Avoid the Pitfalls: Mastering Entity Services Implementation
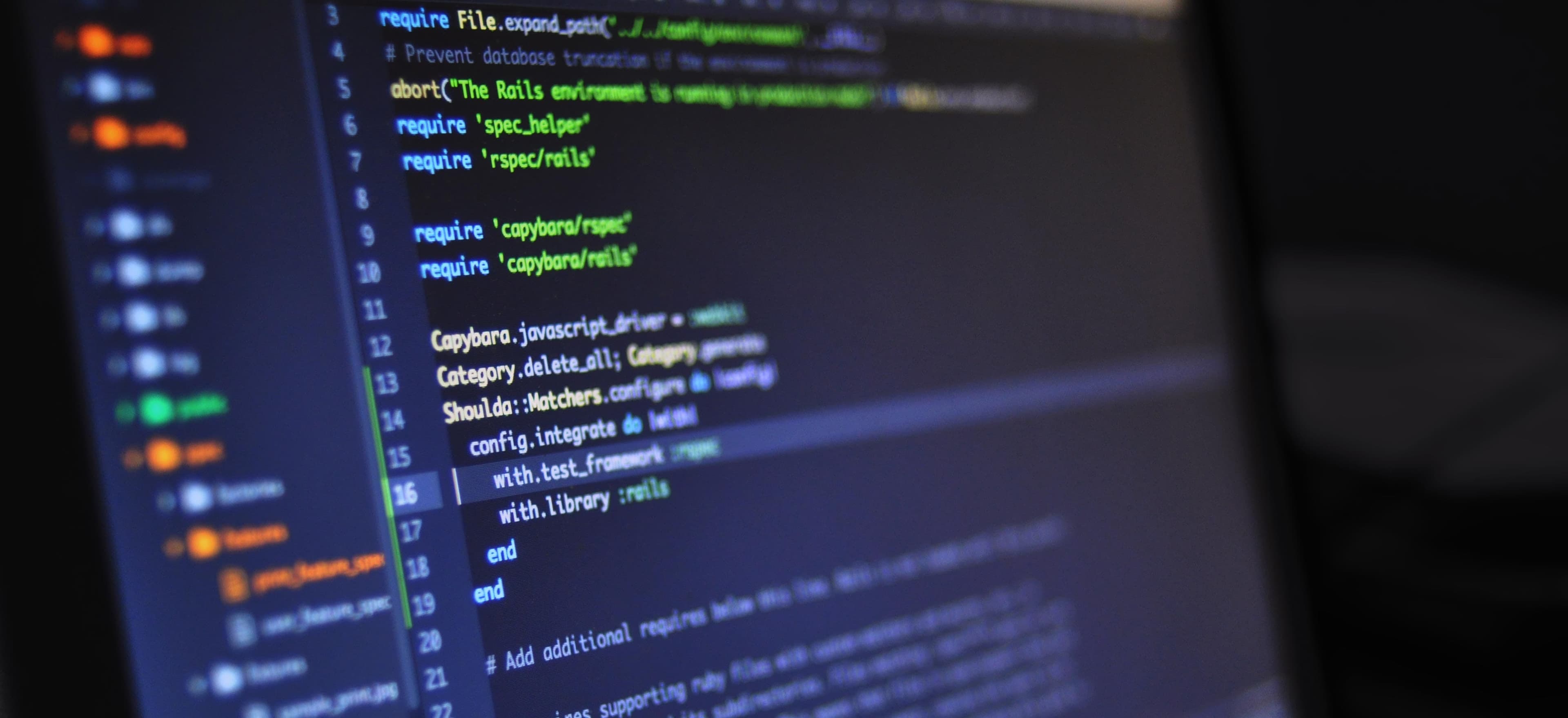
- Published on
Avoid the Pitfalls: Mastering Entity Services Implementation
In the world of enterprise applications, implementing entity services is a crucial aspect of maintaining and manipulating data. Java, being a versatile language, offers several ways to implement entity services, each with its own set of advantages and pitfalls. In this blog post, we will delve into the best practices for implementing entity services in Java, and highlight common pitfalls to avoid.
What are Entity Services?
Before we dive into the implementation details, let's clarify what entity services are. In a nutshell, entity services are responsible for providing operations and methods for interacting with data entities, such as databases, APIs, or any external data source. These services encapsulate the business logic associated with the entities and provide a clean interface for the rest of the application to interact with them.
Choosing the Right Design Pattern
When implementing entity services, it's crucial to choose the right design pattern to ensure maintainability, scalability, and testability. One of the most popular patterns for implementing entity services in Java is the Repository pattern.
The Repository Pattern
The Repository pattern abstracts the data access logic from the rest of the application, providing a clean separation between the data layer and the business logic. By utilizing interfaces and implementations, the Repository pattern allows for easy swapping of data sources and promotes testability by enabling the use of mock repositories in unit tests.
Let's take a look at a simple example of a UserRepository interface and its implementation using JPA (Java Persistence API):
public interface UserRepository {
User findById(Long id);
void save(User user);
void delete(User user);
}
public class JpaUserRepository implements UserRepository {
private EntityManager entityManager;
// Constructor injection of EntityManager
public User findById(Long id) {
return entityManager.find(User.class, id);
}
public void save(User user) {
entityManager.persist(user);
}
public void delete(User user) {
entityManager.remove(user);
}
}
By adhering to the Repository pattern, we can easily switch to a different data source, such as MongoDB or a web service, by implementing a new UserRepository without impacting the rest of the application.
Handling Entity Relationships
One of the common pitfalls when implementing entity services is managing entity relationships. It's crucial to carefully design and implement entity relationships to avoid performance issues and data inconsistencies. When working with JPA, understanding the FetchType and Cascade options is essential for effectively managing entity relationships.
FetchType and Cascade Options in JPA
The FetchType option in JPA determines whether the related entities should be loaded eagerly or lazily. Eager loading retrieves all related entities at the same time as the main entity, while lazy loading retrieves related entities only when accessed.
The choice between eager and lazy loading depends on the use case and the size of related data. Eager loading can lead to performance issues when dealing with large datasets, so it's essential to analyze the application's requirements carefully.
On the other hand, the Cascade option in JPA specifies the operations that should be cascaded to the related entities. These operations include persist, merge, remove, refresh, and detach. Careful consideration should be given to cascade options to avoid unintended side effects, especially when working with complex entity relationships.
Implementing Transaction Management
Another important aspect of entity services implementation is transaction management. Proper transaction management ensures data integrity and consistency, especially in multi-operation scenarios where multiple entities are involved.
Using @Transactional Annotation
In Java, Spring's @Transactional annotation provides a convenient way to manage transactions declaratively. By annotating the service or method with @Transactional, Spring handles the transaction boundaries, committing changes if the method completes successfully and rolling back if an exception occurs.
@Service
@Transactional
public class UserService {
private UserRepository userRepository;
// Constructor injection of UserRepository
public void updateUserAndLog(User user, String logMessage) {
userRepository.save(user);
logService.log(logMessage);
}
}
By leveraging the @Transactional annotation, we delegate the transaction management to Spring, reducing the boilerplate code and ensuring consistent transaction behavior across the application.
Handling Performance Considerations
Efficient handling of performance considerations is crucial for entity services, especially when dealing with large datasets or complex queries. Leveraging caching mechanisms, optimizing database queries, and utilizing appropriate indexing are essential for achieving optimal performance.
Utilizing Hibernate Caching
When working with JPA and Hibernate, caching can significantly improve the performance of read-heavy applications. Hibernate provides first and second-level caching mechanisms that help reduce the number of database round trips by caching entity data in memory.
By enabling and tuning the appropriate level of caching, we can minimize the database load and improve the overall responsiveness of the application. However, it's essential to be cautious with caching, as it can lead to stale data and increased memory consumption if not managed properly.
Testing Entity Services
Testing entity services is crucial to ensure their correctness and reliability. Unit tests, integration tests, and mocking frameworks play a vital role in validating the behavior of entity services and detecting potential issues early in the development cycle.
Using JUnit and Mockito for Testing
JUnit and Mockito are popular testing frameworks in the Java ecosystem that facilitate unit testing and mocking of dependencies. By writing comprehensive unit tests for entity services, we can validate their functionalities in isolation and identify any logic errors or integration issues.
@RunWith(MockitoJUnitRunner.class)
public class UserRepositoryTest {
@Mock
private EntityManager entityManager;
@InjectMocks
private JpaUserRepository userRepository;
@Test
public void testFindById() {
// Mockito setup and assertions
}
@Test
public void testSave() {
// Mockito setup and assertions
}
@Test
public void testDelete() {
// Mockito setup and assertions
}
}
Mockito allows us to mock the EntityManager and its behavior, enabling us to focus solely on testing the UserRepository functionality without relying on a real database connection.
A Final Look
Mastering entity services implementation in Java requires a deep understanding of design patterns, entity relationships, transaction management, performance considerations, and testing strategies. By choosing the right design pattern, handling entity relationships carefully, implementing transaction management effectively, considering performance implications, and thorough testing, developers can steer clear of common pitfalls and ensure robust and efficient entity services.
To further enhance your understanding of entity services and Java best practices, consider exploring the Java Persistence API (JPA) and Spring Framework documentation.
By following these best practices and staying vigilant against potential pitfalls, developers can create scalable, maintainable, and high-performing entity services that form the backbone of robust enterprise applications.
Checkout our other articles