Overcoming Lazy Assignment Pitfalls in Java: Best Practices
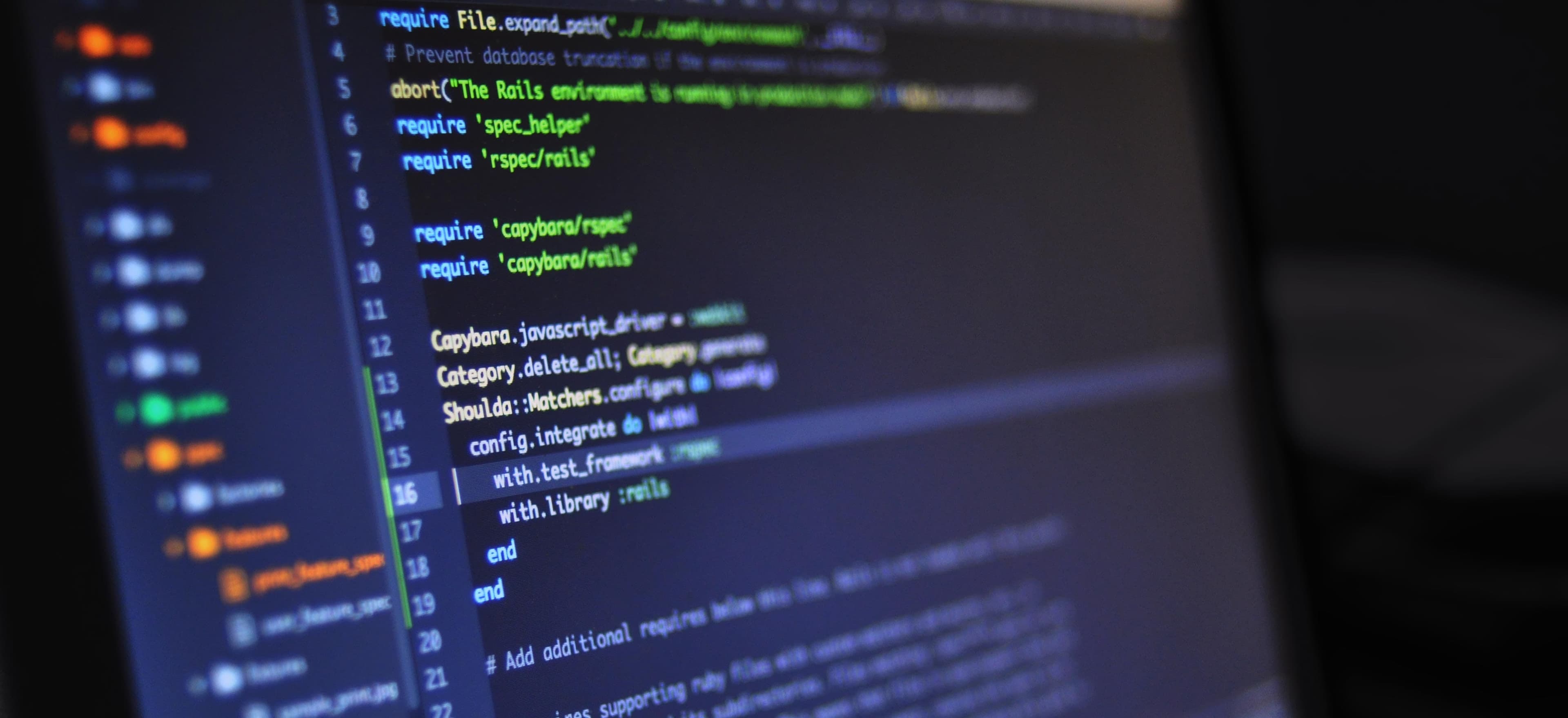
- Published on
Overcoming Lazy Assignment Pitfalls in Java: Best Practices
Let us Begin
In the world of Java programming, lazy evaluation and assignment are crucial concepts used to enhance performance and memory efficiency. However, embracing lazy assignment can also lead to several pitfalls if not implemented diligently. This article aims to explore the common pitfalls associated with lazy assignment in Java and provide practical strategies and best practices to avoid these pitfalls effectively.
What is Lazy Assignment in Java?
Definition and Explanation
Lazy assignment, also known as lazy initialization, is a design pattern where the initialization of an object is deferred until it is required. This means that the object is only initialized when it is first accessed, rather than being instantiated immediately. Lazy assignment is often used to improve the performance of an application by postponing the resource-intensive initialization of objects until they are actually needed.
Why Use Lazy Assignment
The primary benefit of lazy assignment is improved performance and reduced memory usage. By delaying the initialization of objects until they are actually needed, unnecessary resource consumption is avoided, leading to more efficient memory utilization. This becomes particularly significant in scenarios where the creation of an object is expensive in terms of time and resources.
Examples
public class LazyInitializationExample {
private ExpensiveObject expensiveObject;
public ExpensiveObject getExpensiveObject() {
if (expensiveObject == null) {
expensiveObject = new ExpensiveObject();
}
return expensiveObject;
}
}
In this example, the ExpensiveObject
is only initialized when the getExpensiveObject()
method is called for the first time. Subsequent calls to the method will return the previously initialized object.
Common Pitfalls of Lazy Assignment
Lazy assignment, while beneficial, comes with its own set of pitfalls that developers need to be mindful of.
Overview
- Thread Safety Issues: Lazy initialized objects in a multithreaded environment can lead to race conditions and data inconsistency.
- Increased Complexity: Implementing lazy assignment can introduce additional complexity to the codebase.
- Debugging Difficulties: Identifying issues related to lazy initialization can be challenging, especially when they occur sporadically.
- Unintended Side Effects: Lazy initialization can inadvertently trigger unexpected behavior or side effects in the application.
Real-life Scenarios
Consider a scenario where a lazy-initialized singleton object is accessed concurrently by multiple threads. Without proper synchronization, this can lead to unpredictable behavior and data corruption.
Best Practices to Overcome Lazy Assignment Pitfalls
To address the pitfalls associated with lazy assignment in Java, certain best practices and strategies can be employed. Let's explore some of these best practices in detail.
Ensuring Thread Safety
Overview
Thread safety is a critical concern when it comes to lazy initialization. Without proper synchronization, concurrent access to lazily initialized objects can lead to data corruption and inconsistencies.
Solutions and Code Snippets
Use of volatile
keyword with double-checked locking pattern
public class LazyInitializedSingleton {
private static volatile LazyInitializedSingleton instance;
private LazyInitializedSingleton() {
}
public static LazyInitializedSingleton getInstance() {
if (instance == null) {
synchronized (LazyInitializedSingleton.class) {
if (instance == null) {
instance = new LazyInitializedSingleton();
}
}
}
return instance;
}
}
The use of the volatile
keyword ensures that changes to the instance variable are immediately visible to other threads. This, combined with the double-checked locking pattern, ensures that only one instance of the singleton is created, even in a multithreaded scenario.
Utilize AtomicReference
or ConcurrentHashMap
for thread-safe lazy initialization
public class LazyInitializationWithAtomicReference {
private static final AtomicReference<ExpensiveObject> instance = new AtomicReference<>();
public ExpensiveObject getExpensiveObject() {
ExpensiveObject result = instance.get();
if (result == null) {
result = new ExpensiveObject();
if (instance.compareAndSet(null, result)) {
// Object successfully initialized
} else {
// Another thread initialized the object, use the existing one
result = instance.get();
}
}
return result;
}
}
In this example, the AtomicReference
ensures atomic updates to the reference, enabling safe and efficient lazy initialization in a multithreaded environment.
Why
These solutions effectively address the issues related to thread safety in lazy assignment. They ensure that objects are initialized in a thread-safe manner, preventing race conditions and data inconsistencies.
Simplifying Complexity
When implementing lazy assignment, it's essential to keep the logic simple and maintainable.
Tips
- Use Standard Design Patterns: Leverage well-established design patterns such as Singleton or Factory to encapsulate and manage lazy initialization logic.
- Avoid Nested Initialization Logic: Keep the lazy initialization logic straightforward and free from complex nested constructs, making the code more maintainable.
Enhancing Debuggability
Tracing issues in code that utilizes lazy assignment can be challenging. Employing strategies to improve debuggability is crucial.
Strategies
- Logging: Incorporate logging statements to track the state transitions during lazy initialization, enabling the identification of potential issues.
- Effective Use of Debugging Tools: Make use of debugging tools provided by IDEs to step through the lazy initialization process, allowing for thorough inspection and diagnosis of issues.
Avoiding Unintended Side Effects
Guidelines on how to minimize or avoid side effects of lazy assignment are paramount for producing robust and predictable code.
Guidelines
- Immutable Initialization Data: If possible, initialize objects in a manner that does not alter their state after the initial lazy initialization.
- Unit Testing: Implement comprehensive unit tests to validate the behavior of lazily initialized objects and ensure they do not produce unintended side effects.
Best Practice
public class LazyInitializationTestingExample {
@Test
public void testLazyInitialization() {
ExpensiveObject obj = new LazyInitializationExample().getExpensiveObject();
// Perform assertions to validate the behavior and state of the initialized object
}
}
By writing unit tests that specifically target the lazy initialization process, developers can ensure that unintended side effects are caught and prevented.
Tools and Frameworks to Simplify Lazy Assignment in Java
In addition to adhering to best practices, developers can leverage certain tools and frameworks that simplify the implementation of lazy assignment in Java.
Overview
Some of the popular tools and frameworks that facilitate and streamline lazy assignment in Java include:
- Spring Framework's Lazy Initialization Feature: The Spring Framework provides support for lazy initialization of beans, enabling efficient resource usage in applications.
- Java 8's
Supplier<T>
Interface for Lazy Evaluation: TheSupplier
interface in Java 8 can be utilized to implement lazy evaluation of objects and functions, offering a functional programming approach to lazy initialization.
Why
These tools and frameworks offer pre-defined mechanisms and abstractions that align with best practices, simplifying the implementation of lazy initialization and reducing the likelihood of encountering pitfalls.
A Final Look
In conclusion, while lazy assignment is a powerful technique for optimizing the performance and resource utilization of Java applications, it comes with its own set of challenges. By employing the best practices and strategies discussed in this article, developers can effectively mitigate the pitfalls associated with lazy assignment and ensure the robustness and reliability of their code. It is crucial to approach lazy assignment responsibly and implement it with a thorough understanding of its potential implications, leveraging the available tools and frameworks to streamline the process. By integrating these best practices into their Java projects, developers can confidently harness the benefits of lazy initialization while mitigating its inherent risks.
Call to Action
I encourage you to apply the best practices and strategies discussed in this article to your Java projects, particularly when dealing with lazy assignment and initialization. Embracing these techniques will empower you to build more efficient and resilient Java applications, contributing to enhanced performance and reliability. Additionally, continue to explore and deepen your understanding of effective programming techniques to continually elevate your skills as a Java developer.
Instructions for Writing Code Snippets
When writing code snippets for this article, ensure that they adhere to the following guidelines:
- Clarity and Simplicity: Code snippets should be clear, concise, and accompanied by relevant comments explaining the key workings and the rationale behind them.
- Realistic Examples: Provide examples that are realistic and applicable to real-world scenarios, demonstrating the practical application of the solutions.
- Testing and Validation: Encourage readers to test the code snippets independently to ensure they work as intended and to reinforce their learning through hands-on practice.
Incorporating Links
Incorporate links in the article by following these guidelines:
- Official Documentation: Link to the official documentation of concepts, tools, or frameworks introduced in the article, providing readers with the opportunity to explore further.
- Further Reading: Include links to further reading for advanced topics or related concepts not covered in depth, offering additional resources for readers interested in delving deeper into the subject matter. Ensure that the links are seamlessly integrated, adding value and context to the article without disrupting its flow.