API Gateway vs. Service Mesh: Navigating Your Choice
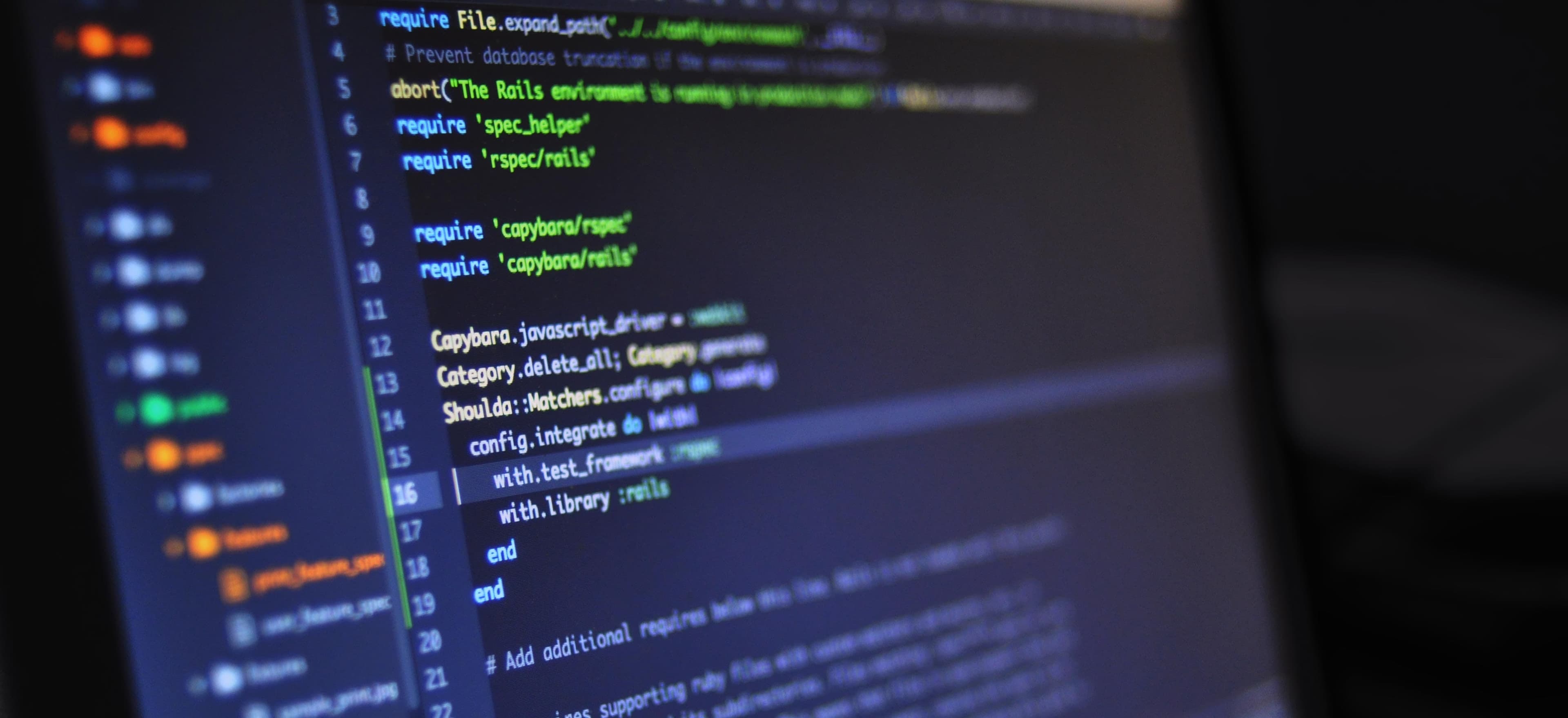
- Published on
API Gateway vs. Service Mesh: Navigating Your Choice
In the world of microservices and distributed systems, managing and securing communication between services is crucial. Two common solutions that address this challenge are API gateways and service meshes. Both offer distinct features and capabilities, and understanding their differences is essential for making an informed decision regarding which one to employ in your architecture.
Overview of API Gateway
An API gateway serves as a single entry point for client requests, functioning as a reverse proxy that directs incoming traffic to the appropriate services. It provides a consolidated interface for accessing various microservices, shielding clients from the intricacies of the underlying architecture. Additionally, API gateways often incorporate functionalities such as request routing, authentication, rate limiting, and caching, contributing to improved performance and security.
Advantages of Using an API Gateway
-
Centralized Management: API gateways enable centralized management of cross-cutting concerns such as authentication, authorization, and traffic control, freeing individual services from having to implement these functionalities independently.
-
Increased Security: By serving as a security enforcement point, API gateways facilitate the implementation of authentication, authorization, and SSL termination, fortifying the perimeter of the system against external threats.
-
Improved Performance: Caching, request/response compression, and load balancing implemented by an API gateway can significantly enhance the overall performance of the system, leading to reduced response times and lower latencies.
Example of Implementing an API Gateway
public class ApiGatewayFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) {
// Perform authentication and authorization checks
// Perform request routing based on the incoming request
// Apply rate limiting and other traffic control policies
chain.doFilter(request, response);
// Apply response transformation and caching before sending the response back to the client
}
}
In this example, the ApiGatewayFilter
intercepts incoming requests, applying various processing steps before forwarding the requests to the respective services.
Overview of Service Mesh
Conversely, a service mesh is concerned with the internal communication between microservices within a network. It consists of a dedicated infrastructure layer that facilitates service-to-service communication, incorporating functionalities such as service discovery, load balancing, encryption, and observability. Service meshes are typically implemented as sidecar proxies, meaning that each service is paired with a proxy that manages its inbound and outbound traffic.
Advantages of Using a Service Mesh
-
Uniform Connectivity: Service meshes ensure consistent connectivity across services, abstracting the complexities of network communication and standardizing features such as load balancing and encryption.
-
Enhanced Observability: By capturing metrics, logs, and tracing data at the network level, service meshes offer comprehensive insights into the interactions between services, facilitating troubleshooting and performance optimization.
-
Dynamic Routing and Resilience: Service meshes enable dynamic routing, fault injection, and circuit breaking, empowering the seamless management of traffic and the enforcement of resilience strategies.
Example of Implementing a Service Mesh
public class ServiceProxy {
public void handleInboundRequest(Request request) {
// Perform service discovery to determine the destination service
// Apply load balancing and encryption before forwarding the request
// Capture metrics and tracing data for observability purposes
}
public void handleOutboundResponse(Response response) {
// Apply response transformation before sending the response back to the calling service
}
}
In this example, the ServiceProxy
acts as a sidecar proxy, intercepting both inbound requests and outbound responses to apply various functionalities, such as load balancing and observability.
Making the Choice
When contemplating whether to employ an API gateway or a service mesh, it is essential to contemplate the specific needs of your architecture and the problems you aim to resolve.
Choose an API Gateway When:
- You require a centralized entry point for external client communication.
- Cross-cutting concerns such as authentication, rate limiting, and caching need to be managed uniformly across services.
- Your focus is on securing and optimizing external API interactions.
Choose a Service Mesh When:
- Internal service communication within the network demands standardized capabilities such as load balancing, service discovery, and encryption.
- Detailed observability and control over internal traffic are critical for maintaining system reliability and performance.
- Dynamic routing and resilience mechanisms need to be enforced at the service level.
In Summary
API gateways and service meshes address distinct aspects of microservices architecture, with the former concentrating on external client communication and the latter centering on internal service-to-service communication. By comprehending their individual strengths and considering the unique requirements of your system, you can make an informed decision on whether to leverage an API gateway, a service mesh, or a combination of both in your microservices environment.
Ultimately, the choice should align with the specific challenges and objectives of your architecture, guiding you towards a solution that optimally manages communication, security, and observability within your distributed system.
For further insights into API gateways and service meshes, consider referring to the following resources:
With a clear understanding of the distinctions between API gateways and service meshes, you are now equipped to navigate the complexities of microservices communication, making an informed choice that aligns with the needs of your architecture.
Checkout our other articles