Unlocking the Puzzle: Testing Multithreaded Java Code
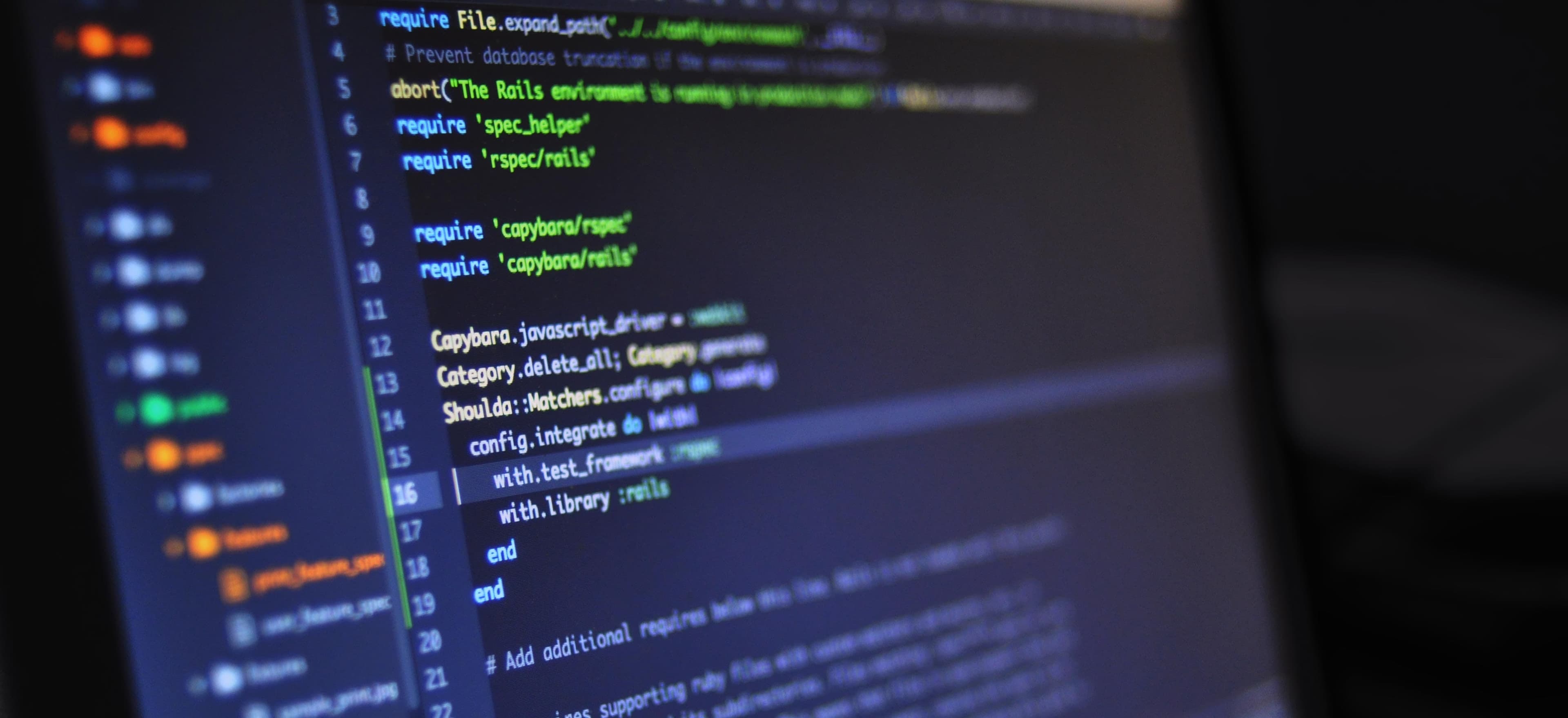
- Published on
Unlocking the Puzzle: Testing Multithreaded Java Code
Multithreading is a powerful feature in Java, allowing applications to perform multiple tasks concurrently. However, with more threads running simultaneously, the potential for race conditions, deadlocks, and other concurrency issues increases. This makes testing multithreaded Java code vital for ensuring the stability and correctness of our applications.
In this post, we'll delve into the strategies and best practices for testing multithreaded Java code, covering techniques such as Thread.sleep(), CountDownLatch, and CompletableFuture to write effective multithreaded tests. We'll also explore the use of tools like JUnit and Mockito to mock and verify multithreaded behavior in our tests.
Understanding the Challenges of Testing Multithreaded Java Code
When testing multithreaded Java code, there are several challenges that need to be addressed:
-
Non-deterministic behavior: Multithreaded code introduces non-deterministic behavior, making it difficult to predict the order of execution and potential race conditions.
-
Concurrency issues: Concurrent access to shared resources can lead to data corruption, deadlocks, and other concurrency-related problems.
-
Complexity: Writing test cases for multithreaded code can be complex and require a deep understanding of threading concepts.
Writing Effective Multithreaded Tests
Using Thread.sleep() for Synchronization
One way to test multithreaded code is to use the Thread.sleep() method to introduce delays, allowing certain threads to complete their work before verifying the results. While this method can be simple and effective, it has some limitations. It can make tests slower, and it doesn’t guarantee the order of execution, leading to potential flakiness in the tests.
Here's an example of using Thread.sleep() for testing multithreaded code:
@Test
public void testMultithreadedCode() throws InterruptedException {
SomeClass obj = new SomeClass();
Thread t1 = new Thread(() -> {
// Perform some operation
obj.someMethod();
});
t1.start();
Thread.sleep(1000); // Introduce delay to ensure completion
assertTrue(obj.getResult());
}
Leveraging CountDownLatch for Synchronization
Another approach is to use CountDownLatch, which allows one or more threads to wait until a set of operations being performed in other threads completes.
@Test
public void testMultithreadedCodeWithCountDownLatch() throws InterruptedException {
CountDownLatch latch = new CountDownLatch(1);
SomeClass obj = new SomeClass(latch);
Thread t1 = new Thread(() -> {
// Perform some operation
obj.someMethod();
latch.countDown();
});
t1.start();
latch.await(); // Wait for latch to be counted down
assertTrue(obj.getResult());
}
Using CountDownLatch provides more control and avoids hardcoding delays, leading to more reliable and efficient tests.
Harnessing CompletableFuture for Asynchronous Testing
With the introduction of CompletableFuture in Java 8, testing asynchronous and multithreaded code has become more streamlined. CompletableFuture allows us to write tests that wait for asynchronous operations to complete, enabling a more natural and expressive way of testing multithreaded code.
@Test
public void testMultithreadedCodeWithCompletableFuture() {
SomeClass obj = new SomeClass();
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// Perform some operation
obj.someMethod();
});
future.join(); // Wait for the asynchronous operation to complete
assertTrue(obj.getResult());
}
Using CompletableFuture simplifies the testing of asynchronous code and provides a more fluent and readable testing approach.
Leveraging Tools for Multithreaded Testing
JUnit and Multithreaded Testing
JUnit, the de facto standard for unit testing in Java, provides support for writing multithreaded tests. By using the @Test annotation along with assertions and synchronization mechanisms, we can create robust multithreaded test cases.
@Test
public void testMultithreadedCodeWithJUnit() {
// Create and start multiple threads
// Perform multithreaded operations
// Assert expected results
assertTrue(true);
}
By utilizing JUnit's capabilities, we can structure and organize multithreaded tests effectively, ensuring clarity and maintainability.
Mockito for Mocking Multithreaded Behavior
Mockito, a popular mocking framework for Java, can be used effectively in multithreaded testing scenarios. By mocking dependencies and controlling their behavior, we can simulate multithreaded interactions and verify the expected behavior.
@Test
public void testMultithreadedCodeWithMockito() {
SomeDependency mockDependency = mock(SomeDependency.class);
// Stub/mock behavior for multithreaded interactions
// Perform multithreaded operations using the mocked dependency
// Verify the expected interaction and behavior
verify(mockDependency, times(1)).someMethod();
}
Mockito enables us to isolate multithreaded behavior, focus on specific interactions, and verify the expected behavior within our tests.
Key Takeaways
Testing multithreaded Java code is essential for ensuring the stability and reliability of our applications. By understanding the challenges and leveraging effective techniques and tools such as Thread.sleep(), CountDownLatch, CompletableFuture, JUnit, and Mockito, we can write comprehensive and robust multithreaded tests.
Testing multithreaded code may be challenging, but with the right approach and tools, we can unlock the puzzle and ensure that our multithreaded Java applications perform optimally, even under concurrent and asynchronous conditions.
Incorporating best practices and embracing the power of multithreaded testing, we can confidently navigate the complexities of concurrent programming in Java, ensuring the integrity and resilience of our applications.
As we continue to push the boundaries of concurrent and parallel programming, testing multithreaded Java code will remain an indispensable aspect of software development, enabling us to conquer the intricacies and complexities of multithreading with precision and confidence.
Unlock the power of multithreaded testing, and unleash the potential of your Java applications!
So, what are your experiences with multithreaded testing in Java? Share your thoughts and insights in the comments below!
To deepen your understanding of multithreading in Java, check out this resource on Java Multithreading and Concurrency in Java.
Feel free to explore more about the intricacies of testing multithreaded code and the nuances of concurrent programming in Java.