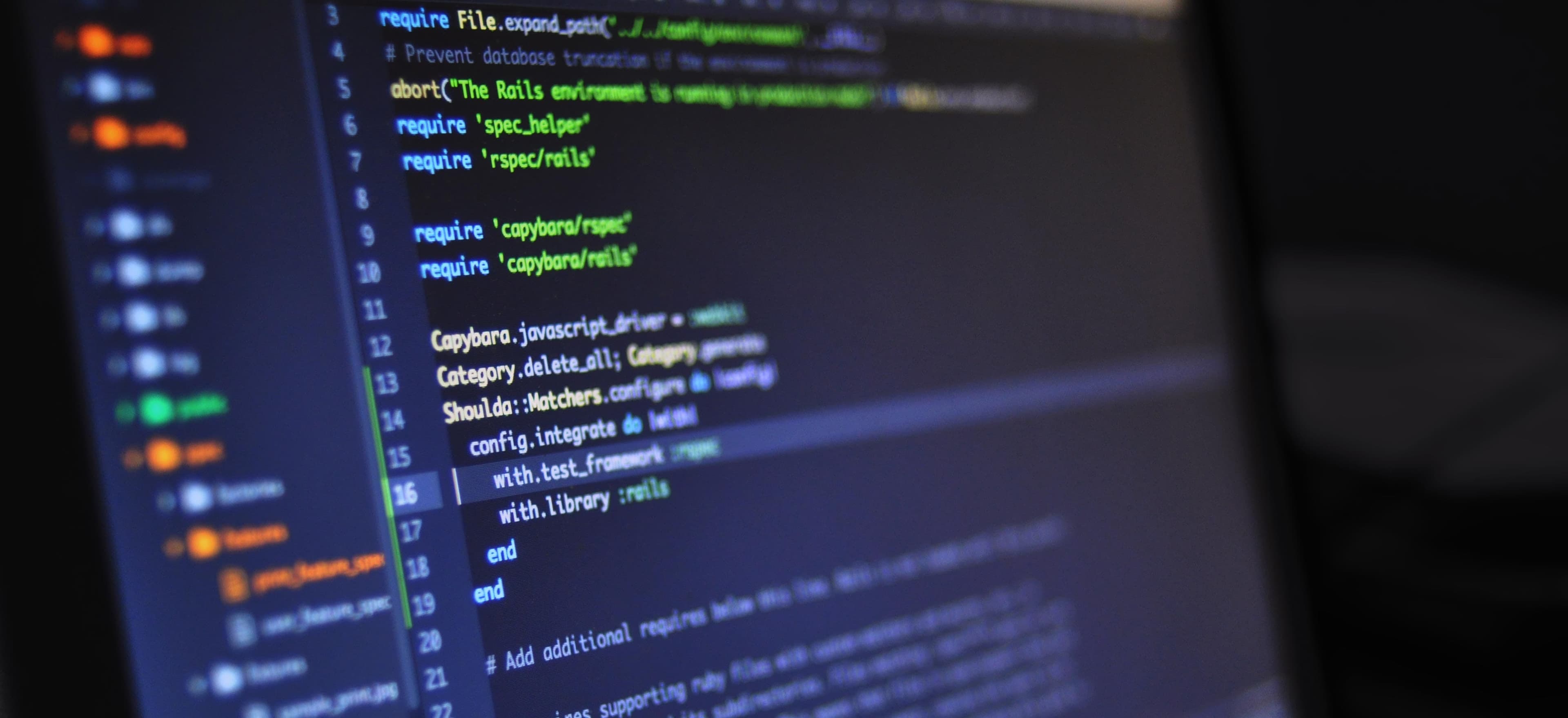
- Published on
Jersey 2 Meets Spring: Navigating Integration Hurdles
In the world of Java development, integrating different frameworks and libraries is a common challenge. When it comes to building RESTful web services, Jersey 2 and Spring are two popular choices. Jersey 2 is the reference implementation of JAX-RS (Java API for RESTful Web Services), while Spring is a powerful and widely used framework for building Java applications. In this blog post, we will explore the process of integrating Jersey 2 with Spring, highlighting the potential hurdles and providing solutions to ensure a smooth integration.
Why Integrate Jersey 2 with Spring?
Before delving into the integration process, let's briefly discuss why one might want to integrate Jersey 2 with Spring. Both Jersey 2 and Spring provide features for building RESTful web services, but there are scenarios where leveraging these two technologies together can be beneficial. For example, you may have an existing Spring-based application and want to expose certain functionality as RESTful services using Jersey 2. Integrating Jersey 2 with Spring allows you to harness the strengths of both frameworks, enabling seamless interaction between Spring components and JAX-RS resources.
Setting Up the Project
To begin the integration process, let's set up a new Maven project. Using Maven simplifies the management of dependencies and project structure. Here's the initial structure of our Maven project:
project-root/
├── src/
│ ├── main/
│ │ ├── java/
│ ├── resources/
├── pom.xml
We will add the necessary dependencies for Jersey 2 and Spring in the pom.xml
file. It's essential to ensure that versions of the dependencies are compatible to prevent potential compatibility issues.
Configuring Spring with Jersey 2
One of the primary steps in integrating Jersey 2 with Spring is to configure the Spring application context to include components and resources from Jersey 2. This can be achieved using the SpringBeanAutowiringSupport
class provided by Jersey 2. Let's create a configuration class to initialize the Spring context and register Jersey 2 resources:
import javax.ws.rs.ApplicationPath;
import org.glassfish.jersey.server.ResourceConfig;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.context.support.SpringBeanAutowiringSupport;
@ApplicationPath("/api")
@Configuration
public class JerseyConfig extends ResourceConfig {
public JerseyConfig() {
SpringBeanAutowiringSupport.processInjectionBasedOnCurrentContext(this);
packages("com.example.jersey2.resources");
}
}
In this configuration class, we are extending ResourceConfig
from Jersey 2 and using SpringBeanAutowiringSupport
to enable injection of Spring beans into Jersey 2 resources. The @ApplicationPath
annotation defines the base URI for the Jersey 2 resources. Additionally, we specify the package where our Jersey 2 resources are located using the packages
method.
Integrating Jersey 2 and Spring Components
To facilitate seamless integration between Jersey 2 and Spring components, we can utilize the @Component
annotation provided by Spring. This allows us to designate Spring-managed beans as Jersey 2 resources. For example, let's create a simple service class and expose it as a Jersey 2 resource:
import org.springframework.stereotype.Component;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.core.Response;
@Component
@Path("/hello")
public class HelloResource {
@GET
public Response getHelloMessage() {
String message = "Hello, Jersey 2 and Spring!";
return Response.ok(message).build();
}
}
In this example, we use the @Component
annotation to designate the HelloResource
class as a Spring-managed bean. Additionally, the @Path
annotation from Jersey 2 specifies the base URI for this resource, and the @GET
annotation denotes the HTTP GET method. This integration allows the HelloResource
to be managed by Spring and exposed as a Jersey 2 resource.
Resolving Dependency Injection Issues
When integrating Jersey 2 with Spring, it's crucial to address dependency injection issues to ensure that Spring-managed beans can be injected into Jersey 2 resources. One common hurdle is related to the inability to inject dependencies into constructors of Jersey 2 resources due to the way Jersey instantiates these resources.
To overcome this hurdle, we can utilize the @Context
annotation provided by Jersey 2 to inject dependencies into fields, setter methods, or resource methods. Here's an example of injecting a Spring-managed service into a Jersey 2 resource using the @Context
annotation:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class HelloService {
public String getMessage() {
return "Hello, Jersey 2 and Spring!";
}
}
@Path("/hello")
public class HelloResource {
@Autowired
private HelloService helloService;
@GET
public Response getHelloMessage() {
String message = helloService.getMessage();
return Response.ok(message).build();
}
}
In this example, we use the @Autowired
annotation to inject the HelloService
into the HelloResource
class. The @Context
annotation is not required in this scenario because we are leveraging Spring's dependency injection capabilities to inject the HelloService
.
Key Takeaways
Integrating Jersey 2 with Spring provides a powerful combination for building RESTful web services in Java. By configuring the Spring application context to include Jersey 2 components, leveraging Spring's dependency injection, and designating Spring-managed beans as Jersey 2 resources, developers can seamlessly integrate these two technologies to create robust and scalable applications.
With a well-structured project setup, thoughtful configuration, and understanding of dependency injection mechanisms, the hurdles of integrating Jersey 2 with Spring can be effectively navigated, leading to a harmonious fusion of these two powerful frameworks in the realm of Java web development.
In conclusion, the integration of Jersey 2 with Spring offers a potent synergy that empowers developers to build sophisticated and feature-rich RESTful web services with ease.
To delve deeper into the topics discussed in this article, check out Jersey 2 documentation and Spring documentation. These resources will provide additional insights and guidance for leveraging the capabilities of Jersey 2 and Spring in your Java projects.
Checkout our other articles