Fixing Memory Leaks: Resetting Thread Context Class Loaders
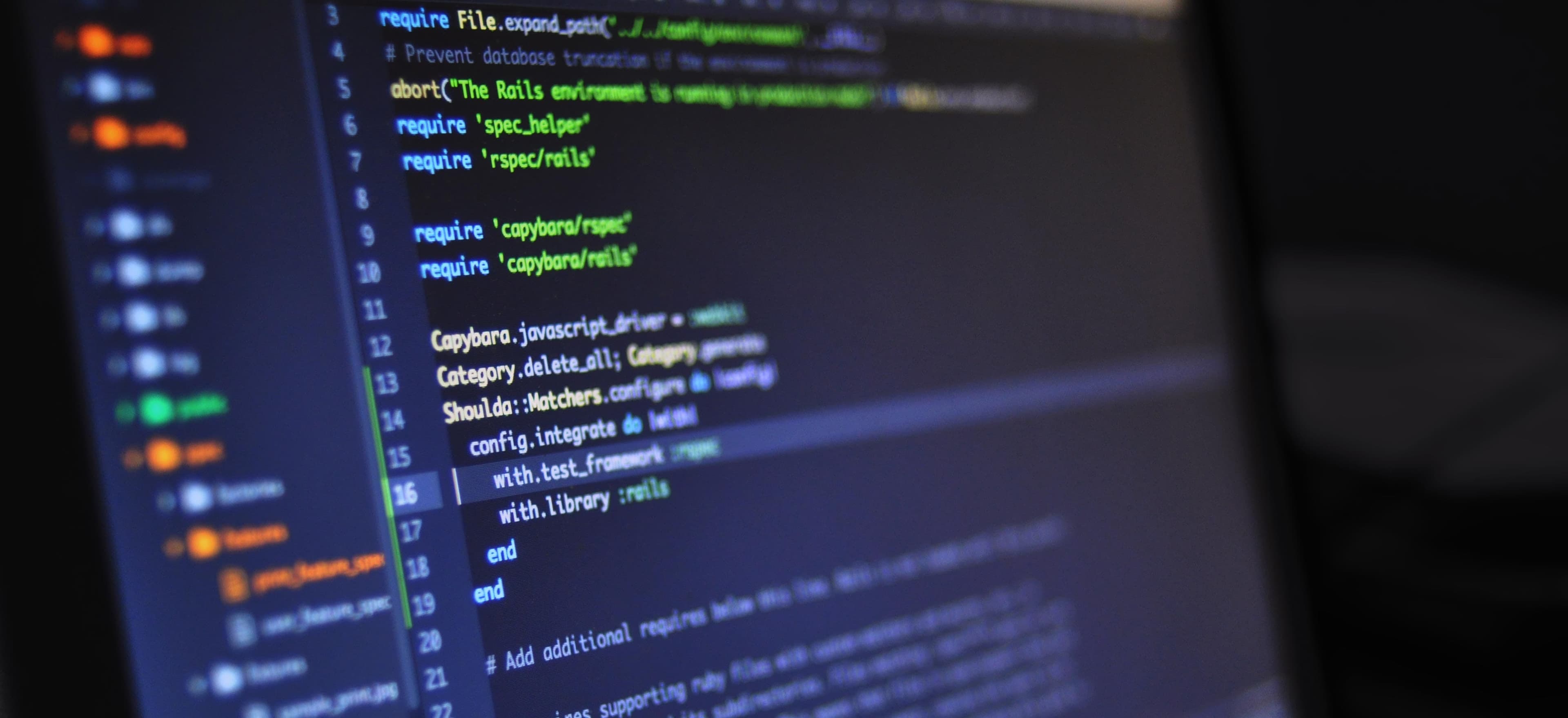
- Published on
Fixing Memory Leaks: Resetting Thread Context Class Loaders
Memory leaks in Java applications can be a source of frustration for developers. Among the many potential causes of memory leaks, an often overlooked yet critical factor is the improper handling of class loaders within the application. This is particularly prevalent in scenarios where thread context class loaders are not reset, leading to memory leaks due to the retention of class loader instances and their associated classes, preventing their garbage collection.
In this article, we will explore the concept of thread context class loaders, understand how memory leaks can occur due to their mismanagement, and then delve into the practical aspects of resetting thread context class loaders to prevent memory leaks in Java applications.
Understanding Thread Context Class Loaders
In Java, each thread has an associated context class loader that helps in locating and loading classes and resources. This is especially useful in scenarios such as JNDI lookups and dynamic class loading, where the default class loader may not suffice.
The thread context class loader is set and retrieved using the methods Thread.currentThread().getContextClassLoader()
and Thread.currentThread().setContextClassLoader(ClassLoader cl)
respectively.
How Memory Leaks Occur
When a class is loaded by a class loader and the class has a reference to a resource, the class loader holds a reference to the resource as well. If these references are not released properly, for example, by not resetting the thread context class loader, the classes and resources will not be garbage collected even after they are no longer required. This results in memory leaks as the class loader and its associated classes remain in memory, consuming valuable resources and potentially causing performance degradation.
Practical Solution: Resetting Thread Context Class Loaders
To address memory leaks related to thread context class loaders, it is essential to reset the class loader when its work is done, ensuring that no unnecessary references are retained.
Let's consider an example to illustrate the potential issue and its resolution.
Example Scenario
Suppose we have a web application running on a servlet container, and within this application, a custom Thread
is created to perform some background tasks. The custom Thread
uses a different class loader to load classes and resources. However, after the custom Thread
completes its tasks, the thread context class loader is not reset, leading to potential memory leaks.
Code Example
public class CustomThread extends Thread {
public void run() {
// Perform background tasks using a custom class loader
// Reset the thread context class loader
Thread.currentThread().setContextClassLoader(getClass().getClassLoader());
}
}
In this example, upon completion of the background tasks, we reset the thread context class loader using Thread.currentThread().setContextClassLoader(getClass().getClassLoader())
. By resetting the class loader to the system class loader, we ensure that any references held by the custom class loader can be released, preventing memory leaks.
Best Practices
Here are some best practices to consider when resetting thread context class loaders:
- Reset at the end of custom thread execution: Ensure that the thread context class loader is reset at the end of a custom
Thread
execution to release any held references. - Use try-finally block: When setting the thread context class loader in a custom
Thread
, consider using a try-finally block to guarantee that the reset operation is always performed, even in the event of exceptions.
Additional Considerations
It's important to note that the issue of memory leaks due to unreset thread context class loaders is not limited to custom threads in web applications but can also occur in various application scenarios involving dynamic class loading and resource handling.
The Bottom Line
In Java applications, memory leaks can stem from various sources, and the mismanagement of thread context class loaders is a significant yet often overlooked contributor. By understanding how memory leaks occur due to unreset thread context class loaders and adopting the practice of resetting them appropriately, developers can take proactive measures to mitigate memory leak issues and ensure the efficient utilization of resources within their applications.
By incorporating the best practices outlined in this article, it is possible to address memory leak concerns related to thread context class loaders and promote the robustness and stability of Java applications.
For further exploration of memory management and optimization in Java, consider diving into Java's Garbage Collection mechanisms, which play a pivotal role in reclaiming memory occupied by unused objects, thus preventing memory leaks and enhancing application performance.
To stay updated with the latest Java best practices and tips, explore the comprehensive resources available on Java Platform, Standard Edition and Java Community Process.
Checkout our other articles