Tackling Ineffective Exception Handling in NetBeans 7.4 Beta
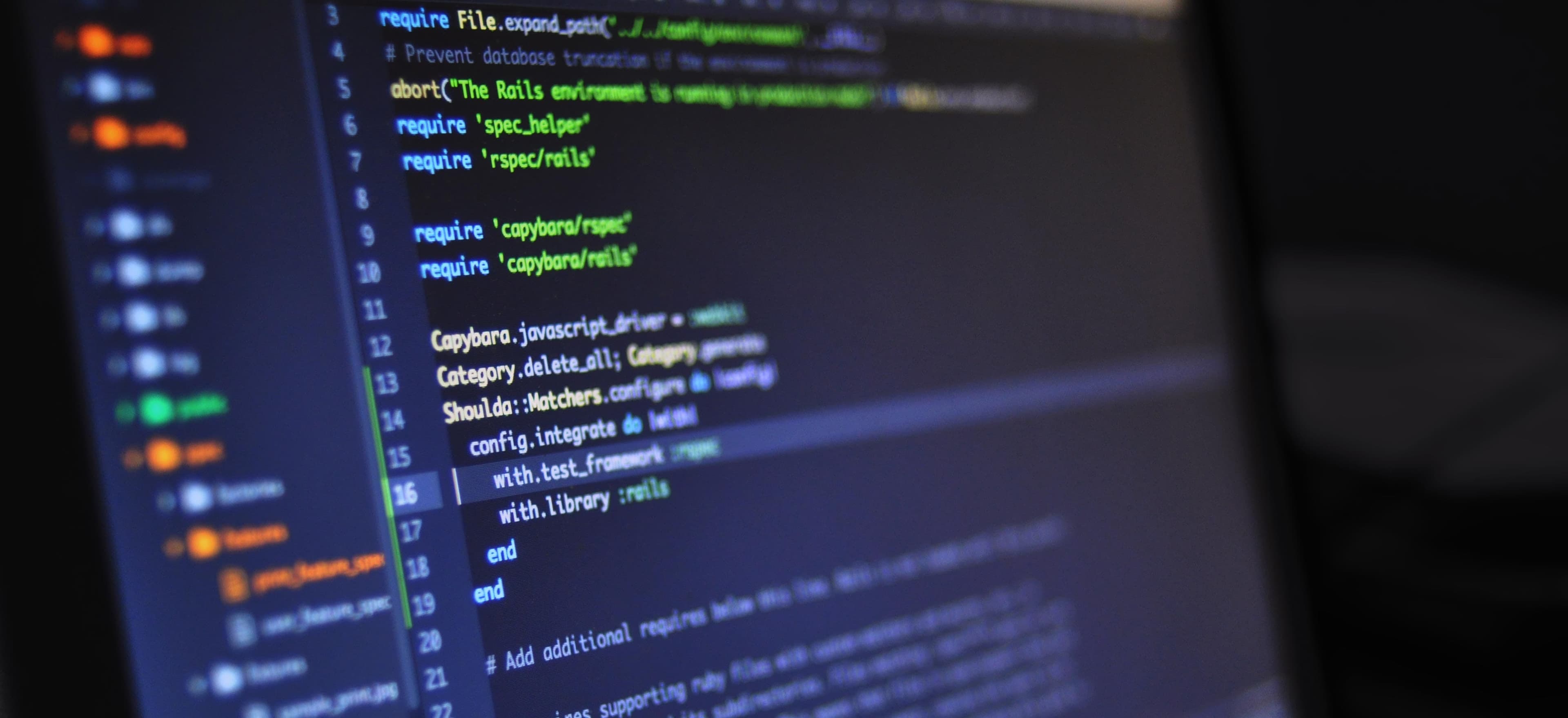
- Published on
Tackling Ineffective Exception Handling in NetBeans 7.4 Beta: A Comprehensive Guide
Beginning Insights
NetBeans 7.4 Beta holds a significant position in the Java ecosystem, serving as a powerful integrated development environment (IDE) for Java developers. However, a common challenge encountered by developers lies within the realm of exception handling. Inadequate or ineffective exception handling can lead to instability and unreliability in Java applications, ultimately impacting end-user experience.
Effective exception handling is crucial for maintaining the stability and resilience of Java applications. In this comprehensive guide, we will delve into the fundamentals of exception handling in Java, common mistakes to avoid, and how NetBeans 7.4 Beta provides features and tools to enhance exception handling. We will also explore best practices and strategies to improve exception handling in Java, empowering developers to write more robust and maintainable code.
Understanding Exceptions in Java
Exceptions play a pivotal role in Java programming, enabling developers to handle unexpected or erroneous occurrences during runtime. An exception represents an event that disrupts the normal flow of a program's execution. Java classifies exceptions into two categories: checked exceptions and unchecked exceptions. Checked exceptions must be either caught or declared to be thrown, while unchecked exceptions do not require such handling.
The try-catch-finally
block serves as the cornerstone of exception handling in Java. Within the try
block, code that may raise an exception is enclosed, and in the catch
block, specific exception types are caught and handled. The finally
block allows for the execution of code, regardless of whether an exception is thrown.
Code Snippet: Basic Exception Handling
try {
// Code that may raise an exception
FileReader file = new FileReader("file.txt");
// Additional code
} catch (FileNotFoundException e) {
// Handle the FileNotFoundException
System.out.println("File not found: " + e.getMessage());
} finally {
// Code that will always execute, like releasing resources
}
Common Mistakes in Exception Handling
Despite the fundamental nature of exception handling, developers often encounter a range of common mistakes when dealing with exceptions in Java applications. Some of these mistakes include swallowing exceptions, using generic catch
blocks, and not releasing resources properly.
For instance, swallowing exceptions occurs when a developer catches an exception but fails to handle or log it effectively, which can lead to silent failures and complicate debugging efforts. Similarly, using generic exception handling, such as catching Exception
instead of specific exception types, can obscure underlying issues and hinder effective diagnosis and resolution.
Code Snippet: Highlighting a Common Mistake
try {
// Code that may raise an exception
FileReader file = new FileReader("file.txt");
// Additional code
} catch (Exception e) {
// Swallowing the exception without proper handling or logging
}
Effective Exception Handling Strategies in NetBeans 7.4 Beta
NetBeans 7.4 Beta offers a range of features and tools to empower developers in addressing and resolving exceptions more effectively. Among these features, exception breakpoints and the "Surround with try-catch" refactoring tool are particularly valuable for pinpointing and managing exceptions efficiently.
Exception breakpoints allow developers to halt the program's execution when a specific exception is encountered during runtime, enabling them to inspect the state of the application at the point of exception occurrence. This real-time insight can significantly aid in diagnosing and addressing issues that lead to exceptions.
Code Snippet: Using Exception Breakpoints
In NetBeans 7.4 Beta, setting an exception breakpoint is simple. By following these steps, developers can leverage this feature to catch exceptions at runtime:
- Navigate to the "Breakpoints" tab in the NetBeans IDE.
- Click on "Add Breakpoint" and select "Exception Breakpoint."
- Specify the exception type and the class or method where the breakpoint should trigger.
- Run the program in debug mode to observe the exception being caught in real-time.
Best Practices for Exception Handling in Java
To ensure the reliability and maintainability of Java applications, adhering to best practices in exception handling is essential. One of the key aspects is the provision of meaningful exception messages, which facilitates effective troubleshooting and debugging. Additionally, creating custom exceptions for distinct error scenarios promotes better categorization and clarity in the handling of exceptional conditions.
Using logging frameworks to capture and record the occurrence of exceptions provides valuable insight into application behavior and potential issues. By incorporating these best practices, developers can enhance their code's maintainability and the overall resilience of their applications.
Code Snippet: Custom Exception and Logging
public class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
try {
// Code that may raise an exception
throw new CustomException("Custom exception message");
} catch (CustomException e) {
// Log the exception
LOGGER.error("Custom exception occurred: " + e.getMessage(), e);
}
Key Takeaways
In conclusion, effective exception handling is integral to the stability and reliability of Java applications. By understanding the foundational concepts of exception handling, recognizing common pitfalls, and leveraging the tools and features available in NetBeans 7.4 Beta, developers can elevate their ability to manage exceptions in their code. By adhering to best practices and embracing these strategies, developers can fortify their applications against unexpected events and empower themselves to build more resilient and maintainable Java software.
Additional Resources
For further exploration of exception handling in Java and leveraging NetBeans 7.4 Beta for efficient development, consider the following resources:
- Official NetBeans Documentation
- Comprehensive Guides on Java Exception Handling
- NetBeans Forums and Community Discussions
As you expand your knowledge in the realm of Java development and exception handling, these resources provide valuable insights and opportunities to engage with the wider developer community.
By leveraging the tools and knowledge presented in this guide, developers can enhance their proficiency in writing robust, stable, and resilient Java applications.
Remember, effective exception handling is not just about managing errors – it's about empowering your code to gracefully handle the unexpected, ensuring a seamless experience for end users.
Happy coding!