Double Trouble: Our Hibernate JDBC Traffic Mishap Unveiled
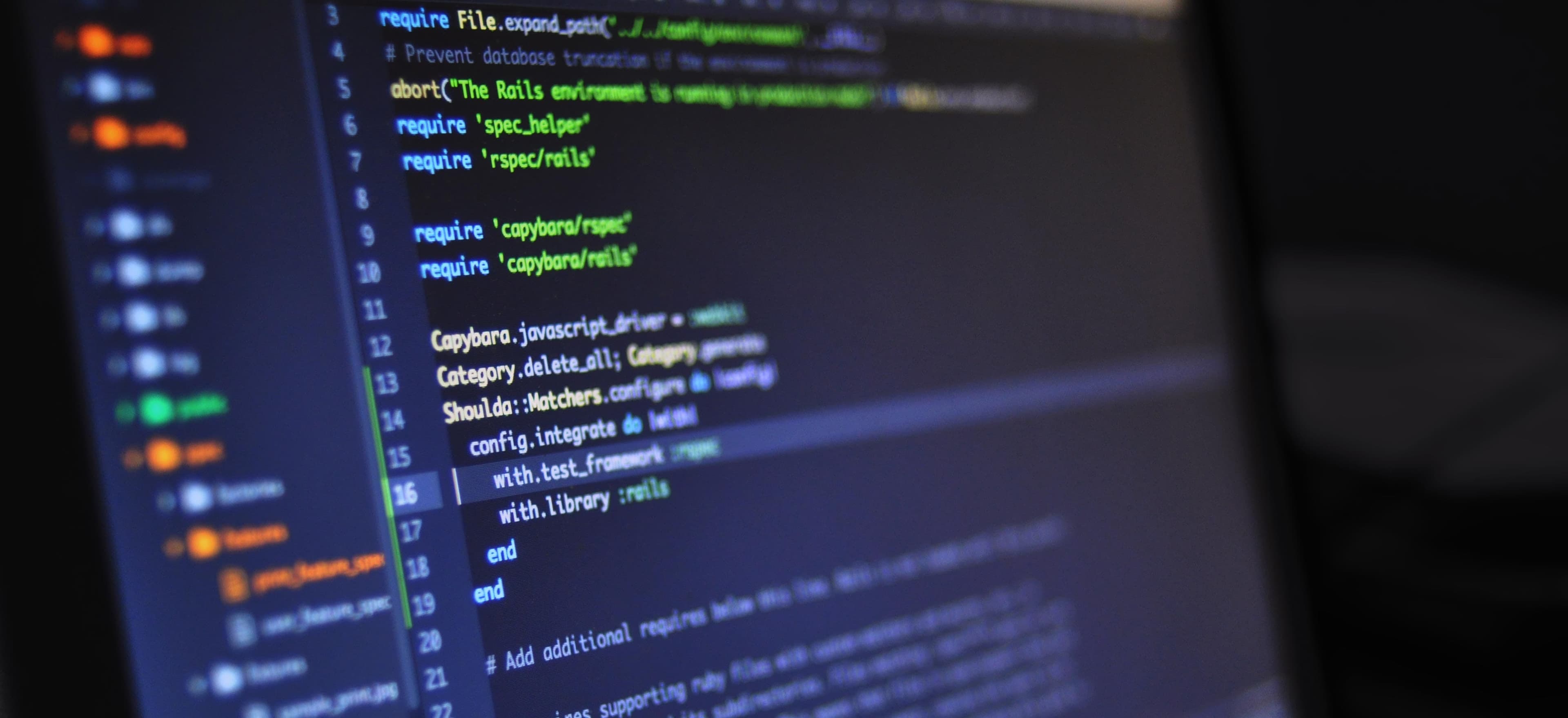
- Published on
Double Trouble: Our Hibernate JDBC Traffic Mishap Unveiled
If you're a Java developer working with databases, you’ve probably encountered the powerful and complex process of managing database communications. Hibernate, a popular object-relational mapping (ORM) framework for Java, is widely used to simplify this task. However, efficient database communication is crucial for the performance of your application. In this blog post, we'll delve into a common Java Hibernate issue related to JDBC traffic and how we can handle it effectively.
Understanding the Problem
When using Hibernate, one common issue is dealing with an excessive amount of JDBC traffic being generated. This can lead to decreased application performance, increased database load, and potential scalability challenges. This issue often arises due to Hibernate's default behavior of fetching data eagerly.
Eager fetching of data means that when an entity is retrieved from the database, its associated entities are also fetched immediately, which can result in unnecessary data retrieval and increased JDBC traffic. While eager fetching can be convenient, it can lead to performance bottlenecks, especially when dealing with large datasets and complex object relationships.
The Root of the Problem
Let's consider an example where we have two entities, Author
and Book
, with a one-to-many relationship, i.e., an author can have multiple books. When we retrieve an Author
using Hibernate, by default, it fetches all the associated Book
entities as well, leading to increased JDBC traffic.
@Entity
public class Author {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL)
private Set<Book> books = new HashSet<>();
// Getters and setters omitted for brevity
}
@Entity
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
@ManyToOne
@JoinColumn(name = "author_id")
private Author author;
// Getters and setters omitted for brevity
}
In this scenario, if we retrieve an Author
, Hibernate, by default, will also fetch all the associated Book
entities for that author, which could result in a substantial amount of unnecessary data retrieval.
Overcoming the Hibernate JDBC Traffic Mishap
1. Lazy Loading
To mitigate the excessive JDBC traffic caused by eager fetching, we can utilize the concept of lazy loading in Hibernate. Lazy loading defers the fetching of associated entities until they are explicitly accessed, thus reducing the unnecessary retrieval of data.
We can make use of the fetch
attribute in the @OneToMany
and @ManyToOne
annotations to specify lazy loading. By setting the fetch type to LAZY
, we instruct Hibernate to only fetch the associated entities when they are specifically accessed.
@OneToMany(mappedBy = "author", cascade = CascadeType.ALL, fetch = FetchType.LAZY)
private Set<Book> books = new HashSet<>();
With this modification, when an Author
entity is retrieved, the associated Book
entities will not be fetched immediately, resulting in reduced JDBC traffic.
2. Fetch Join
Another approach to address the excessive JDBC traffic issue is through the use of fetch joins. A fetch join is a specific type of join that allows us to fetch the associated entities in a single query, thereby reducing the number of database round-trips.
We can utilize the JOIN FETCH
clause in HQL (Hibernate Query Language) or JPQL (Java Persistence Query Language) to perform a fetch join. This instructs Hibernate to retrieve the associated entities eagerly in the initial query, avoiding the need for additional queries to fetch them later.
String query = "SELECT a FROM Author a JOIN FETCH a.books WHERE a.id = :authorId";
Author author = entityManager.createQuery(query, Author.class)
.setParameter("authorId", authorId)
.getSingleResult();
In this example, we use a fetch join to retrieve an Author
along with its associated Book
entities in a single query, optimizing the database communication and reducing JDBC traffic.
Wrapping Up
Hibernate, while providing powerful capabilities for database communication, can inadvertently lead to excessive JDBC traffic if not handled carefully. Understanding the concepts of lazy loading and fetch joins, and implementing them appropriately in your Hibernate mappings and queries, can significantly improve the performance and scalability of your application.
By addressing the JDBC traffic mishap through the effective use of lazy loading and fetch joins, you can streamline database communication, minimize unnecessary data retrieval, and enhance the overall efficiency of your Java Hibernate application.
In conclusion, by strategically managing JDBC traffic through proper data fetching strategies and query optimization, your Java Hibernate applications can achieve optimal performance and scalability while effectively interacting with the underlying database.
Remember, when it comes to database communication in your Hibernate-powered Java application, managing JDBC traffic effectively can make all the difference!
References:
- Hibernate Documentation
- Java Persistence API (JPA) Specification