Overcoming Spring & jOOQ Code Generation Hurdles
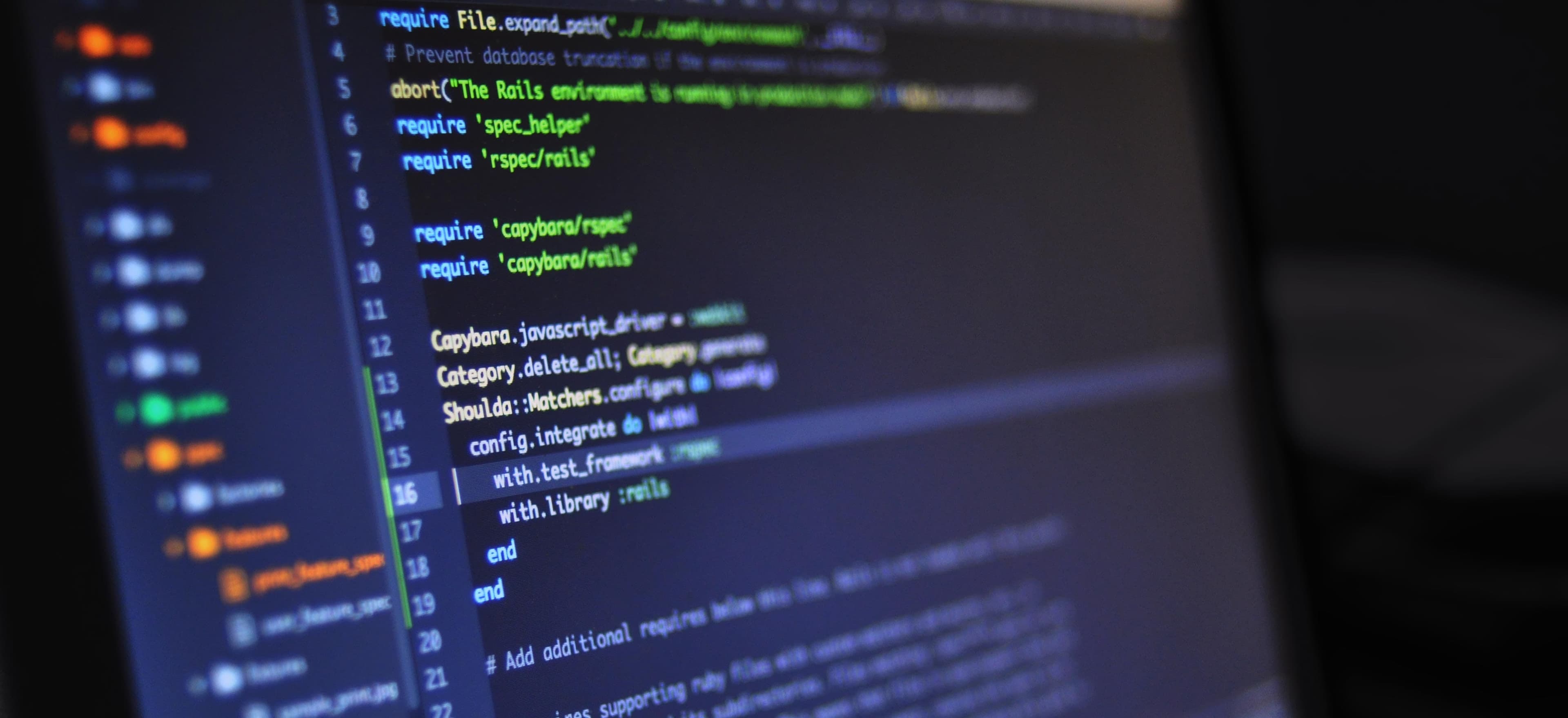
- Published on
Overcoming Spring & jOOQ Code Generation Hurdles
As a Java developer, you might have found yourself in a situation where you needed to work with a large database schema and wanted a smooth transition between database tables and Java objects. This is where tools like Spring and jOOQ come into play. Spring provides a robust framework for building Java applications, while jOOQ facilitates type-safe SQL query construction and execution for relational databases.
In this blog post, we will explore the common hurdles faced during the integration of Spring and jOOQ, specifically focusing on code generation, and discuss effective strategies to overcome them.
Understanding Code Generation in jOOQ
jOOQ takes a unique approach to interacting with databases by generating Java classes from a database schema. These generated classes provide a type-safe environment for writing database queries in Java, as opposed to using traditional string-based SQL queries.
Before diving into the hurdles and their solutions, let's take a brief look at the process of code generation in jOOQ.
Code Generation Configuration
To start generating code with jOOQ, you need to create a code generation configuration XML or use the programmatic API to configure the generation process. This configuration includes details about the database connection, target package for generated classes, and other customization options.
<configuration xmlns="http://www.jooq.org/xsd/jooq-codegen-3.14.4.xsd">
<jdbc>
<driver>com.mysql.cj.jdbc.Driver</driver>
<url>jdbc:mysql://localhost:3306/your_database</url>
<user>username</user>
<password>password</password>
</jdbc>
<generator>
<database>
<name>org.jooq.meta.mysql.MySQLDatabase</name>
<inputSchema>your_schema</inputSchema>
</database>
<target>
<packageName>com.yourapp.generated</packageName>
<directory>src/main/java</directory>
</target>
</generator>
</configuration>
The configuration specifies the database details, the target package, and the directory where the generated classes will reside.
Execution of Code Generation
Once the configuration is set up, you can execute the code generation process. This will connect to the specified database, introspect the schema, and generate the corresponding Java classes representing tables, records, and other database objects.
Common Hurdles and Their Solutions
Hurdle 1: Integration with Spring Boot
Integrating jOOQ code generation with a Spring Boot project can be challenging. You may encounter issues related to the correct placement of the generated code, resolving dependencies, and ensuring that the generated classes are available during runtime.
Solution:
One effective approach to tackle this hurdle is to use the Maven or Gradle build system to automatically run the code generation process during the build phase. By doing this, the generated classes will be available on the classpath when the application starts.
You can use the maven-jooq-codegen-plugin
in Maven or the org.jooq:jooq-codegen
Gradle plugin to configure and trigger the code generation process as part of the build lifecycle.
Hurdle 2: Customizing Code Generation
While jOOQ provides default code generation configurations, you may need to customize the generated code to align with specific project requirements. This could involve modifying the naming conventions, excluding certain tables or columns, or applying custom annotations to the generated classes.
Solution:
jOOQ allows you to extend the code generation process by providing custom code generation strategies and templates. By creating custom generator strategies, you can override the default behavior of the code generation process and tailor it to your needs. Additionally, using templates enables you to control the generated code's structure and content, allowing for extensive customization.
Hurdle 3: Handling Changes in the Database Schema
As the database schema evolves over time, keeping the generated code in sync with the changes becomes crucial. Manually updating the generated code to reflect these changes can be error-prone and time-consuming.
Solution:
To address this hurdle, jOOQ offers the org.jooq.codegen.ConfigurableGenerator
interface, which allows you to react to database schema changes and customize the code generation process accordingly. By implementing this interface, you can programmatically handle schema changes and ensure that the generated code remains aligned with the database schema.
In addition, utilizing version control systems and establishing a well-defined process for updating the code generation configuration can streamline the management of schema changes.
In Conclusion, Here is What Matters
In this blog post, we delved into the common hurdles associated with Spring and jOOQ code generation, as well as effective solutions to overcome them. By understanding the intricacies of code generation in jOOQ and leveraging its customization capabilities, you can seamlessly integrate database schema and Java objects in your Spring applications.
Remember, embracing code generation with jOOQ not only simplifies database interactions but also fosters maintainability and type safety in your Java projects.
With the insights gained from this discussion, you are now better equipped to tackle code generation challenges and harness the full potential of jOOQ in your Spring applications.
For more in-depth information about jOOQ code generation, you can refer to the official jOOQ documentation.
Happy coding!
Checkout our other articles