Boost Your Test Automation with Design for Testability!
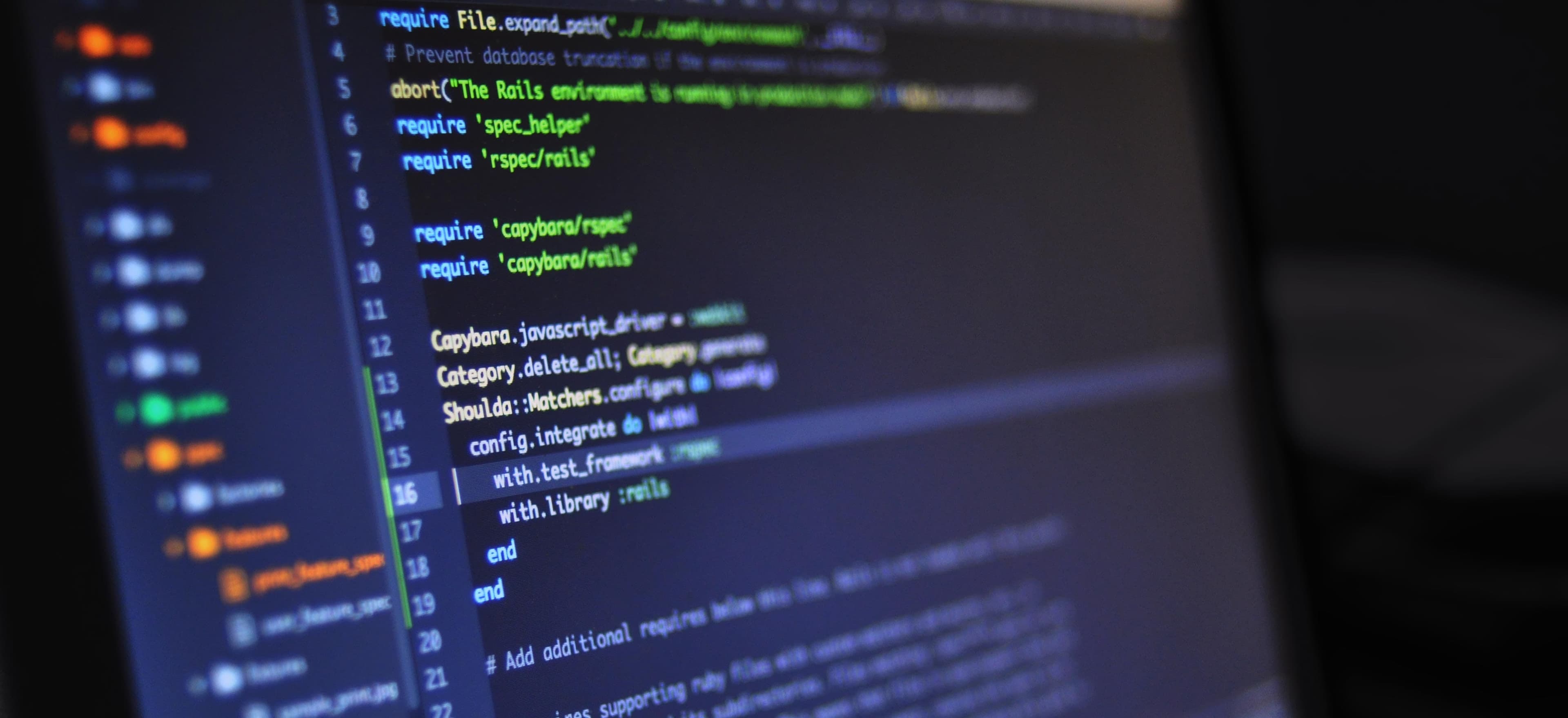
- Published on
Boost Your Test Automation with Design for Testability!
In the world of software development, automated testing plays a crucial role in ensuring the quality and reliability of applications. When it comes to Java, leveraging design for testability can significantly enhance the effectiveness of automated testing processes. In this article, we'll delve into the concept of design for testability, explore how it can elevate your test automation efforts, and provide practical examples to illustrate its impact.
Understanding Design for Testability
Design for testability is a software development principle that emphasizes the importance of creating code that is inherently easier to test. By incorporating design patterns, coding practices, and architectural strategies that facilitate testing, developers can streamline the creation and maintenance of automated test suites.
When it comes to Java, several principles and techniques contribute to enhancing testability, including dependency injection, interface segregation, and adherence to SOLID principles. By adhering to these best practices, developers can write code that is more modular, decoupled, and ultimately, easier to test.
Benefits of Design for Testability in Java
Embracing design for testability in Java brings about a myriad of benefits, including:
- Improved Code Quality: Writing testable code often leads to higher quality code overall, as it encourages modularization, reduced coupling, and adherence to best practices.
- Enhanced Maintainability: Testable code is generally easier to maintain and refactor, promoting long-term sustainability and agility in the software development lifecycle.
- Faster Feedback Loops: Testable code allows for rapid feedback through automated testing, enabling developers to catch and address issues early in the development process.
- Facilitated Collaboration: Testable code fosters collaboration between developers and QA professionals, as it provides a solid foundation for creating and maintaining automated test suites.
Practical Examples of Design for Testability in Java
Let's dive into some practical examples to demonstrate the impact of design for testability in Java.
Example 1: Dependency Injection
public class PaymentService {
private final PaymentGateway paymentGateway;
public PaymentService(PaymentGateway paymentGateway) {
this.paymentGateway = paymentGateway;
}
public boolean processPayment(double amount) {
// Business logic for payment processing using paymentGateway
}
}
In this example, the PaymentService
class utilizes constructor injection to receive an instance of PaymentGateway
. This approach adheres to the principle of inversion of control, making the PaymentService
more testable by allowing us to easily inject a mock or stub PaymentGateway
for testing purposes.
Example 2: Interface Segregation
public interface UserRepository {
User findById(Long id);
void save(User user);
void delete(User user);
}
public class UserRepositoryImpl implements UserRepository {
// Implementation of interface methods
}
By adhering to the interface segregation principle, we can define cohesive and granular interfaces that are tailored to specific client requirements. This allows for better testability by enabling the use of mock implementations for testing different scenarios without the need for complex setup or mocking of unnecessary methods.
Example 3: Unit Testing with JUnit
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class StringUtilsTest {
@Test
public void testReverseString() {
String original = "hello";
String reversed = StringUtils.reverseString(original);
assertEquals("olleh", reversed);
}
}
In this example, we have a simple unit test using JUnit to test the StringUtils
class's reverseString
method. By writing concise and focused unit tests like this, we ensure that our code is both testable and tested effectively, allowing us to catch regressions and bugs early on.
Best Practices for Designing Testable Java Code
To effectively harness the power of design for testability in Java, consider the following best practices:
-
Adhere to SOLID Principles: Embrace principles such as Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion to craft code that is inherently testable and maintainable.
-
Utilize Dependency Injection: Leverage frameworks like Spring or Google Guice to promote loose coupling and facilitate easier testing through dependency injection.
-
Write Focused Unit Tests: Write unit tests that are focused, concise, and address specific behaviors of the code. Avoid overly complex or sprawling tests that make maintenance and debugging challenging.
-
Mock Collaborators Effectively: Use mocking frameworks like Mockito to create mock objects for collaborating classes or interfaces, allowing you to isolate the code under test and simulate various scenarios.
-
Refactor for Testability: Refactor existing code to adhere to testable design patterns, making it easier to introduce automated testing without introducing unnecessary complexity.
Final Thoughts
Design for testability is a pivotal factor in the success of test automation efforts, and Java provides a robust foundation for creating testable code. By embracing principles, patterns, and practices that prioritize testability, developers can unlock the full potential of automated testing and ensure the delivery of high-quality, dependable software solutions.
Incorporating testability into the development process isn't just a technical decision; it's a strategic investment in the long-term success of your software projects. As you continue to refine your skills in Java development and test automation, always keep the principles of design for testability top of mind, and reap the rewards of robust, maintainable, and thoroughly tested code.
Remember, the journey to testability begins with a single step – and the results are well worth the effort!
Want to delve deeper into the world of Java and test automation? Check out this insightful guide on Java testing frameworks to expand your knowledge and elevate your skills!
Checkout our other articles