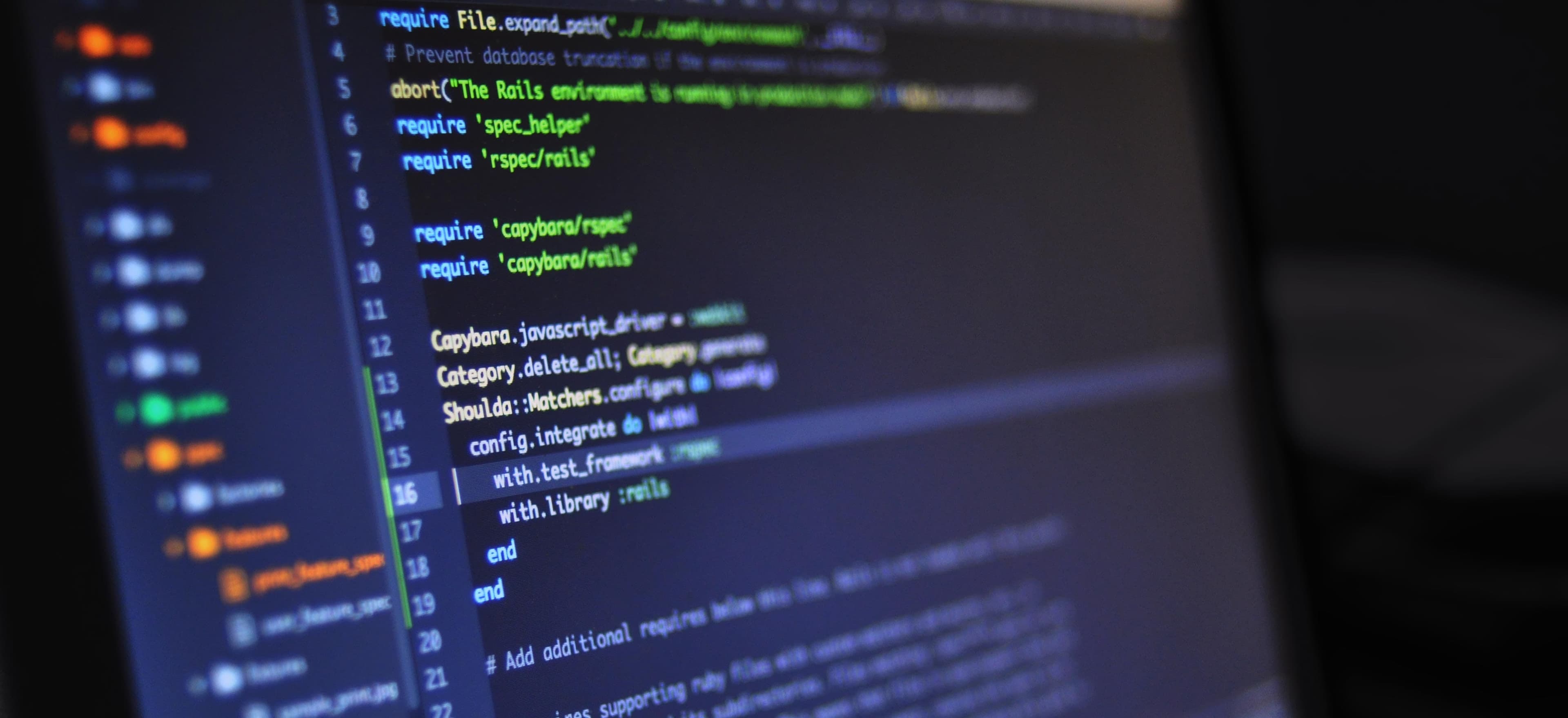
- Published on
Spring Data REST Magic: Easy Exporting of JPA Repositories!
Do you want to expose your JPA repositories as RESTful resources with minimal effort? Look no further than Spring Data REST! In this blog post, we'll explore how Spring Data REST can work its magic to effortlessly expose JPA repositories as RESTful APIs.
What is Spring Data REST?
Spring Data REST is a part of the larger Spring Data project that aims to make it easier to build Spring-powered applications that use new data access technologies such as NoSQL databases and cloud-based data services. Spring Data REST builds on top of Spring Data repositories and automatically exports them as hypermedia-driven REST resources.
By combining the power of Spring Data repositories and Spring MVC, Spring Data REST exposes your JPA repositories as RESTful APIs without the need for manual controller configuration.
Getting Started
To get started with Spring Data REST, you'll need a Spring Boot application with Spring Data JPA on the classpath. If you don't have a project set up yet, you can use Spring Initializr to create a new Spring Boot project with the required dependencies.
Exposing an Entity as a REST Resource
Let's say you have a JPA entity Product
:
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
// Getters and setters
}
To expose the Product
entity as a REST resource, all you need to do is create a Spring Data JPA repository interface for it:
public interface ProductRepository extends JpaRepository<Product, Long> {
}
With just these two pieces in place, Spring Data REST will automatically expose the Product
entity as a REST resource at the /products
endpoint.
Customizing Endpoints
Spring Data REST uses sensible defaults for exposing repositories, but you can customize the REST endpoints by using @RepositoryRestResource
annotation:
@RepositoryRestResource(path = "items")
public interface ProductRepository extends JpaRepository<Product, Long> {
}
By adding @RepositoryRestResource
with the path
attribute, you can change the base path from /products
to /items
.
HATEOAS and Content Negotiation
One of the great things about Spring Data REST is that it provides HATEOAS (Hypermedia as the Engine of Application State) out of the box. This means that the REST resources it exposes include links to related resources, making it easier to navigate the API.
In addition, Spring Data REST supports content negotiation, allowing clients to request resources in different formats (e.g., JSON, XML) by setting the Accept
header in the HTTP request.
Pagination and Sorting
Another benefit of using Spring Data REST is the built-in support for pagination and sorting. By default, the REST resources are paginated, and clients can control the page size, number, and sorting parameters through query parameters.
Security and Custom Endpoints
While exposing JPA repositories as REST resources can be convenient, it's essential to consider security implications. You may need to secure the exposed endpoints with authentication and authorization mechanisms. Spring Security can be seamlessly integrated with Spring Data REST to enforce security requirements.
For cases where you need custom behavior beyond what Spring Data REST provides out of the box, you can create custom controller endpoints and handle the logic manually. This allows you to strike a balance between leveraging the automatic REST exporting and implementing specific business requirements.
In Conclusion, Here is What Matters
Spring Data REST is a powerful tool for effortlessly exporting JPA repositories as RESTful resources. It significantly reduces the boilerplate code required to set up REST APIs for your data model.
By combining the capabilities of Spring Data repositories, Spring MVC, HATEOAS, and content negotiation, Spring Data REST simplifies the process of building hypermedia-driven RESTful APIs. However, it's important to be mindful of security considerations and the need for custom endpoints in more complex scenarios.
So, the next time you need to expose your JPA repositories as RESTful APIs, take advantage of Spring Data REST and let it work its magic for you!
If you found this article helpful and want to dive deeper into Spring Data REST, check out the official Spring Data REST documentation.
Happy coding with Spring Data REST!
Checkout our other articles