Streamlining Tests: Merge AssertJ & Awaitility with Java 8
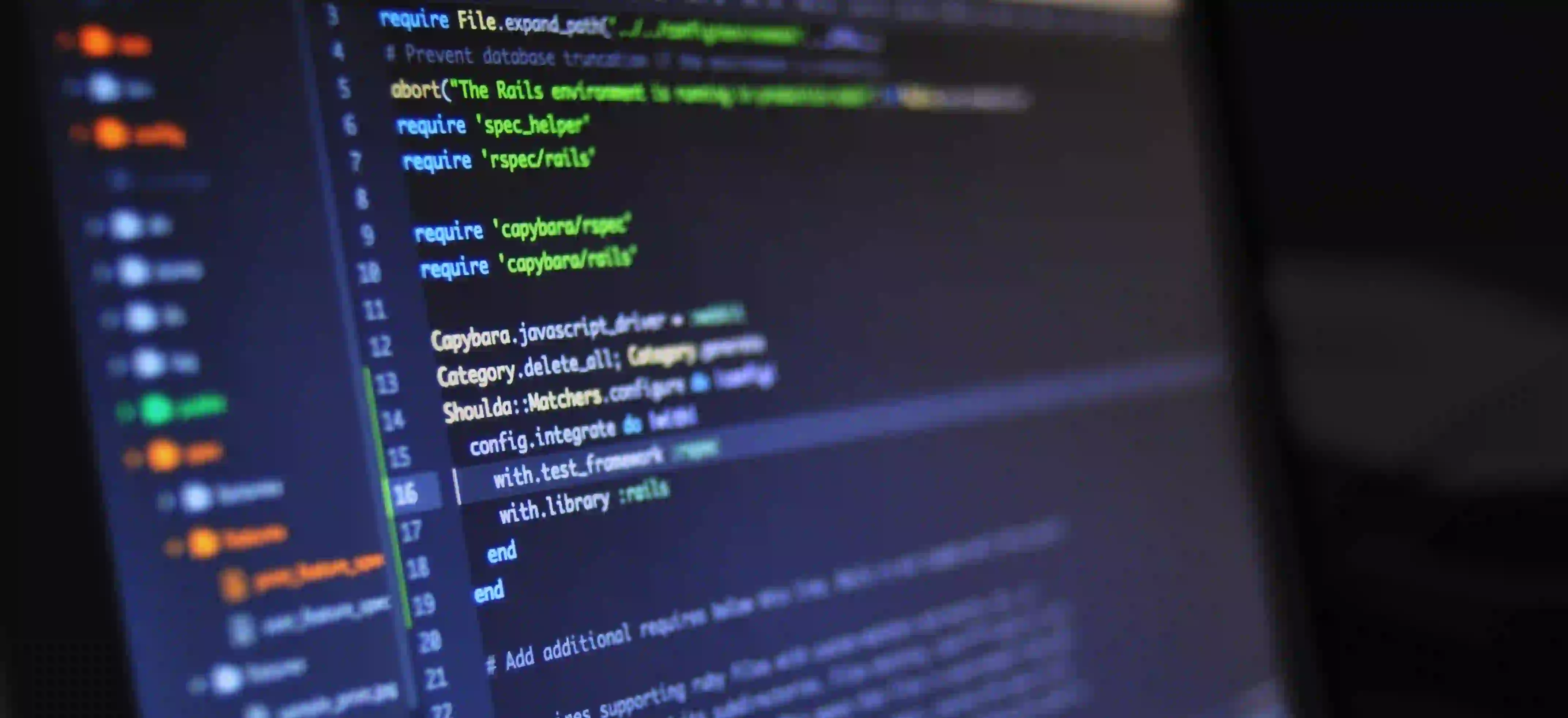
Streamlining Tests: Merge AssertJ & Awaitility with Java 8
In the world of Java development, writing robust and reliable tests is crucial for ensuring the quality and stability of your applications. Test automation frameworks such as AssertJ and Awaitility play a vital role in this process by simplifying the creation and maintenance of test suites.
AssertJ: Fluent Assertions for Java
AssertJ is a popular assertion library that provides a fluent API for performing assertions in Java tests. With its expressive syntax and vast array of built-in assertions, AssertJ allows developers to write highly readable and maintainable tests.
Consider the following example, where we use AssertJ to assert the equality of two objects:
import static org.assertj.core.api.Assertions.*;
public class UserTest {
@Test
public void testUserEquality() {
User user1 = new User("John");
User user2 = new User("John");
assertThat(user1).isEqualTo(user2);
}
}
In this test, the assertThat
method provides a clear and concise way to verify that user1
is equal to user2
, making the test case easily understandable for anyone reading the code.
Awaitility: Fluent API for Asynchronous Testing
On the other hand, asynchronous testing can be challenging, especially when dealing with time-bound operations such as waiting for a certain condition to be met. This is where Awaitility comes into play, offering a fluent API for creating asynchronous assertions in Java tests.
Let's consider a scenario where we want to test if a message is eventually published to a message queue:
import static org.awaitility.Awaitility.*;
public class MessageQueueTest {
@Test
public void testMessagePublish() {
// Publish a message asynchronously
publishMessage("Hello, World!");
// Use Awaitility to wait for the message to be published
await().atMost(5, SECONDS).until(() -> messageQueue.size() == 1);
}
}
In this test, we use Awaitility's await().atMost().until()
construct to wait for the condition (i.e., the message queue size reaches 1) to be satisfied within a specified time frame. This eliminates the need for cumbersome polling mechanisms and thread sleeps in our test code.
Integrating AssertJ with Awaitility using Java 8
While AssertJ and Awaitility both excel in their respective domains, integrating the two libraries can bring significant benefits to our test suites. Combining the fluent assertions of AssertJ with the asynchronous capabilities of Awaitility can result in more cohesive and powerful tests.
Simplifying Test Cases with Merged Assertions
By leveraging Java 8's lambda expressions and method references, we can seamlessly merge AssertJ assertions with Awaitility conditions, leading to more concise and readable test cases. Let's see how we can achieve this integration.
Consider a scenario where we want to test an asynchronous operation while asserting its result using AssertJ. Here's how we can accomplish this using the merged approach:
import static org.assertj.core.api.Assertions.*;
import static org.awaitility.Awaitility.*;
public class BackgroundJobTest {
@Test
public void testBackgroundJobCompletion() {
// Initiate an asynchronous background job
BackgroundJob job = new BackgroundJob();
job.start();
// Use merged assertions with Awaitility
await().atMost(10, SECONDS).until(() -> job.isCompleted());
assertThat(job.getResult()).isEqualTo("SUCCESS");
}
}
In this example, we combine the expressive assertions of AssertJ with the asynchronous condition provided by Awaitility to create a cohesive test case. The await().atMost().until()
construct waits for the background job to complete, while the subsequent assertThat
statement verifies its result. The resulting test is more readable and comprehensible compared to using traditional assertion and polling techniques separately.
Handling Exceptions and Timeouts with Merged Approach
In addition to simplifying test cases, merging AssertJ with Awaitility allows for seamless handling of exceptions and timeouts in asynchronous operations. Consider a scenario where we need to verify the presence of a particular element in an asynchronously updated collection:
import static org.assertj.core.api.Assertions.*;
import static org.awaitility.Awaitility.*;
public class AsyncCollectionTest {
@Test
public void testAsyncCollectionUpdate() {
// Update the collection asynchronously
updateCollectionAsynchronously();
// Use merged assertions with Awaitility to handle exceptions and timeouts
await().atMost(5, SECONDS)
.untilAsserted(() -> assertThat(collection).contains("updatedElement"));
}
}
In this example, the untilAsserted
method from Awaitility ensures that the assertion provided by AssertJ is periodically re-evaluated until it passes, effectively handling any exceptions that may occur during the asynchronous update. Additionally, the specified timeout within atMost
guards against the test getting stuck indefinitely, providing a safety net for the test execution.
Final Considerations
Incorporating AssertJ's fluent assertions with Awaitility's asynchronous testing capabilities using Java 8's functional features can greatly enhance the clarity and effectiveness of your test suites. The merged approach not only simplifies the creation of test cases but also enables seamless handling of exceptions and timeouts, resulting in more robust and reliable tests.
By unifying the strengths of AssertJ and Awaitility, developers can streamline their testing processes and build resilient test suites that effectively validate the functionality of their applications in various scenarios.
So, why not give this merged approach a try in your next testing endeavor? Your future self, and your teammates, will thank you for the readability and reliability of the tests you write.