Boost Log4j2: Slash Costs with Non-Logging Logger Tricks!
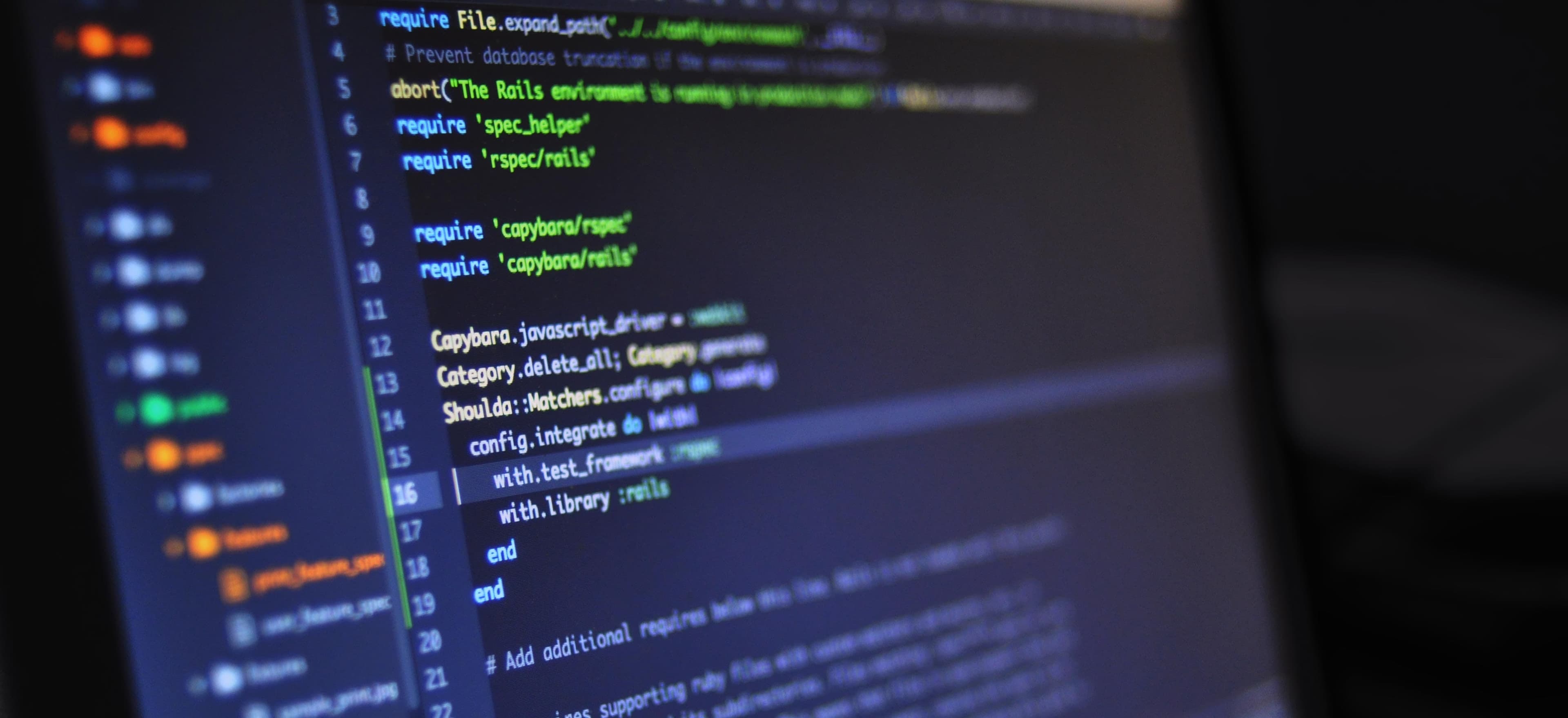
- Published on
Boost Log4j2: Slash Costs with Non-Logging Logger Tricks!
Log4j2 is a powerful and popular logging library for Java, known for its performance and flexibility. However, there are times when logging is expensive and might not be necessary, especially in hot code paths. In this article, we'll explore some non-logging logger tricks that can help you optimize your Log4j2 usage and slash costs in your applications.
1. Conditional Logging
When using Log4j2, it's essential to avoid unnecessary string concatenation and processing for log messages that may not even be logged. By utilizing the conditional level checks, you can ensure that expensive logging operations are only performed when necessary.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class ExampleClass {
private static final Logger logger = LogManager.getLogger(ExampleClass.class);
public void performExpensiveOperation() {
if (logger.isDebugEnabled()) {
// Expensive operation
logger.debug("Expensive operation result: " + expensiveOperation());
}
}
private String expensiveOperation() {
// Perform the expensive operation
return "result";
}
}
In this example, the performExpensiveOperation
method checks if the debug level is enabled in the logger before performing the expensive operation and constructing the log message. This prevents the costly operation and string concatenation when debug logging is disabled.
2. Lambda Expressions for Lazy Logging
Log4j2 supports lambda expressions for lazy logging, which can be particularly useful for logging complex objects or messages that require expensive computation. By using lambda expressions, you can defer the execution of the log message construction until it's determined that the message will actually be logged.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class ExampleClass {
private static final Logger logger = LogManager.getLogger(ExampleClass.class);
public void logWithLambda() {
SomeObject someObject = // Retrieve or construct the object
logger.debug(() -> "Complex object details: " + someObject.computeDetails());
}
}
In this example, the computeDetails
method of SomeObject
will only be executed if the debug level is enabled in the logger. This lazy evaluation can significantly reduce unnecessary computation and object manipulation when the log message is not logged.
3. Custom Log Levels
Log4j2 allows you to define custom log levels, which can be utilized to fine-tune the logging behavior based on specific application requirements. By using custom log levels, you can have more control over the granularity of logging and optimize performance by filtering out less critical logs at runtime.
import org.apache.logging.log4j.Level;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class ExampleClass {
private static final Logger logger = LogManager.getLogger(ExampleClass.class);
private static final Level IMPORTANT = Level.forName("IMPORTANT", 350);
public void logImportantMessage() {
if (logger.isEnabled(IMPORTANT)) {
logger.log(IMPORTANT, "This is an important message");
}
}
}
In this example, a custom log level "IMPORTANT" is defined with a specific integer value. The logImportantMessage
method checks if the logger is enabled for the custom level before logging the important message, allowing for fine-grained control over which logs are processed.
Wrapping Up
By leveraging conditional logging, lambda expressions for lazy logging, and custom log levels, you can optimize your Log4j2 usage and minimize the performance impact of logging in critical sections of your application. These non-logging logger tricks can help you slash costs and ensure that your logging activities are efficient and effective.
Integrating these techniques into your Log4j2 usage can lead to improved performance and resource utilization, especially in high-throughput and low-latency systems where efficient logging can make a significant impact.
Enhance your knowledge by exploring the official Log4j2 documentation.