Mockito for Beginners: Avoiding Initial Pitfalls
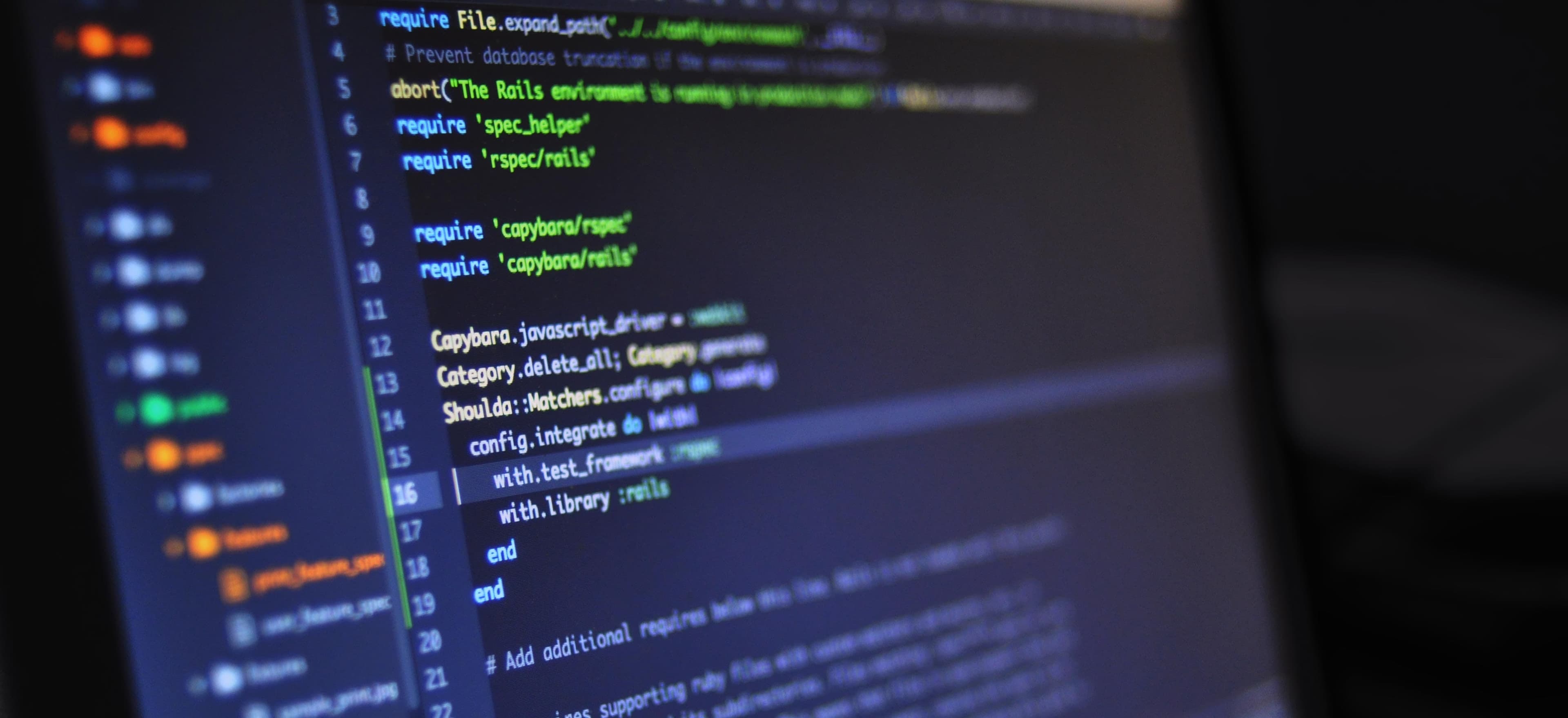
- Published on
Mockito for Beginners: Avoiding Initial Pitfalls
Are you new to Java development and eager to learn about mock testing? Look no further, as this blog post is designed to guide you through the initial stages of using Mockito, a popular mocking framework in Java, and help you avoid common pitfalls. Mockito is widely used for creating mock objects in automated unit tests, and by understanding its basic concepts and steering clear of potential stumbling blocks, you can jump-start your journey into the world of Mockito with confidence.
In this post, we'll cover some essential concepts of Mockito, common mistakes to avoid, and how to use Mockito effectively to write robust and maintainable unit tests.
Getting Started with Mockito
What is Mockito?
Mockito is a powerful and flexible open-source Java mocking framework that enables effective unit testing by creating mock objects. These mock objects simulate the behavior of real objects in controlled ways, allowing developers to isolate the code under test and verify its interactions with dependencies.
Key Concepts
Before diving into writing mock tests with Mockito, let's familiarize ourselves with some key concepts:
-
Mocks: These are the objects that simulate the behavior of real objects in controlled ways. When using Mockito, you can create mock objects to represent dependencies of the code under test.
-
Stubbing: This involves specifying the behavior of mock objects when certain methods are called. By stubbing methods, you can define the return values or behavior of the mock objects to suit your test scenarios.
-
Verification: Mockito allows you to verify how the code under test interacts with its dependencies. This involves checking if specific methods of mock objects are called with the expected arguments and how many times they are invoked.
-
Matchers: Mockito provides various argument matchers to define flexible and precise verification and stubbing. Matchers help you specify the expected arguments when setting up stubbing or verifying method invocations.
Common Pitfalls to Avoid
Pitfall 1: Misusing Matchers
One common mistake for beginners is misusing argument matchers when setting up stubbing or verification. It's essential to understand how to use matchers correctly based on the context of your tests.
// Incorrect usage of matchers
when(mockObject.method(anyString())).thenReturn(42);
// Correct usage of matchers
when(mockObject.method(eq("input"))).thenReturn(42);
Why?
The incorrect usage in the first line can lead to unexpected behaviors or even failing tests due to mismatched method invocations. Using matchers like eq("input")
ensures that the stubbing or verification is applied only when the exact specified argument is passed.
Pitfall 2: Overusing Mocks
While using mock objects is essential for isolating the code under test, overusing mocks can lead to overly coupled and less maintainable tests. It's crucial to strike a balance and recognize when to use real objects, fakes, or mocks in your tests.
// Overusing mocks
MockObject dependencyMock = mock(MockObject.class);
// Finding the right balance
RealObject realDependency = new RealObject();
Why?
Using real objects for simpler dependencies or creating fake implementations can sometimes be more effective and maintainable than creating and stubbing multiple mock objects.
Best Practices for Effective Mockito Usage
As you venture into the realm of Mockito, it's vital to follow best practices to ensure your mock tests are robust, maintainable, and beneficial for your development workflow.
Best Practice 1: Use Mocks Thoughtfully
When creating mock objects, consider the necessity and impact of each mock on your tests. Use mocks to isolate the code under test and verify interactions, but also evaluate if using real objects or fakes would lead to clearer and more maintainable tests.
Best Practice 2: Keep Verifications Clear
When verifying method invocations on mock objects, aim to keep the verifications clear and relevant to the behavior being tested. Avoid cluttering your test code with excessive verifications that might obscure the primary objectives of the tests.
// Clear and relevant verification
verify(mockObject, times(1)).method("input");
// Avoid cluttering verifications
verify(mockObject, times(1)).method(anyString());
Why?
Clear verifications enhance the readability of the tests and provide precise insights into the interactions between the code under test and its dependencies.
Best Practice 3: Leverage Annotations for Mockito Integration
In modern Java development, leveraging annotations for Mockito integration can streamline the setup and usage of mock objects in your tests. Annotations such as @Mock
, @InjectMocks
, and @RunWith(MockitoJUnitRunner.class)
can enhance the clarity and organization of your test code.
@Mock
MockObject mockObject;
@InjectMocks
TestClassWithDependencies testClass;
@Before
public void setUp() {
MockitoAnnotations.initMocks(this);
}
Why?
Using annotations simplifies and organizes the process of integrating Mockito into your tests, making the setup and usage of mock objects more explicit and systematic.
A Final Look
Embarking on the Mockito journey as a beginner can be an enriching experience, provided you grasp the foundational concepts and steer clear of the initial pitfalls. By understanding the nuances of stubbing, verification, and practical best practices, you can harness the power of Mockito to write effective mock tests for your Java applications.
In closing, remember that Mockito is a valuable tool for unit testing in Java and, with deliberate practice and exploration, you can harness its capabilities to write comprehensive, reliable tests that contribute to the overall quality and stability of your codebase.
As you continue to delve into the world of Java and Mockito, remember to stay curious, experiment with different test scenarios, and seek out additional resources and tutorials to deepen your understanding and proficiency in using Mockito for writing exemplary unit tests.
Happy mocking and testing!
Note: For further in-depth understanding and advanced Mockito usage, consider exploring the official Mockito documentation and other reputable resources.