Overcoming Lombok Pitfalls: Safe Getters & Setters in Java
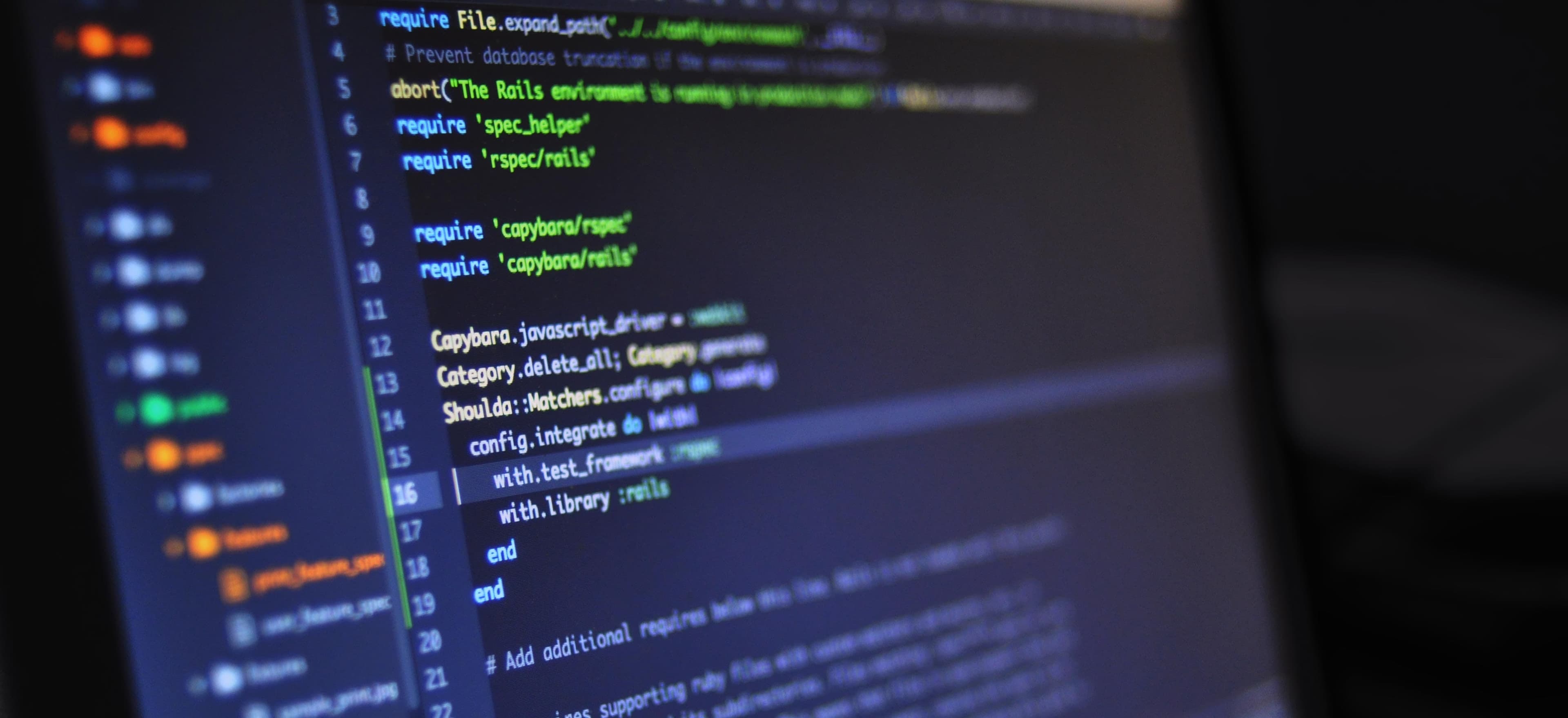
- Published on
Overcoming Lombok Pitfalls: Safe Getters & Setters in Java
In the realm of Java programming, Lombok has emerged as a popular tool for reducing boilerplate code. By using Lombok annotations such as @Getter
and @Setter
, developers can automatically generate getters and setters for their Java classes. This certainly streamlines the codebase and enhances readability. However, utilizing Lombok for getters and setters comes with its own set of uncertainties and potential pitfalls.
In this post, we will explore the potential issues with Lombok-generated getters and setters and shed light on how to implement safe and robust getters and setters in Java without compromising on code conciseness. We will delve into the significance of ensuring that getters and setters are implemented with security and encapsulation in mind. Let’s start by addressing the drawbacks associated with Lombok-generated getters and setters.
The Lombok Conundrum
Lombok allows developers to generate getters and setters with ease, ensuring that the class remains concise and readable. However, this convenience often leads to the oversight of encapsulation and security within the class. As a result, the class's internal state can be compromised, potentially leading to unexpected behaviors and security vulnerabilities.
When using Lombok to auto-generate getters and setters, it is crucial to recognize the trade-offs. While the reduction in boilerplate code is appealing, it is equally essential to ensure that the class's internal state is protected from unauthorized access and manipulation.
Safe Getters and Setters: Implementing Encapsulation
To overcome the pitfalls associated with Lombok-generated getters and setters, it is imperative to implement safe and encapsulated access to the class's attributes. Let’s consider an example where a simple User
class represents a user entity with a name
attribute. We will explore how to create safe getters and setters for the name
attribute to maintain encapsulation and data integrity.
public class User {
private String name;
public String getName() {
return this.name;
}
public void setName(String name) {
if (name != null && !name.isBlank()) {
this.name = name;
} else {
throw new IllegalArgumentException("Name cannot be null or empty");
}
}
}
In the above code snippet, we have explicitly defined the getName
and setName
methods, which provide controlled access to the name
attribute. By doing so, we enforce specific validation rules, ensuring that the name
attribute cannot be set to a null or empty value. This approach safeguards the integrity of the User
class by maintaining a valid state at all times.
Leveraging Lombok with Caution
While it is essential to implement customized getters and setters to uphold encapsulation and security, there are scenarios where leveraging Lombok cautiously can still be beneficial. The key lies in understanding when to rely on Lombok's automation and when to intervene with custom accessors.
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
public class Book {
private String title;
private String author;
}
In the above example, we utilize Lombok's @Getter
and @Setter
annotations to automatically generate the getters and setters for the title
and author
attributes of the Book
class. This approach significantly reduces the boilerplate code while maintaining the simplicity and readability of the class. However, it is important to note that for more complex validation and behavior, manually implementing getters and setters, as demonstrated earlier, is a preferable approach.
Safeguarding Against Insecure Access
Ensuring that getters and setters provide secure and encapsulated access to class attributes is paramount in Java development. It is crucial to consider scenarios where malicious or unintended access to the class's internal state can lead to adverse consequences. Let’s explore the implications of insecure access and how to mitigate such risks.
Consider a BankAccount
class with a balance
attribute, representing the account balance. Without proper encapsulation and security measures, unauthorized manipulation of the balance
attribute can lead to potential financial discrepancies and security breaches.
By implementing secure getters and setters, we can mitigate such risks and maintain the integrity of the BankAccount
class:
public class BankAccount {
private double balance;
public double getBalance() {
// Perform additional security checks if necessary
return this.balance;
}
public void setBalance(double balance) {
// Perform validation and additional security measures
if (balance >= 0) {
this.balance = balance;
} else {
throw new IllegalArgumentException("Invalid balance");
}
}
}
In the above example, the getBalance
and setBalance
methods are designed to provide secure access to the balance
attribute, ensuring that the account balance remains consistent and protected from unauthorized modifications.
Key Takeaways
In conclusion, while Lombok offers a convenient approach to auto-generate getters and setters, it is imperative to prioritize encapsulation and security when designing access to class attributes. By implementing customized getters and setters, Java developers can uphold data integrity and mitigate potential security risks, ensuring that the class remains robust and reliable.
Incorporating a balanced approach by leveraging Lombok for straightforward scenarios and custom accessors for intricate validation and security requirements can strike a harmonious chord between code conciseness and data integrity.
Adopting safe and encapsulated access to class attributes through meticulously crafted getters and setters is a fundamental aspect of Java development, safeguarding the integrity of class internals and fortifying the overall robustness of the codebase.
By adhering to the best practices outlined in this post, Java developers can overcome the pitfalls associated with Lombok-generated getters and setters, instilling confidence in the security and encapsulation of their code.