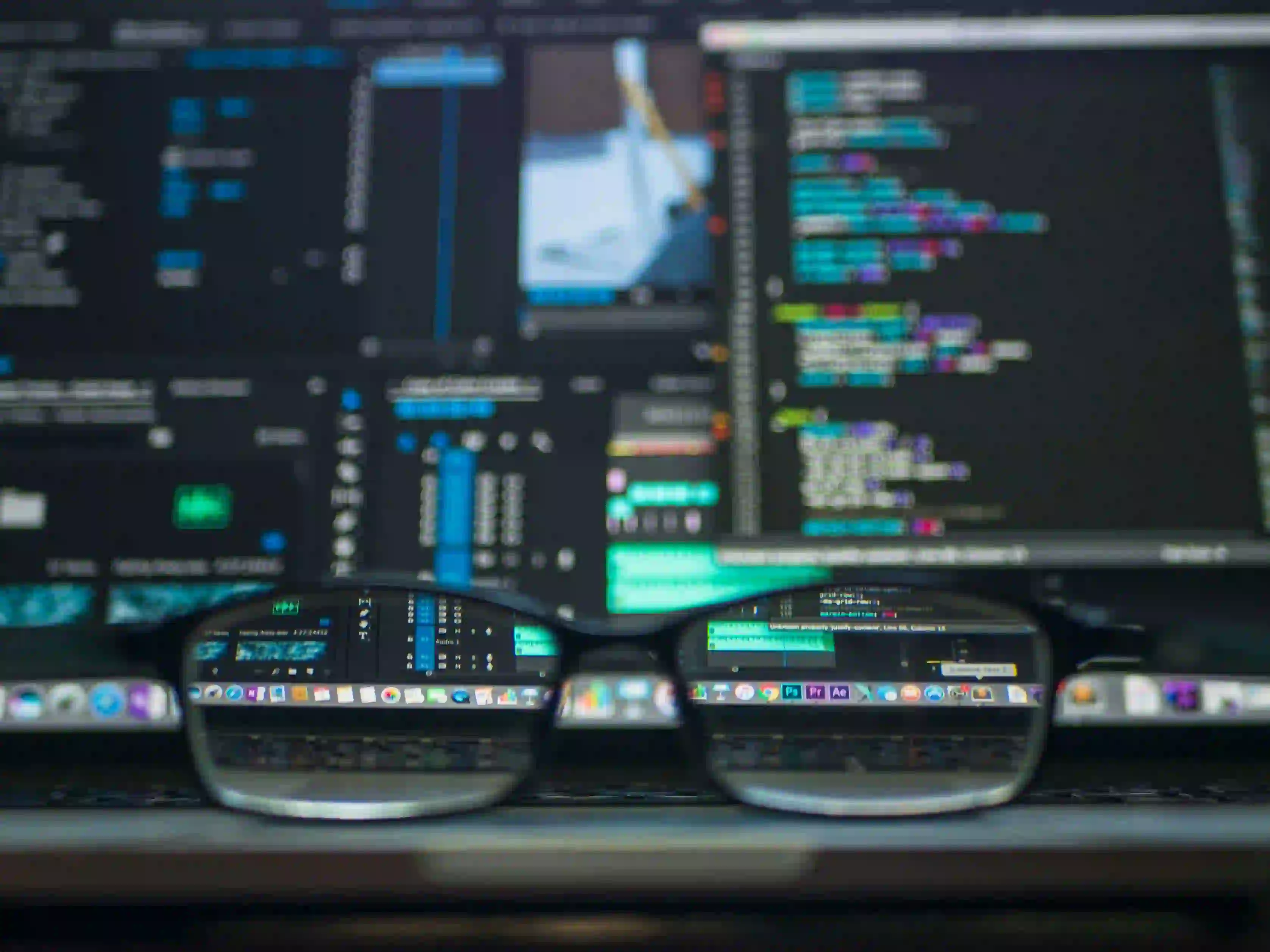
Avoiding Common JUnit Pitfalls: A Programming Guide
Diving Into the Subject
JUnit is a popular unit testing framework in the Java ecosystem, playing a key role in ensuring high-quality, bug-free code. However, using JUnit effectively can be challenging, and developers often encounter common pitfalls that affect the reliability and maintainability of their test suites. In this guide, we'll explore these pitfalls and provide insights into best practices to help you avoid them, ensuring that your unit tests are robust, reliable, and effective.
What is JUnit?
JUnit is a widely-used unit testing framework for Java, deeply rooted in the principles of test-driven development (TDD). Its purpose is to provide a structured and repeatable way to test individual units of source code. The latest version of JUnit, as of writing, is JUnit 5, which introduces significant advancements over its predecessors, including improved support for parameterized tests, dynamic tests, and test suites.
Common Pitfalls in JUnit Testing
Ignoring Test Naming Conventions
One common pitfall in JUnit testing is ignoring the importance of test naming conventions. Meaningful and descriptive test names significantly aid in understanding test failures and errors, making it easier to pinpoint issues in the code.
// Poorly named test
@Test
void testAdd() {
// test logic
}
// Well-named test
@Test
void shouldReturnSum_whenAddingTwoNumbers() {
// test logic
}
In the example above, the poorly named test provides no insight into what's being tested, while the well-named test clearly expresses the expected behavior.
Not Isolating Test Cases
Test isolation is crucial to ensure that tests remain independent of each other, mitigating side effects and the risk of one test impacting the execution of another. It also prevents unpredictable behavior and flakiness in the test suite.
// Shared state between tests
class CalculatorTest {
private Calculator calculator;
@BeforeEach
void setup() {
calculator = new Calculator();
}
@Test
void testAdd() {
// test logic using calculator
}
@Test
void testSubstract() {
// test logic assuming no side effects from previous test
}
}
In this example, the calculator
state is shared between tests, potentially leading to unexpected outcomes in the second test.
Overuse of Mocks
While mocks are valuable for isolating code under test, overusing them can lead to tests that are too tightly coupled to the implementation details of the code. Using real instances when possible and resorting to mocks only for external dependencies or complex interactions tends to result in more robust and effective tests.
// Overuse of mocks
@Test
void testCalculateTotal_withMocks() {
when(orderRepository.getOrders()).thenReturn(Collections.singletonList(order));
// test logic
}
In this example, the use of mocks makes the test overly reliant on the internal details of the orderRepository
, making the test fragile.
Testing Private Methods
Directly testing private methods may indicate a need to reconsider the class's design or the organization of responsibilities. Instead of testing private methods in isolation, consider testing through public interfaces, as they are more indicative of the class's externally observable behavior.
// Private method under test
private int add(int a, int b) {
// implementation
}
// Consider testing through public interface
@Test
void shouldReturnSum_whenAddingTwoNumbers() {
// test the public method that utilizes the private logic
}
Testing through the public interface provides better insight into the behavior of the class while also promoting more maintainable and resilient tests.
Skipping or Ignoring Tests
It's tempting to skip failing tests using @Ignore
or similar annotations, especially when under time pressure. However, skipping or ignoring failing tests can lead to a decline in the test suite's effectiveness and, in the worst cases, to an environment where failing tests are regularly overlooked.
@Ignore
@Test
void testFeatureUnderDevelopment() {
// test logic
}
Using @Ignore
without a clear plan to address the failing test can lead to long-standing issues in the codebase. Instead, focus on promptly addressing failing tests and using continuous integration systems to prevent passing on failing tests.
Advanced JUnit Features You Should Be Using
Parameterized Tests
Parameterized tests allow you to run the same test logic with different inputs, reducing code duplication and making it easier to add new test cases.
@ParameterizedTest
@ValueSource(ints = {1, 2, 3})
void shouldReturnTrueForPositiveNumbers(int number) {
assertTrue(number > 0);
}
In this parameterized test example, the same test logic is executed with different input values, improving test coverage and reducing repetitive code.
Test Suites
Test suites enable you to bundle multiple test classes together and execute them as a group. This is particularly useful when organizing and running a comprehensive set of tests for a particular module or feature.
@RunWith(Suite.class)
@SuiteClasses({CalculatorTest.class, MathFunctionsTest.class})
public class AllTests {
// This class remains empty. It's used only as a holder for the above annotations.
}
The AllTests
class serves as a test suite, combining the CalculatorTest
and MathFunctionsTest
classes and executing them together.
Dynamic Tests
Dynamic tests, introduced in JUnit 5, allow for the generation of tests at runtime, enabling more flexibility in test execution and supporting scenarios where the exact number of tests isn't known until runtime.
@TestFactory
Stream<DynamicTest> testDynamicAddition() {
return Stream.of(1, 2, 3)
.map(number ->
dynamicTest("Test adding " + number,
() -> assertEquals(number, number + 0))
);
}
In the example above, dynamic tests are dynamically generated based on the input numbers, offering a flexible approach to test case generation.
Best Practices for Effective JUnit Testing
Keep Tests Readable and Maintainable
When writing unit tests, focus on creating self-explanatory test names and assertions, reducing the need for excessive comments. Good test names and well-crafted assertions enhance test maintainability by providing clear documentation for the code's expected behavior.
Balance Between Unit and Integration Tests
Striking a balance between unit and integration tests is crucial for an effective testing strategy. Unit tests should focus on testing individual units in isolation, while integration tests cover the interactions between the units. Maintaining an appropriate mix ensures both depth and breadth in test coverage.
Use Assertions Effectively
Choose assertion types that best express the intent of the test and make them as informative as possible by adding custom messages. This practice helps to enhance the clarity of test failures, aiding in quick issue resolution.
// Well-crafted assertion with a custom message
assertEquals("The result should be 100", 100, result);
My Closing Thoughts on the Matter
In conclusion, JUnit is a powerful tool for ensuring software quality through robust unit testing, and adopting best practices is crucial for maximizing its benefits. By avoiding common pitfalls and leveraging advanced features, developers can drive their testing efforts towards more reliable, maintainable, and effective unit tests. Continuously adapting to best practices and leveraging the full potential of JUnit will lead to better testing strategies and improved software quality.
Further Reading and Resources
For further exploration of JUnit and effective unit testing in Java, consider the following resources:
- Official JUnit 5 Documentation
- Advanced JUnit Tutorials on Baeldung
- Practical Unit Testing with JUnit and Mockito (Book)
These resources provide valuable insights into practical and efficient unit testing in Java, helping you deepen your understanding of JUnit and strengthen your testing skills.
In conclusion, understanding and effectively utilizing JUnit can significantly enhance the quality and reliability of your Java code. By avoiding common pitfalls, incorporating advanced features, and following best practices, you can create a robust testing infrastructure that strengthens your software development process. Remember, the journey to writing exceptional code is paved with continuous learning and adaptation of best practices.