Navigating Rate Limits: Mastering Twitter's Streaming API
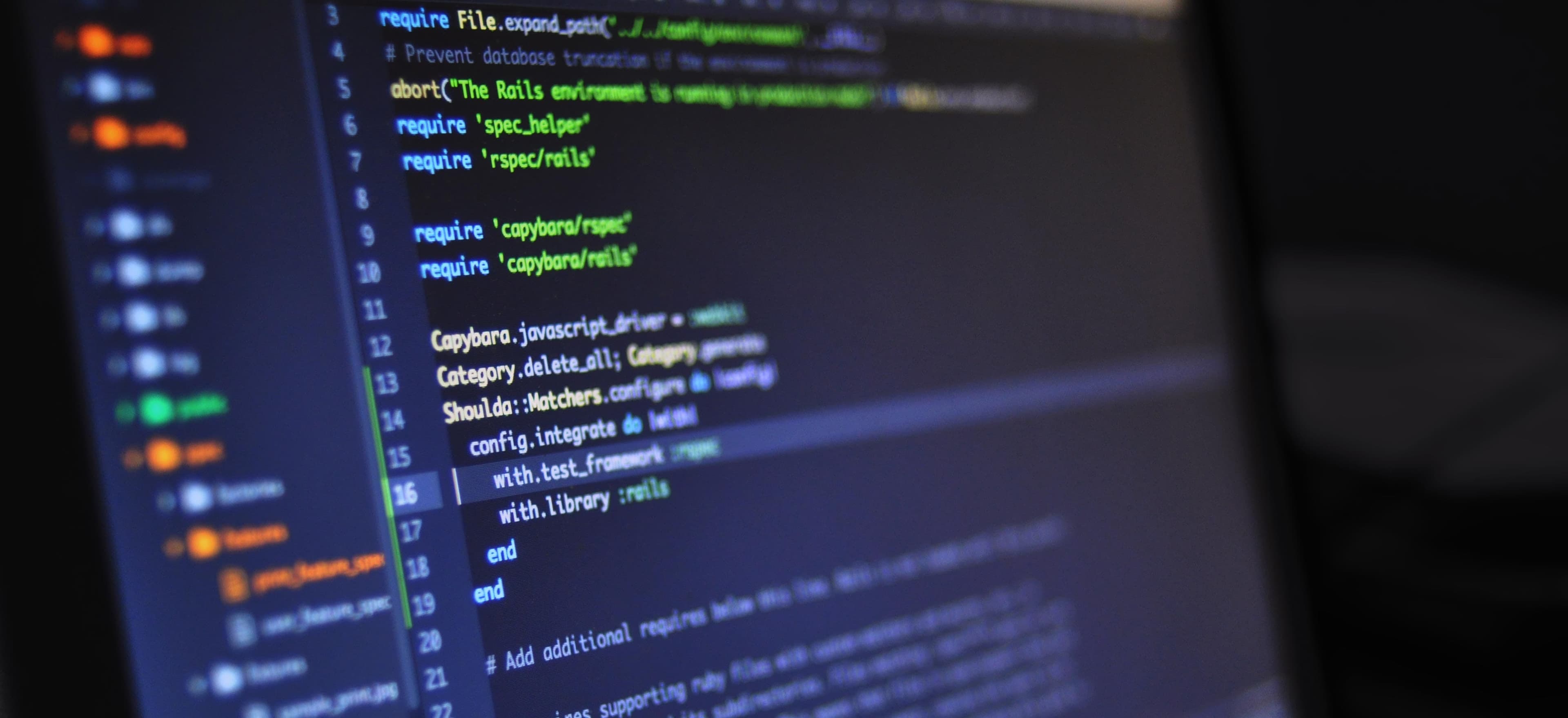
- Published on
Navigating Rate Limits: Mastering Twitter's Streaming API
Establishing the Context
In the world of social media and real-time data analysis, Twitter's Streaming API is a powerful tool for developers to access live tweets and user activities. However, like any other API, Twitter's Streaming API comes with rate limits that developers must be mindful of to avoid being throttled.
Understanding and navigating rate limits is crucial for effectively utilizing Twitter's Streaming API. In this blog post, we will delve into the concept of rate limits, explore how they apply to Twitter's Streaming API, and discuss strategies for working within these limits to build efficient and scalable applications.
What are Rate Limits?
In the context of web APIs, rate limits refer to the constraints imposed on the number of requests that a client can make to the API within a given time period. These limits are put in place by API providers to ensure fair usage, prevent abuse, and maintain system performance and stability.
When it comes to Twitter's Streaming API, rate limits are set to govern the volume of data that a developer can access over a specified time frame. The API enforces limits on the number of connections, the number of messages per second, and the overall volume of data that can be retrieved.
Rate Limit Strategies
1. Understanding Rate Limit Parameters
Before diving into code, it's crucial to understand the rate limit parameters specific to Twitter's Streaming API. Twitter's API documentation provides detailed information about rate limits, including the types of endpoints, their corresponding rate limits, and how these limits are enforced.
2. Implementing Exponential Backoff
When working with the Twitter Streaming API, it's essential to handle rate limit errors gracefully. Exponential backoff is a technique where the client retries the request after an increasing amount of time upon encountering a rate limit error. This approach prevents overwhelming the API and increases the chances of successful requests.
Here's an example of implementing exponential backoff in Java:
int retries = 0;
while (retries < MAX_RETRIES) {
try {
// Make request to Twitter's Streaming API
// Process the received data
break; // Break out of the loop if successful
} catch (RateLimitException e) {
// Handle rate limit error
long waitTime = (long) Math.pow(2, retries) * 1000;
Thread.sleep(waitTime);
retries++;
}
}
In this example, the MAX_RETRIES
constant determines the maximum number of retry attempts, and the wait time between retries grows exponentially with each attempt.
3. Monitoring Rate Limit Usage
It's important to keep track of your API usage to avoid hitting rate limits unexpectedly. Twitter provides endpoint-specific rate limit status endpoints that allow you to query the current rate limit status for your application.
In Java, you can use the Twitter4J library to retrieve rate limit status:
Map<String, RateLimitStatus> rateLimitStatus = twitter.getRateLimitStatus();
By monitoring rate limit usage, you can adjust your application's behavior dynamically and optimize its performance within the enforced limits.
Rate Limit Handling Best Practices
-
Caching Data: If your application frequently accesses the same data, consider caching the results to minimize the number of requests made to the API. This reduces the likelihood of hitting rate limits for repetitive queries.
-
Optimizing Requests: Make efficient use of available endpoints and query parameters to retrieve the necessary data in a single request whenever possible. Minimizing unnecessary requests helps conserve your rate limit quota.
-
Prioritizing Data: Identify and prioritize the most critical data for your application. By focusing on essential data retrieval, you can allocate your rate limit allowance to the most valuable and relevant information.
To Wrap Things Up
Mastering Twitter's Streaming API involves not only understanding the technical aspects of data retrieval but also navigating the intricacies of rate limits. By comprehending rate limits, implementing effective strategies such as exponential backoff, and continuously monitoring API usage, developers can build robust and scalable applications that harness the power of real-time Twitter data.
As you embark on your journey to integrate Twitter's Streaming API into your Java applications, remember that adeptly managing rate limits is the key to unlocking the full potential of real-time data access.
Incorporate these rate limit strategies, and watch your Twitter-powered applications thrive with efficiency and reliability.
References:
- Twitter Developer Documentation
- Twitter4J Library
Start implementing these strategies in your Java applications and maximize the potential of Twitter's Streaming API while staying within the bounds of rate limits. Happy coding!
Checkout our other articles