Unlocking Java EE: Master Camel Routes for Efficient Coding
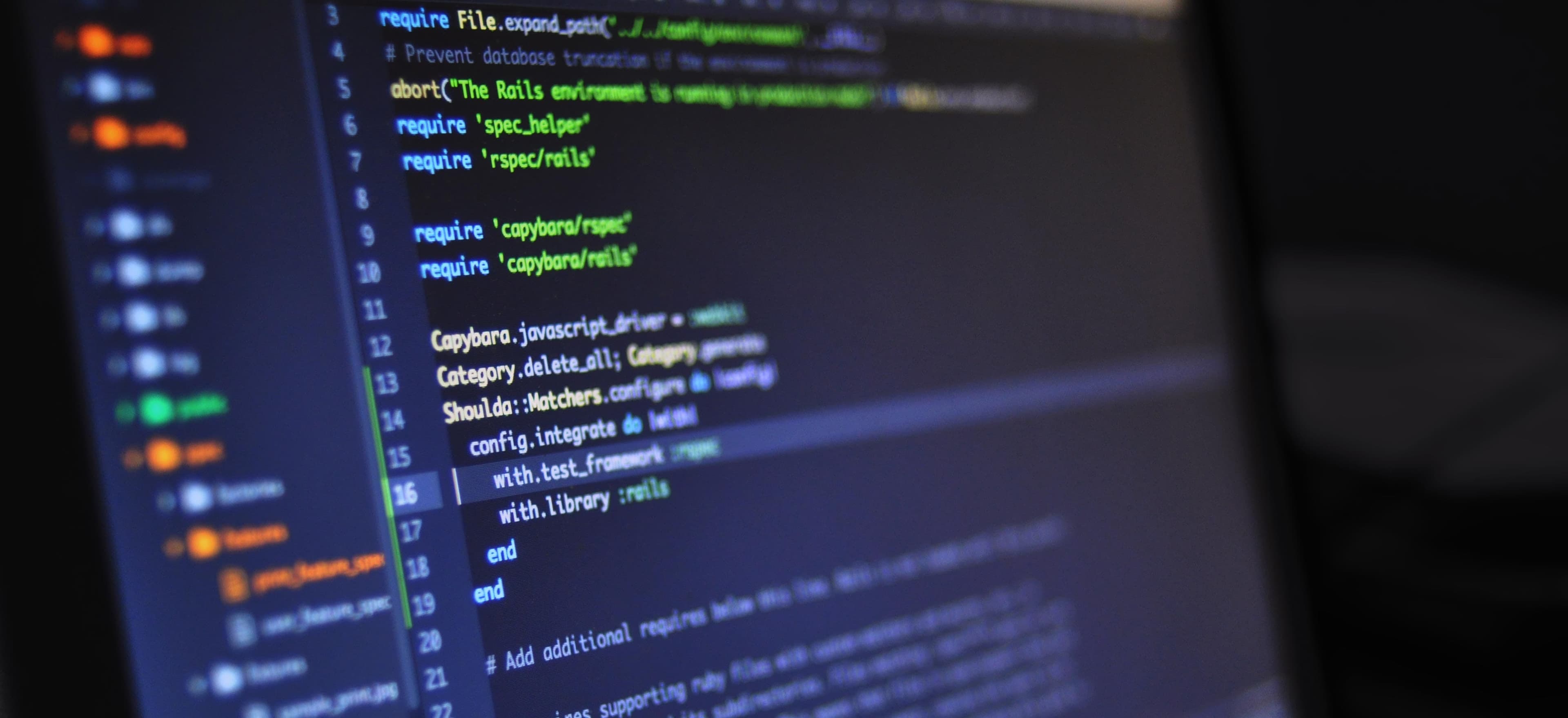
- Published on
Unlocking Java EE: Master Camel Routes for Efficient Coding
In the world of Java EE, Apache Camel has emerged as a powerhouse for facilitating seamless integration and fostering efficient coding practices. By mastering Camel routes, developers can streamline the process of routing and mediating the exchange of data between a wide array of technologies. This article serves as a comprehensive guide to understanding and implementing Camel routes in Java EE.
What are Camel Routes?
At its core, a Camel route defines the path that a message will take as it moves from one endpoint to another. This routing is based on a set of rules and actions, allowing for message mediation, transformation, and enrichment along the way. Camel routes are constructed using the Enterprise Integration Patterns (EIP), enabling developers to elegantly solve complex integration challenges.
Getting Started with Camel Routes
Let's kick off our exploration of Camel routes by setting up a simple Maven project. Ensure you have the Camel Maven plugin installed, and then add the necessary dependencies to your project's pom.xml
file.
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>{version}</version>
</dependency>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-spring</artifactId>
<version>{version}</version>
</dependency>
<!-- Add other dependencies as per your requirements -->
Once the project is set up, create a new Camel route by extending the RouteBuilder
class and implementing the configure
method.
import org.apache.camel.builder.RouteBuilder;
public class MyCamelRoute extends RouteBuilder {
@Override
public void configure() throws Exception {
from("direct:start")
.to("log:mylogger?level=INFO");
}
}
In this basic example, a route is configured to consume messages from the direct:start
endpoint and log them with the level set to INFO. As you delve deeper into Camel, you will discover a rich set of components and EIPs that can be leveraged in your routes.
The Power of Components in Camel Routes
Camel embraces a wide variety of components that serve as connectors to different systems and protocols. These components enable seamless integration with technologies such as JMS, HTTP, JDBC, and many more. By employing components in your Camel routes, you can effortlessly orchestrate the flow of data between disparate systems.
Let's take a look at an example where the HTTP component is used to build a simple route that exposes a RESTful service.
from("jetty:http://0.0.0.0:8080/myservice")
.to("log:mylogger?level=INFO")
.transform().constant("Response from the service");
In this example, the route listens for HTTP requests on the specified URI, logs the received messages, and sends a constant response. This illustrates how easy it is to integrate HTTP functionality into your Camel routes.
Managing Message Content with EIPs
Enterprise Integration Patterns play a pivotal role in Camel's capabilities. These patterns offer a standardized way to address common integration challenges, shaping the flow of messages as they traverse through Camel routes. Let's delve into a few essential EIPs that demonstrate their significance in message mediation.
Content-Based Router (CBR)
The Content-Based Router helps make routing decisions based on message content. This is particularly useful when dealing with messages of varying types or characteristics.
from("direct:start")
.choice()
.when(header("type").isEqualTo("important"))
.to("direct:important")
.otherwise()
.to("direct:unimportant");
In this example, the route examines the message's header to direct it to either the 'important' or 'unimportant' processing path. This powerful way of routing based on content can greatly enhance the flexibility of your integration solutions.
Splitter
The Splitter EIP allows for the splitting of a message into parts, subsequently processing each part individually.
from("direct:start")
.split(body().tokenize(","))
.to("log:mylogger?level=INFO");
Here, the route splits the message's body based on comma delimiters, logging each part separately. Utilizing the Splitter EIP enables efficient handling of complex message structures.
Error Handling and Fault Tolerance
Error handling is a critical aspect of any integration solution. Camel offers robust features for managing errors and ensuring fault tolerance within your routes.
Exception Clause
In the event of an exception, Camel allows you to gracefully handle errors using the doTry, doCatch, and doFinally construct.
from("direct:start")
.doTry()
.to("bean:myBean?method=process")
.doCatch(Exception.class)
.to("log:mylogger?level=ERROR&showStacktraces=true")
.end();
This example showcases how exceptions thrown during message processing can be caught and logged, preventing them from disrupting the flow of the route.
Dead Letter Channel
The Dead Letter Channel provides a reliable mechanism for handling failed messages by redirecting them to a separate endpoint for further processing.
errorHandler(deadLetterChannel("log:errors?level=ERROR"));
from("direct:start")
.to("seda:queue");
In this instance, any failed messages are routed to the 'log:errors' endpoint, securing them for subsequent analysis and resolution.
Testing Camel Routes
Unit testing Camel routes is an indispensable practice to ensure the reliability and correctness of your integration logic. The Camel framework provides excellent support for testing routes through the use of the Camel Test Kit.
Here's a concise example demonstrating how to use the Camel Test Kit to test a simple Camel route.
public class MyCamelRouteTest extends CamelTestSupport {
@Override
protected RoutesBuilder createRouteBuilder() throws Exception {
return new MyCamelRoute();
}
@Test
public void testRoute() throws Exception {
getMockEndpoint("mock:result").expectedMessageCount(1);
template.sendBody("direct:start", "Test Message");
assertMockEndpointsSatisfied();
}
}
By extending CamelTestSupport
and utilizing MockEndpoint
, you can effectively write unit tests to validate the behavior of your Camel routes.
To Wrap Things Up
In this article, we've traversed the landscape of Camel routes within Java EE, unveiling their capabilities and significance in the realm of integration. From constructing basic routes to harnessing powerful EIPs and handling errors, mastering Camel routes empowers developers to craft efficient and resilient integration solutions.
As you continue your journey with Camel, exploring its extensive documentation and vibrant community will further enrich your understanding. Embrace the elegance of Camel routes, and unlock a realm of seamless integration within your Java EE projects.
Let Apache Camel pave the way for your integration triumphs!
Checkout our other articles