Master Multi-Version Java Modules with Maven Made Easy
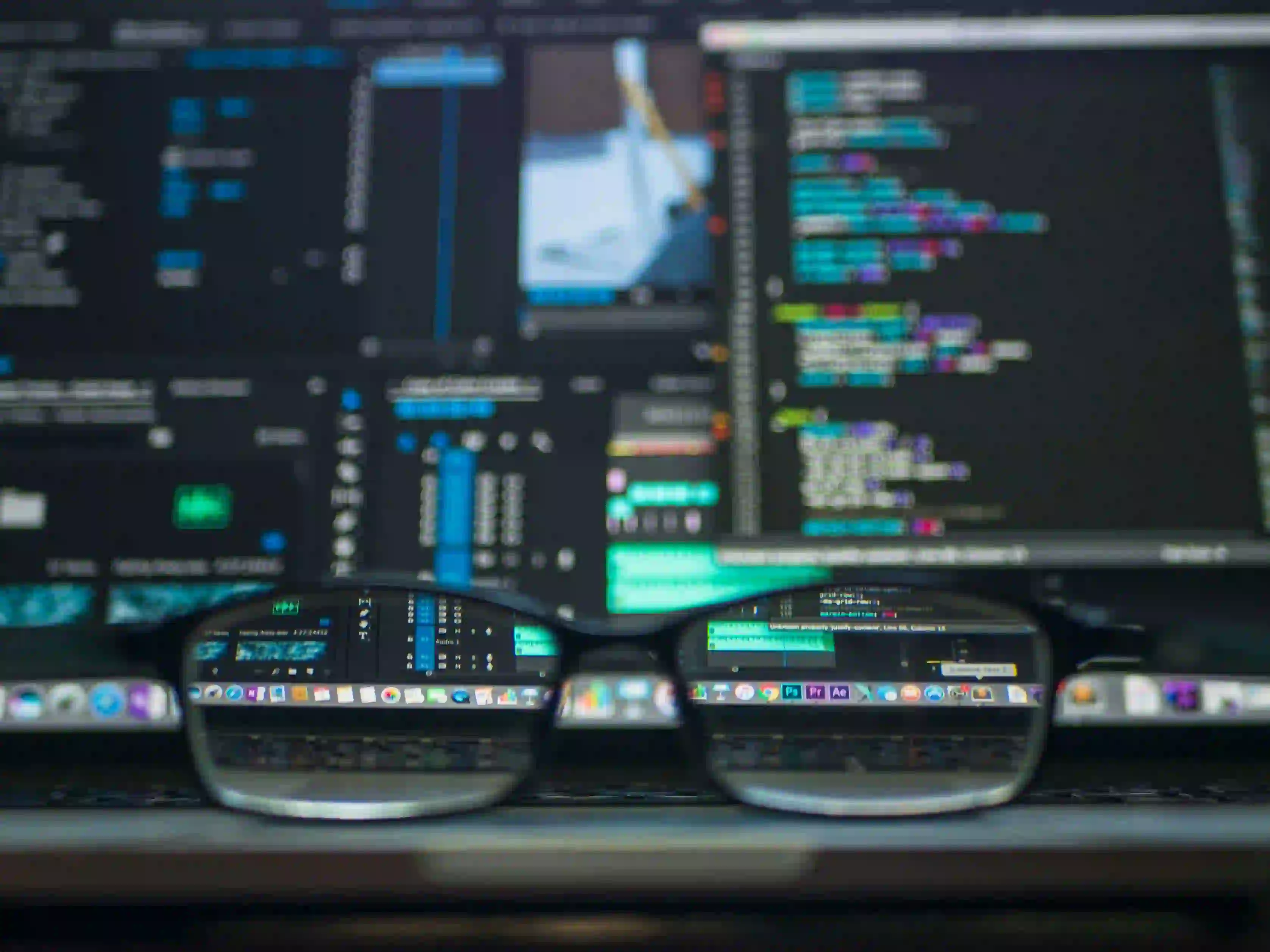
Master Multi-Version Java Modules with Maven Made Easy
When working on Java projects, managing multiple versions of modules with Maven can be a daunting task. However, with the right approach, it can become a straightforward and efficient process. In this guide, you will learn how to master multi-version Java modules with Maven, enabling you to seamlessly handle different versions of modules within your project.
Understanding the Need for Multi-Version Java Modules
In the complex ecosystem of Java development, it is not uncommon to encounter the need for multiple versions of modules within a single project. This necessity often arises when working with libraries or dependencies that have undergone version updates, yet coexist within the same codebase. It is imperative to address this requirement in a way that ensures modularity, version compatibility, and overall stability of the project.
Leveraging Maven for Multi-Version Module Management
Maven, being a powerful build automation tool primarily used for Java projects, provides robust capabilities for handling multi-version modules. By leveraging Maven's features, such as profiles and dependency management, developers can effectively address the challenges posed by multiple module versions.
Working with Maven Profiles
Maven profiles allow you to customize the build process based on different conditions. This feature is instrumental in managing multi-version modules within a project. By defining profiles in the pom.xml
file, developers can specify different sets of dependencies and configurations for each module version.
Creating Maven Profiles
Let's consider a scenario where a project requires support for two different versions of a specific module, denoted as module-1.0
and module-2.0
. We can create profiles for each version in the pom.xml
as follows:
<profiles>
<profile>
<id>module-1.0</id>
<dependencies>
<!-- Define dependencies for module-1.0 -->
</dependencies>
</profile>
<profile>
<id>module-2.0</id>
<dependencies>
<!-- Define dependencies for module-2.0 -->
</dependencies>
</profile>
</profiles>
Activating Maven Profiles
Profiles can be activated based on various conditions such as environment variables, property values, or JDK versions. For instance, to activate the profile for module-1.0
based on a specific property value, you can include the following configuration within the pom.xml
:
<properties>
<module.version>1.0</module.version>
</properties>
<profiles>
<profile>
<id>module-1.0</id>
<activation>
<property>
<name>module.version</name>
<value>1.0</value>
</property>
</activation>
<!-- Define dependencies for module-1.0 -->
</profile>
</profiles>
By incorporating Maven profiles, you can effectively manage different versions of modules within your project, ensuring proper isolation and configuration based on the specified conditions.
Dependency Management for Multi-Version Modules
Maven's dependency management capabilities play a critical role in handling multi-version modules. By defining and organizing dependencies appropriately, you can streamline the integration of various module versions into your project.
Declaring Module Dependencies
When working with multiple module versions, it's essential to declare dependencies specific to each version. This ensures that the project uses the correct version based on the active profile. In the pom.xml
, you can define module dependencies within the corresponding profiles, as demonstrated below:
<profiles>
<profile>
<id>module-1.0</id>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>module</artifactId>
<version>1.0</version>
</dependency>
</dependencies>
</profile>
<profile>
<id>module-2.0</id>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>module</artifactId>
<version>2.0</version>
</dependency>
</dependencies>
</profile>
</profiles>
By explicitly specifying the module dependencies within the corresponding profiles, you ensure that the correct version is utilized based on the active profile, facilitating seamless integration of multi-version modules.
Enhancing Modularity and Flexibility
Efficiently managing multi-version Java modules with Maven not only addresses the immediate need for version compatibility but also enhances the modularity and flexibility of the project. By utilizing Maven's capabilities, you can establish a robust foundation for handling diverse module versions, empowering you to adapt to evolving requirements and maintain a structured, scalable codebase.
My Closing Thoughts on the Matter
Mastering multi-version Java module management with Maven is a valuable skill that equips developers to navigate the complexities of versioned dependencies within their projects effectively. By leveraging Maven profiles, dependency management, and activation mechanisms, you can streamline the integration of multiple module versions while maintaining modularity and stability. With these techniques at your disposal, you are well-equipped to conquer the challenges associated with handling diverse module versions in your Java projects.
Incorporate these best practices into your development workflow and elevate your proficiency in managing multi-version Java modules with Maven.