Code in Peace: Tackling Programmer Distractions Head-On
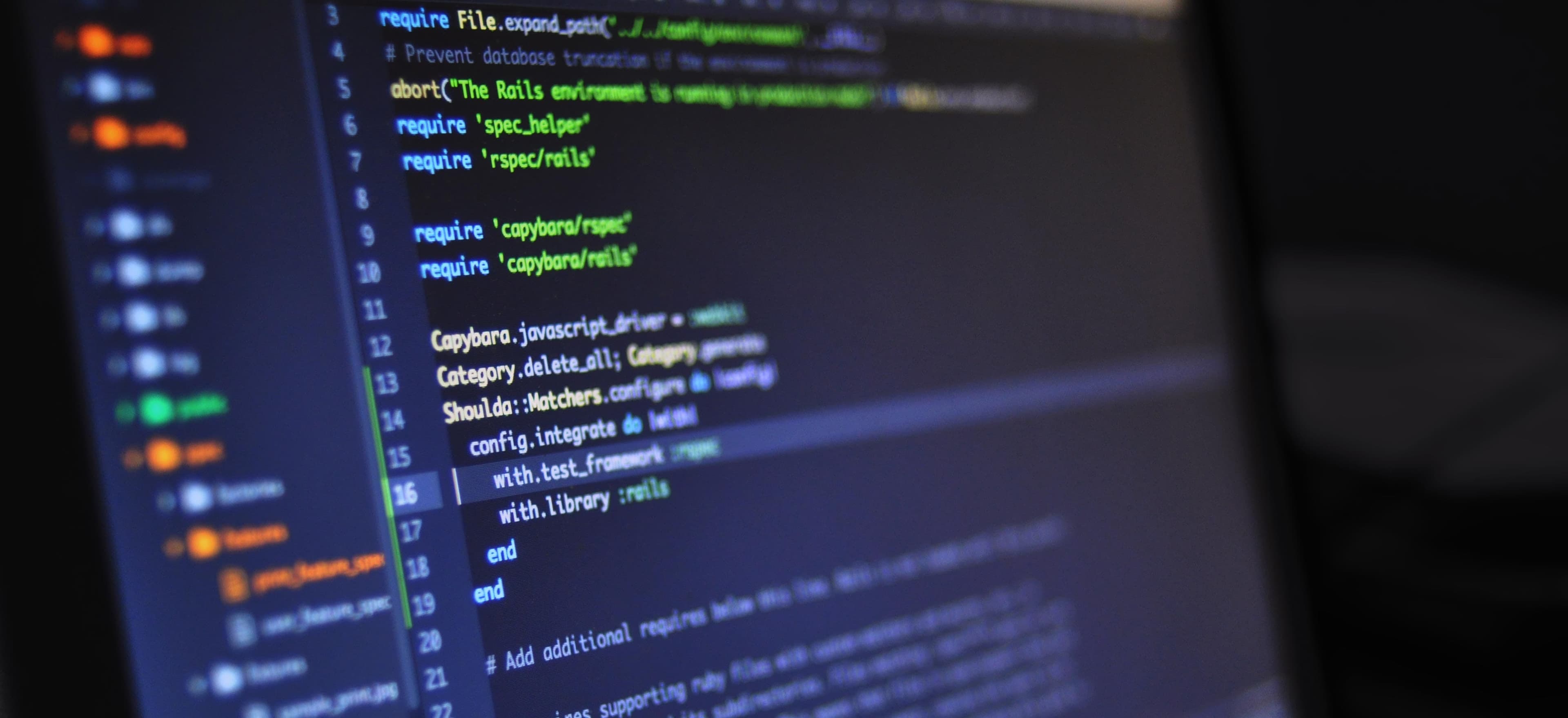
- Published on
Code in Peace: Tackling Programmer Distractions Head-On
In the fast-paced world of software development, distractions are the arch-nemesis of productivity. As a Java developer, maintaining a laser focus is crucial for writing clean, efficient, and error-free code. In this blog post, we'll explore strategies and tools to help Java programmers combat distractions and maximize their coding productivity.
The Battle Against Distractions
Distractions come in many forms – from the incessant ping of notifications to the allure of browsing the web. They disrupt the flow of thoughts and hinder the deep concentration required for effective coding. So, how can Java developers reclaim their focus and achieve a state of "code in peace"?
Creating a Distraction-Free Environment
One of the first steps in combating distractions is to create an environment conducive to deep work. This could mean finding a quiet space to work, using noise-cancelling headphones, or establishing clear boundaries with colleagues to minimize interruptions.
Additionally, tools like Focus@Will can provide specially curated music tracks engineered to enhance concentration, effectively blocking out ambient noise and promoting a state of flow.
Leveraging IDE Features
Most modern Integrated Development Environments (IDEs) offer features designed to minimize distractions and streamline the coding process. For Java developers, IntelliJ IDEA's distraction-free mode provides a clean, minimalistic interface, allowing the focus to remain solely on the code.
// Enable distraction-free mode in IntelliJ IDEA
// Navigate to View -> Appearance -> Enter Distraction Free Mode
The distraction-free mode declutters the interface, hiding all panels and tool windows, ensuring that the developer's attention remains solely on the code being written.
Employing Productivity Tools
A wealth of productivity tools is available to assist developers in managing distractions. For instance, tools like Toggl can help track time spent on specific tasks, offering insights into productivity patterns and identifying potential distractions.
Moreover, the Pomodoro Technique, facilitated by apps like Pomodone, advocates for working in focused, 25-minute intervals with brief breaks in between. This method encourages sustained concentration while mitigating the impact of distractions.
Writing Clean and Efficient Java Code
Once the distractions have been minimized, the focus can shift to the art of writing clean and efficient Java code. Here are some strategies to elevate your coding prowess:
Embracing Java 8 Features
Java 8 introduced a plethora of features aimed at enhancing code readability and conciseness. Leveraging lambdas, streams, and functional interfaces can significantly streamline code, making it easier to read, understand, and maintain.
// Example of using lambda expressions in Java 8
List<String> programmingLanguages = Arrays.asList("Java", "Python", "JavaScript", "C#");
programmingLanguages.forEach(language -> System.out.println(language));
The use of lambda expressions in Java 8 simplifies iteration and can eliminate verbose boilerplate code, leading to a more elegant and concise codebase.
Utilizing Design Patterns
Design patterns are essential tools in a Java developer's arsenal for writing robust and maintainable code. Understanding and applying patterns such as Singleton, Factory, and Observer can significantly improve the structure and extensibility of your codebase.
// Example of Singleton design pattern in Java
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. Employing such patterns enhances code clarity and promotes reusability.
Final Considerations
In the tumult of modern software development, it's essential for Java programmers to proactively combat distractions and enhance their coding efficiency. By creating a distraction-free environment, leveraging IDE features, and employing productivity tools, developers can reclaim their focus and immerse themselves in the art of writing clean and efficient Java code.
Remember, the journey to "code in peace" is a continual process, requiring mindfulness and a commitment to honing one's craft. Embracing modern language features and design patterns further elevates the coding experience, ensuring that every line of Java code is a testament to precision and elegance.
In the end, the pursuit of a distraction-free coding environment and the mastery of clean and efficient Java code intertwine, leading to a heightened state of productivity and artistic expression in the realm of software development.
So, let's embark on this journey together, armed with the tools and strategies to code in peace and deliver exceptional Java solutions.
Join the battle, thrive amidst the code, and let your Java creations flourish in an environment of undisturbed focus and unwavering productivity!
Checkout our other articles