Extracting Image Metadata in Docker with Spring Boot
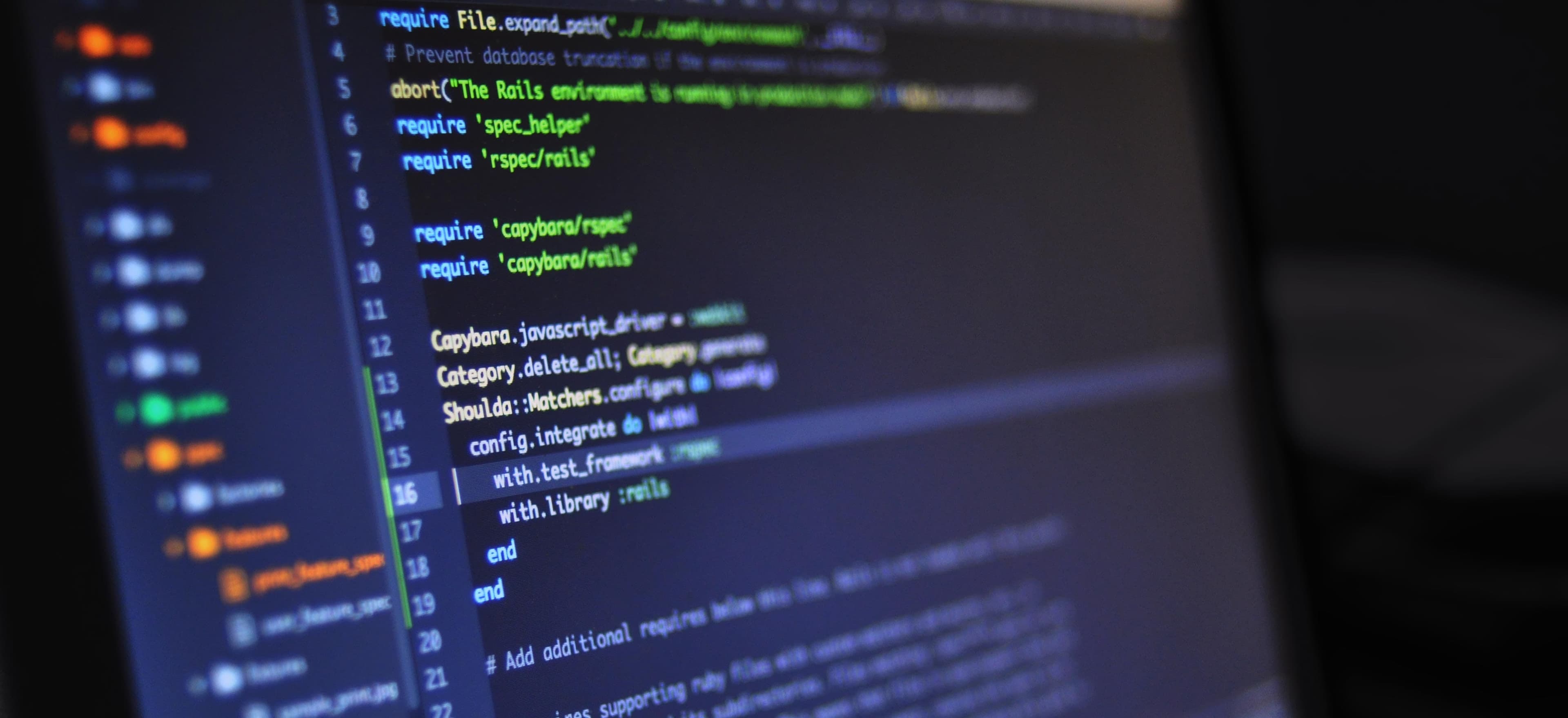
- Published on
Understanding Image Metadata Extraction in Docker with Spring Boot
When it comes to managing and extracting image metadata in a Docker environment using Spring Boot, Java provides powerful tools and libraries to simplify the process. In this article, we will delve into the concept of image metadata, explore how to work with Docker in a Spring Boot application, and demonstrate the extraction of image metadata using Java.
Understanding Image Metadata
Image metadata refers to the auxiliary information embedded in an image file. This information can include details such as the camera model, date and time the image was captured, resolution, GPS coordinates, and much more. Extracting image metadata is essential for various applications, such as digital asset management, image categorization, and content-based image retrieval.
Working with Docker and Spring Boot
Docker provides a platform for packaging, distributing, and running applications within containers. Spring Boot, on the other hand, offers a convenient way to create stand-alone, production-grade Spring-based applications. Combining Docker with Spring Boot allows for the seamless deployment of applications in a containerized environment.
To integrate Docker with Spring Boot, ensure that you have the necessary dependencies configured in your pom.xml
file. Utilize the Maven spring-boot-starter-parent
and docker-maven-plugin
to enable smooth integration between the Spring Boot application and Docker.
<dependencies>
<!-- Other dependencies -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.3</version> <!-- Use the appropriate version -->
</dependency>
<!-- Other dependencies -->
</dependencies>
<build>
<plugins>
<!-- Other plugins -->
<plugin>
<groupId>com.spotify</groupId>
<artifactId>docker-maven-plugin</artifactId>
<configuration>
<!-- Docker configuration -->
</configuration>
</plugin>
<!-- Other plugins -->
</plugins>
</build>
Once the dependencies are set up, Docker commands and configurations can be embedded in Maven build phases, allowing for smooth Docker image creation, container deployment, and integration with the Spring Boot application.
Extracting Image Metadata with Java
Java provides several libraries for extracting image metadata. One popular library is metadata-extractor, which supports various image formats such as JPEG, TIFF, WebP, and even video formats like MP4. This library offers comprehensive support for extracting a wide range of metadata from images, including Exif, IPTC, XMP, and ICC profile data.
To incorporate the metadata-extractor library into your Spring Boot application, simply include it as a dependency in your pom.xml
file.
<dependency>
<groupId>com.drewnoakes</groupId>
<artifactId>metadata-extractor</artifactId>
<version>2.16.0</version> <!-- Use the latest version -->
</dependency>
Using the metadata-extractor library, you can extract image metadata with just a few lines of code. Below is an example of how to extract metadata from an image file in Java:
import com.drew.imaging.ImageMetadataReader;
import com.drew.metadata.Metadata;
import com.drew.metadata.Directory;
import com.drew.metadata.exif.ExifIFD0Directory;
import java.io.File;
public class ImageMetadataExtractor {
public void extractMetadata(String imagePath) {
try {
File file = new File(imagePath);
Metadata metadata = ImageMetadataReader.readMetadata(file);
for (Directory directory : metadata.getDirectories()) {
for (com.drew.metadata.Tag tag : directory.getTags()) {
System.out.println(tag);
}
}
// Accessing specific tag (e.g., camera model)
if (metadata.containsDirectory(ExifIFD0Directory.class)) {
ExifIFD0Directory exifDirectory = metadata.getFirstDirectoryOfType(ExifIFD0Directory.class);
System.out.println("Camera Model: " + exifDirectory.getDescription(ExifIFD0Directory.TAG_MODEL));
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
ImageMetadataExtractor extractor = new ImageMetadataExtractor();
extractor.extractMetadata("path_to_your_image_file.jpg");
}
}
In this example, we use the metadata-extractor library to read the metadata from the specified image file. The extracted metadata is then iterated through, and specific pieces of information, such as the camera model, are accessed and printed.
In Conclusion, Here is What Matters
In conclusion, working with image metadata in a Dockerized Spring Boot application is made easy with Java's robust libraries and the seamless integration of Docker and Spring Boot. Extracting image metadata using the metadata-extractor library allows for efficient processing and utilization of image-related information within your applications.
Understanding the significance of image metadata and how to effectively extract it using Java empowers applications with the ability to manage, categorize, and retrieve images based on their embedded information.
By leveraging the powerful combination of Docker, Spring Boot, and Java's libraries, you can enhance your applications with image metadata extraction capabilities, opening up a world of possibilities for image-driven functionalities.
For further exploration and integration of image metadata extraction in your Java applications, dive into the metadata-extractor documentation and take advantage of the extensive features and support it offers for working with image metadata.
Incorporating image metadata extraction into your Dockerized Spring Boot applications opens up new avenues for image management and retrieval, allowing you to harness the valuable information embedded within images for various use cases.