Avoid Common Mistakes with Java's regionMatches Method
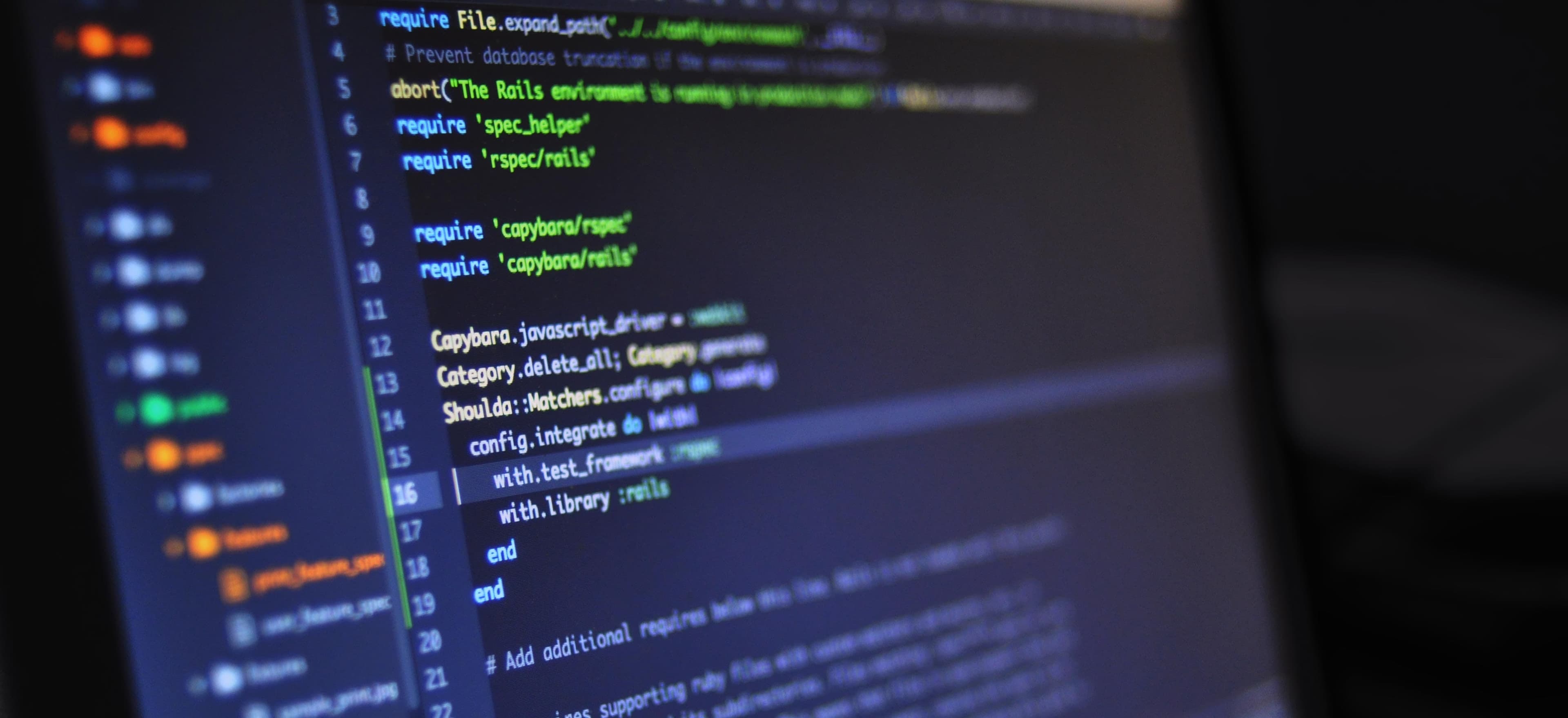
- Published on
Avoid Common Mistakes with Java's regionMatches
Method
When working with string comparisons in Java, the regionMatches
method is a powerful tool for comparing a specific region of one string to another. However, the incorrect usage of this method can lead to unexpected errors and buggy behavior in your applications.
In this article, we'll explore the common mistakes when using the regionMatches
method in Java and how to avoid them. We'll also discuss best practices and provide code examples to clarify its proper usage.
Understanding the regionMatches
Method
The regionMatches
method in Java is used to compare a specific region of one string to a specific region of another string. Its signature is as follows:
public boolean regionMatches(int toffset, String other, int ooffset, int len)
Where:
toffset
is the starting offset in the current string.other
is the other string to compare against.ooffset
is the starting offset in the other string.len
is the length of the region to compare.
The regionMatches
method returns true
if the specified region of the two strings matches, and false
otherwise.
Common Mistakes to Avoid
1. Incorrect Region Length
One common mistake when using the regionMatches
method is providing an incorrect length for the comparison region. This can lead to unexpected results, especially when the length exceeds the actual size of the strings.
String str1 = "Hello, World!";
String str2 = "Hello, Universe!";
boolean matches = str1.regionMatches(0, str2, 0, 15);
In the above example, the length specified for the comparison region in str1
is 15, which exceeds the length of str1
itself. This can lead to IndexOutOfBoundsException or incorrect comparison results.
To avoid this mistake, always ensure that the length parameter for the comparison region does not exceed the actual length of the strings being compared.
2. Case Sensitivity
Another common mistake is failing to consider case sensitivity when using regionMatches
. By default, regionMatches
is case-sensitive, meaning it considers the case of the characters when performing the comparison.
String str1 = "HELLO";
String str2 = "hello";
boolean matches = str1.regionMatches(0, str2, 0, 5); // returns false
In the above example, the comparison returns false
because the case of the characters in str1
and str2
does not match.
If case insensitivity is desired, you can use the regionMatches
overload that accepts a boolean parameter for case insensitivity:
boolean matchesIgnoreCase = str1.regionMatches(true, 0, str2, 0, 5); // returns true
Always consider the case sensitivity requirement and use the appropriate overload of regionMatches
to achieve the desired comparison behavior.
3. Offsets Out of Bounds
Providing incorrect offsets for the comparison regions is another mistake to avoid. When the provided offsets exceed the bounds of the strings, it can lead to IndexOutOfBoundsException or incorrect comparison results.
String str1 = "Hello, World!";
String str2 = "World";
boolean matches = str1.regionMatches(7, str2, 0, 5);
In this example, the offset for str1
is 7, which exceeds its length, causing unexpected results or potential errors.
Always ensure that the provided offsets are within the bounds of the strings being compared to avoid these issues.
Best Practices for Using regionMatches
Now that we've discussed the common mistakes, let's delve into best practices for using the regionMatches
method effectively.
1. Validate Input Parameters
Before calling the regionMatches
method, validate the input parameters such as offsets and lengths to ensure they fall within the bounds of the strings. This validation helps prevent errors and unexpected behavior.
public boolean customRegionMatches(String str1, int toffset, String str2, int ooffset, int len) {
if (toffset < 0 || ooffset < 0 || len < 0 ||
toffset + len > str1.length() || ooffset + len > str2.length()) {
// Handle invalid input parameters
return false;
}
return str1.regionMatches(toffset, str2, ooffset, len);
}
By performing this validation, you can ensure that the regionMatches
method is invoked with valid parameters.
2. Consider Case Sensitivity
Always consider whether case sensitivity is required for your string comparisons. If case insensitivity is needed, utilize the overload of regionMatches
that supports case insensitivity, providing true
as the first argument.
boolean matchesIgnoreCase = str1.regionMatches(true, 0, str2, 0, 5);
By explicitly specifying the case sensitivity requirement, you can avoid unexpected results due to case differences.
3. Document Assumptions and Behavior
When using the regionMatches
method in your code, document the assumptions about the comparison behavior, including the expected case sensitivity, valid offset ranges, and the purpose of the comparison.
/**
* Performs a case-insensitive region match between two strings.
* @param str1 The first string
* @param toffset The starting offset in the first string
* @param str2 The second string
* @param ooffset The starting offset in the second string
* @param len The length of the region to compare
* @return true if the specified region of the two strings matches, ignoring case; false otherwise
*/
public boolean customRegionMatchesIgnoreCase(String str1, int toffset, String str2, int ooffset, int len) {
// Documentation of assumptions about case insensitivity and valid offset ranges
return str1.regionMatches(true, toffset, str2, ooffset, len);
}
By documenting the assumptions and behavior, you provide clarity for future developers who may work with the code.
Closing the Chapter
In conclusion, the regionMatches
method in Java is a powerful tool for comparing specific regions of strings. By understanding its usage, avoiding common mistakes, and following best practices, you can leverage this method effectively in your applications.
Remember to always validate input parameters, consider case sensitivity, and document assumptions and behavior when using regionMatches
. By doing so, you can write robust and reliable string comparison logic in your Java code.
By staying mindful of the potential pitfalls and adhering to best practices, you can harness the full potential of Java's regionMatches
method without falling prey to common mistakes and errors.
References:
- Java String regionMatches Documentation
- String Comparison in Java
Do you have any questions or additional tips for using the regionMatches
method effectively? Share your thoughts in the comments below!