Boost Your Testing Game: JUnit Meets Hamcrest Magic!
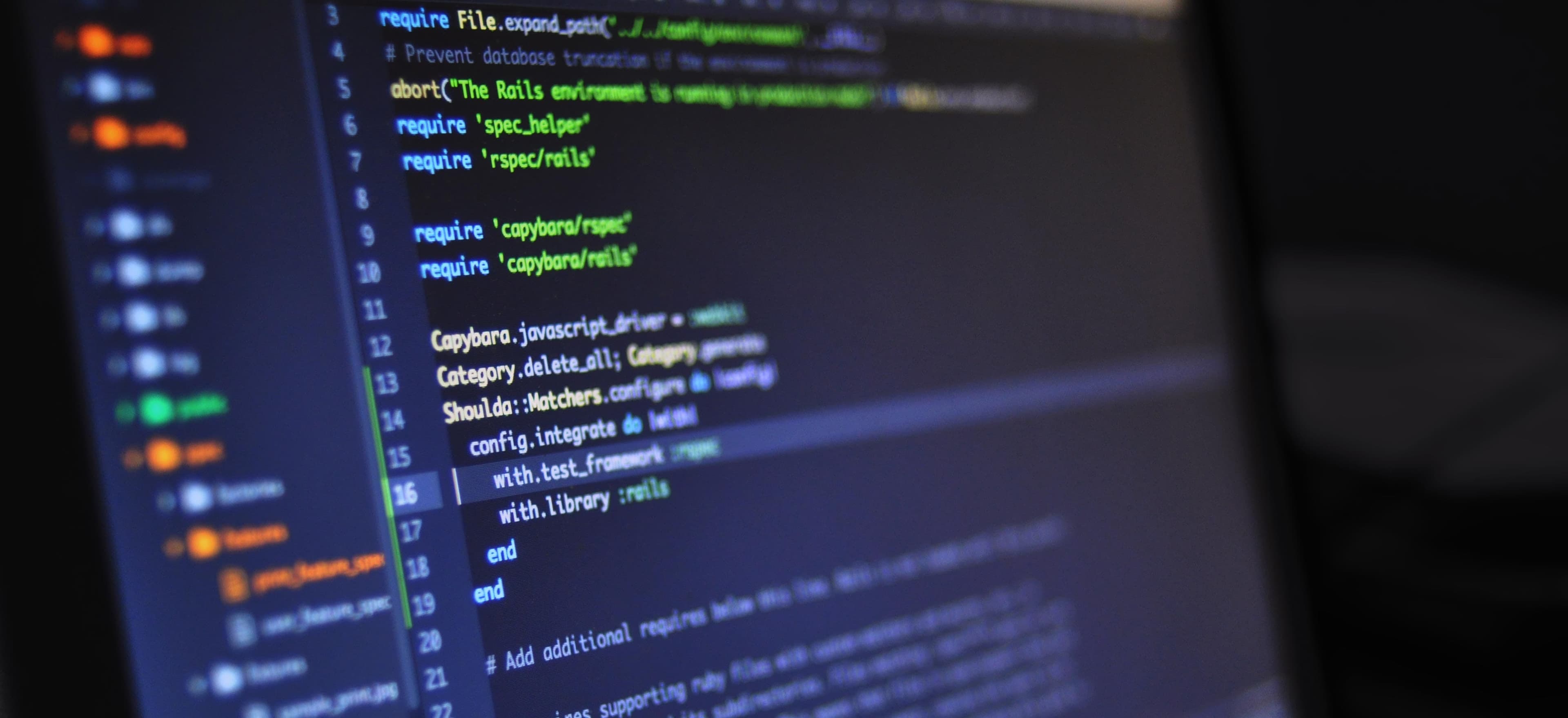
- Published on
Boost Your Testing Game: JUnit Meets Hamcrest Magic!
In the world of Java development, testing is an indispensable part of the software development lifecycle. The JUnit framework has long been the go-to choice for writing and executing unit tests. However, as your testing needs grow in complexity, you may find yourself wanting more expressive and powerful assertions than what JUnit offers. This is where Hamcrest comes into play, providing a wide range of matchers that can make your test code cleaner, more readable, and easier to maintain. In this post, we will dive into the magic of Hamcrest and how it seamlessly integrates with JUnit to elevate your testing game to the next level.
What is Hamcrest?
Hamcrest is a framework for writing matcher objects allowing 'match' rules to be defined declaratively. These matchers can be combined to create flexible and easily readable assertions. When used in conjunction with JUnit, Hamcrest can greatly enhance the expressiveness of your tests, making it easier to discern the intent and purpose of each assertion.
Getting Started with Hamcrest and JUnit
Before delving deep into how Hamcrest can level up your tests, let's set the stage by demonstrating how to integrate Hamcrest with JUnit.
First, you need to include Hamcrest and JUnit dependencies in your project. If you're using Maven, simply add the following dependencies to your pom.xml
:
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest-all</artifactId>
<version>1.3</version>
<scope>test</scope>
</dependency>
</dependencies>
Once the dependencies are in place, you can start leveraging Hamcrest matchers in your JUnit tests.
Writing Expressive Assertions with Hamcrest Matchers
Let's consider a simple scenario where you want to assert that a list contains elements in a specific order. With traditional JUnit assertions, the code might look like this:
@Test
public void testListOrderWithJUnit() {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
assertEquals(Arrays.asList("Alice", "Bob", "Charlie"), names);
}
While this certainly gets the job done, the intent of the assertion isn't immediately clear. Enter Hamcrest to make this assertion more expressive:
import static org.hamcrest.Matchers.contains;
@Test
public void testListOrderWithHamcrest() {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
assertThat(names, contains("Alice", "Bob", "Charlie"));
}
With Hamcrest's contains
matcher, the assertion reads almost like plain English, making the expected result evident at first glance. This is just a glimpse of the power that Hamcrest brings to your testing arsenal.
Custom Matchers for Enhanced Readability
One of the most beautiful aspects of Hamcrest is the ease with which you can create your custom matchers. These custom matchers can be used to encapsulate complex or frequently used assertions into a reusable and readable format.
Let's take an example. Say you have a class Person
with various attributes like name
, age
, and gender
. To improve the readability of your tests, you can create a custom matcher using Hamcrest to assert the equality of Person
objects based on their name
and age
.
import org.hamcrest.Description;
import org.hamcrest.TypeSafeMatcher;
public class PersonMatcher extends TypeSafeMatcher<Person> {
private final Person expected;
public PersonMatcher(Person expected) {
this.expected = expected;
}
@Override
protected boolean matchesSafely(Person actual) {
return actual.getName().equals(expected.getName()) && actual.getAge() == expected.getAge();
}
@Override
public void describeTo(Description description) {
description.appendText("a Person with name " + expected.getName() + " and age " + expected.getAge());
}
public static PersonMatcher matchesPerson(Person expected) {
return new PersonMatcher(expected);
}
}
Now, you can use this custom matcher in your test:
@Test
public void testCustomMatcher() {
Person expectedPerson = new Person("Alice", 30);
Person actualPerson = new Person("Alice", 30);
assertThat(actualPerson, matchesPerson(expectedPerson));
}
By creating custom matchers, you can encapsulate complex comparison logic, leading to more readable and maintainable test code.
Combining Matchers for Complex Assertions
Hamcrest allows you to combine matchers to form complex assertions, enabling you to express intricate conditions with ease. Consider a scenario where you want to verify that a Person
object has a name starting with "A" and an age between 20 and 40. With Hamcrest, you can compose matchers to achieve this succinctly:
import static org.hamcrest.Matchers.allOf;
import static org.hamcrest.Matchers.startsWith;
import static org.hamcrest.Matchers.greaterThanOrEqualTo;
import static org.hamcrest.Matchers.lessThanOrEqualTo;
@Test
public void testCombinedMatchers() {
Person person = new Person("Alice", 30);
assertThat(person.getName(), startsWith("A"));
assertThat(person.getAge(), allOf(greaterThanOrEqualTo(20), lessThanOrEqualTo(40)));
}
By using the allOf
matcher, you ensure that both conditions are satisfied, making your assertion highly readable and comprehensible.
Leverage Hamcrest in JUnit 5
If you're using JUnit 5, you can seamlessly integrate Hamcrest matchers using the assertThat
method provided by JUnit 5's Assertions
class. All you need to do is include the hamcrest
dependency in your build, and you're ready to harness the power of Hamcrest in the world of JUnit 5.
Bringing It All Together
Incorporating Hamcrest into your JUnit tests can significantly enhance the readability and expressiveness of your assertions. By leveraging Hamcrest's rich set of matchers and the ability to create custom matchers, you can write clear, succinct, and maintainable tests. The seamless integration of Hamcrest with JUnit makes it a compelling choice for anyone looking to raise the bar for their testing practices.
So, the next time you find yourself crafting verbose and convoluted assertions in your JUnit tests, remember the magic of Hamcrest and let it work wonders in transforming your testing experience.
Enhance your testing game with the powerful combination of JUnit and Hamcrest - your future self (and fellow developers) will thank you!
Remember, the code and assertions you write today are the foundation of reliable and maintainable software tomorrow. Happy testing with Hamcrest and JUnit!
Now, go forth and write expressive, readable, and maintainable tests with Hamcrest and JUnit!