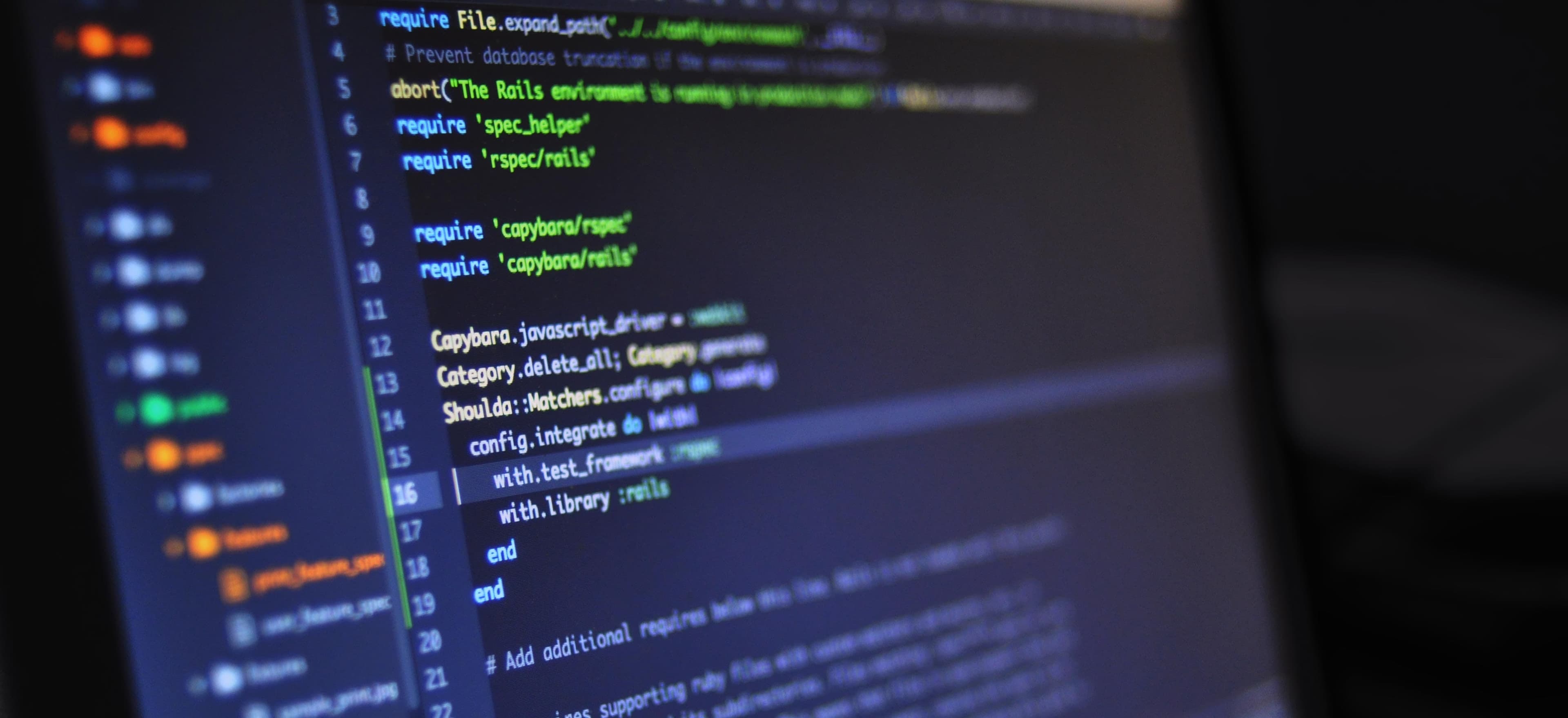
- Published on
Best Practices for Securely Storing SQL Passwords
In today's interconnected world, securing sensitive information is more critical than ever. Passwords serve as the gatekeepers to our databases, and mishandling them can lead to breaches with disastrous consequences. This blog post will guide you through the best practices for securely storing SQL passwords, ensuring that you protect your data while maintaining accessibility for authorized users.
Understanding the Risks
Before we delve into best practices, it's important to understand the risks associated with poor password management:
- Data Breaches: Unauthorized entities gaining access to sensitive data.
- Identity Theft: Stolen credentials can lead to identity theft, impacting both individuals and organizations.
- Reputation Damage: A security breach can shatter the trust of customers and stakeholders.
With this knowledge, let's explore the best practices.
1. Use Strong, Unique Passwords
One of the foundational aspects of password security is using strong, unique passwords.
Why?
Weak passwords are easily explorable through brute-force attacks, and reusing passwords across various systems increases vulnerability if one system is compromised.
Implementation
When creating passwords, consider:
- Length: A minimum of 12 characters.
- Complexity: Use a mix of uppercase letters, lowercase letters, numbers, and special characters.
- Uniqueness: Each system or service should have its own unique password.
Example:
# A strong password example
9mX#5yGh*pZ3!8jK
Tools to Use
Consider utilizing a password manager, such as LastPass or 1Password, to generate and store secure passwords.
2. Hash Passwords
Never store plain-text passwords in your SQL database. Instead, opt for hashing.
Why?
If your database is compromised, hashed passwords remain protected because they are not reversible.
Implementation
Use a robust hashing algorithm such as bcrypt, argon2, or PBKDF2. Each hashing method will significantly increase the time and computational resources needed to crack a password through brute-force attacks.
Example:
import org.mindrot.jbcrypt.BCrypt;
public class PasswordUtil {
// Hash a password
public static String hashPassword(String password) {
return BCrypt.hashpw(password, BCrypt.gensalt());
}
// Check a password against a stored hash
public static boolean checkPassword(String password, String hashed) {
return BCrypt.checkpw(password, hashed);
}
}
Commentary
In the above code snippet:
BCrypt.hashpw
hashes the password and generates a salt automatically.BCrypt.checkpw
allows you to compare the user's input with the stored hash.
3. Use Salts for Additional Security
Salting is the process of adding a unique, random string to each password before hashing it.
Why?
Salting ensures that even if two users have the same password, they will have different hashes stored in the database.
Implementation
Incorporate a random salt along with the hashing process:
import java.security.SecureRandom;
import java.util.Base64;
public class PasswordUtil {
private static byte[] generateSalt() {
SecureRandom random = new SecureRandom();
byte[] salt = new byte[16];
random.nextBytes(salt);
return salt;
}
public static String hashPasswordWithSalt(String password) {
byte[] salt = generateSalt();
String saltedPassword = password + Base64.getEncoder().encodeToString(salt);
return BCrypt.hashpw(saltedPassword, BCrypt.gensalt());
}
}
Commentary
Each time a password is hashed, a new salt is generated. This unique salt is then incorporated into the password hashing process.
4. Implement Secure Access Controls
Even with strong passwords, secure access controls are vital to minimize risks.
Why?
Proper access controls limit who can view and manipulate sensitive information, reducing the threat of insider attacks and accidental exposure.
Implementation
Implement role-based access controls in your databases:
- Grant users the least privilege necessary to perform their jobs.
- Regularly review user access and modify permissions as roles change.
SQL Example:
-- Create a role for read-only access
CREATE ROLE read_only;
-- Grant select permission to the role
GRANT SELECT ON my_database.* TO 'read_only';
-- Assign the role to a user
GRANT read_only TO 'username';
Commentary
This SQL command establishes a read_only
role, allowing users to view data without the ability to modify or delete it.
5. Regularly Update and Review Security Measures
It's essential to monitor and update security measures on a regular basis.
Why?
The landscape of cybersecurity threats is constantly evolving. Regular updates ensure you are protected against the latest vulnerabilities.
Implementation
- Schedule routine audits of password policies and database security.
- Stay informed about new security patches and promptly apply them.
Helpful Link: OWASP Password Storage Cheat Sheet
6. Enable Two-Factor Authentication (2FA)
Adding a second layer of authentication bolsters security significantly.
Why?
2FA ensures that even if a password is compromised, an additional verification factor is required for access.
Implementation
Implement a time-based one-time password (TOTP) mechanism:
import com.warrenstrange.googleauth.GoogleAuthenticator;
public class TwoFactorAuthUtil {
private static GoogleAuthenticator gAuth = new GoogleAuthenticator();
public static String createSecret() {
return gAuth.createCredentials().getKey();
}
public static boolean verifyCode(String secret, int code) {
return gAuth.getVerificationCode(secret) == code;
}
}
Commentary
In this snippet, Google Authenticator's library is utilized to generate a secret key and verify a code entered by the user during login. This adds a vital step to authentication.
Wrapping Up
Securing SQL passwords is an essential part of protecting your data. By following the outlined best practices, including using strong passwords, hashing with salt, enforcing access controls, regularly reviewing security measures, and implementing 2FA, you can enhance the security of your systems and mitigate the risks of data breaches.
Investing in these security measures is not just a best practice, but a necessity in today’s digital age. Start incorporating these recommendations today to safeguard your sensitive data!
For continuous learning, check out SQL Server Security Best Practices and stay informed about emerging threats and technology.
Note: Always stay updated on the latest security practices to keep your SQL database and its passwords secure.
Checkout our other articles