Streamline City Traffic Now: A Deep Dive into Microservices
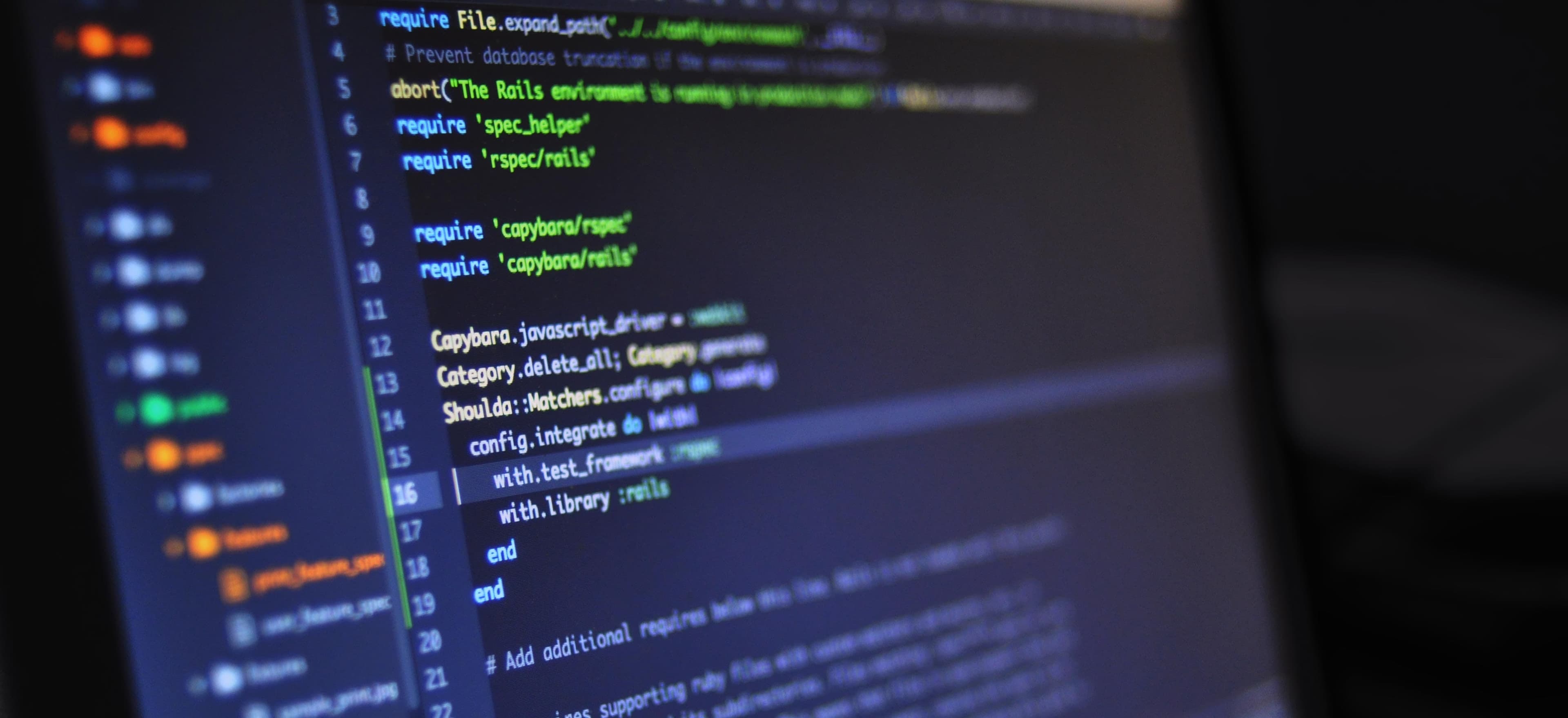
- Published on
Streamline City Traffic Now: A Deep Dive into Microservices
In today's fast-paced world, the concept of microservices has become a beacon of hope for developers looking to streamline operations, akin to traffic controllers aiming to optimize the flow of city traffic. Microservices, with their ability to break down complex applications into manageable, independently deployable services, offer a promising solution to the tangled web of monolithic architectures. This blog post takes you on a deep dive into the world of microservices, illustrating why and how they can streamline your development process just as effectively as traffic lights and roundabouts manage city traffic.
Understanding Microservices
Microservices architecture is a method of developing software applications as a collection of loosely coupled services, each responsible for a unique function and communicating over well-defined APIs. Imagine a bustling city: each district operates independently, but together they form a cohesive urban environment. This is the essence of microservices – a collection of independent services that, when combined, create a robust application.
Why adopt microservices? Here are a few compelling reasons:
- Scalability: Just as a city can grow by adding more districts, applications can scale by adding more services.
- Flexibility: Different teams can work on different services using the technologies best suited for each task.
- Resilience: If one service fails, like a traffic jam in one district, it doesn't cripple the entire application (or city).
Ready to dive into the code? Let’s demonstrate how to create a simple microservice using Java and Spring Boot, a popular framework for building microservices.
Building Your First Microservice with Java and Spring Boot
Spring Boot makes it easy to create stand-alone, production-grade Spring-based applications. Our goal is to build a microservice that manages user data – a critical component of our hypothetical ‘City Traffic’ application, aimed at streamlining traffic flows by providing real-time traffic updates to its users.
Prerequisites
- Java Development Kit (JDK) installed on your machine
- An Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse
- Familiarity with Maven or Gradle
Step 1: Setting Up Your Project
For a quick setup, visit Spring Initializr, a handy tool for bootstrapping your Spring Boot projects. Choose your preferred project metadata and add dependencies for Spring Web and Spring Data JPA.
Step 2: Writing the Code
Let’s create a simple UserController
that can handle HTTP requests to add and retrieve user data.
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@PostMapping("/")
public User addUser(@RequestBody User user) {
return userRepository.save(user);
}
@GetMapping("/")
public List<User> getAllUsers() {
return userRepository.findAll();
}
}
In this snippet, we define a REST controller with two endpoints. The addUser
method handles POST
requests to add a new user, and getAllUsers
handles GET
requests to retrieve all users.
Why use @Autowired
? It allows Spring to resolve and inject collaborating beans into our bean. In this case, UserRepository
is automatically instantiated and injected into UserController
.
Step 3: Running Your Application
To run your application, simply execute the main
method in the class annotated with @SpringBootApplication
. Spring Boot’s embedded server makes it seamless to launch your service without complex server configurations.
@SpringBootApplication
public class TrafficAppApplication {
public static void main(String[] args) {
SpringApplication.run(TrafficAppApplication.class, args);
}
}
Visit http://localhost:8080/users/
in your browser or use a tool like Postman to interact with your microservice.
Navigating the Challenges
Just like managing city traffic, deploying microservices comes with its own set of challenges: service discovery, inter-service communication, and data consistency, to name a few. Fortunately, solutions exist:
- Service Discovery: Tools like Netflix Eureka or Consul can help services find and communicate with each other.
- API Gateways: Tools such as Netflix Zuul provide a single entry point to your application, handling requests to the appropriate microservice.
- Distributed Transactions: Strategies like the saga pattern can ensure data consistency across services.
For a deeper understanding of these solutions, exploring official documentation or community guides is a recommended next step. Here are a couple of resources to get you started:
- Netflix Eureka: https://github.com/Netflix/eureka
- Spring Cloud Gateway: https://spring.io/projects/spring-cloud-gateway
To Wrap Things Up
Adopting a microservices architecture can significantly streamline the development and management of complex applications, much like how efficient traffic management systems streamline city traffic. By breaking down an application into smaller, manageable pieces, you can achieve better scalability, flexibility, and resilience.
Remember, transitioning to microservices requires careful planning and consideration of the associated challenges. However, with the right tools and strategies, microservices can revolutionize your development process, allowing you to deploy faster and adapt more quickly to changing requirements.
Ready to transform your development workflow and streamline your application architecture? Microservices might just be the green light you've been waiting for.
Checkout our other articles