Bridging the Gap: Integrating Architecture in DevOps
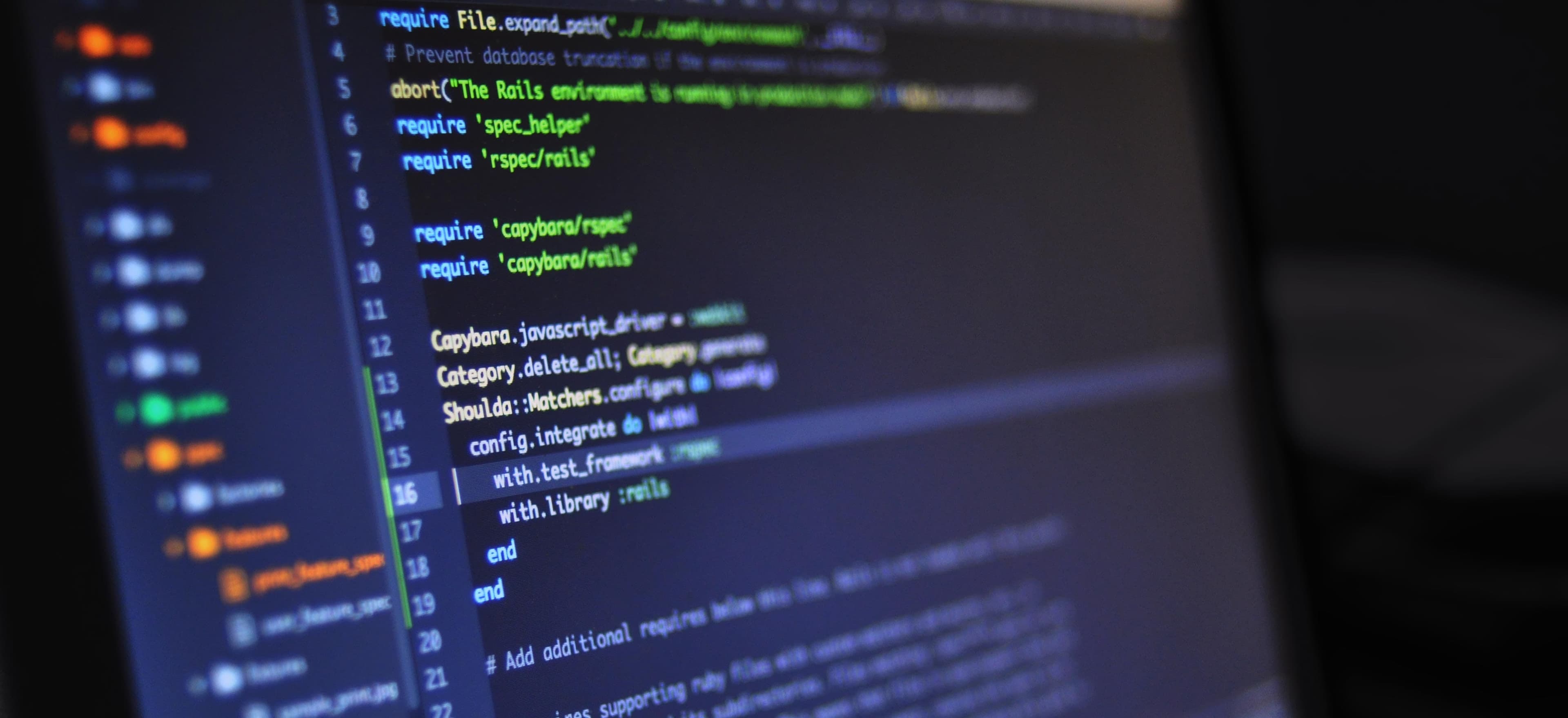
- Published on
Bridging the Gap: Integrating Architecture in DevOps
In the fast-paced world of software development, the integration of architecture in DevOps has become increasingly crucial. While DevOps focuses on the collaboration between development and operations teams, integrating architecture ensures that the software's design aligns with the organization's goals and long-term vision. In this blog post, we will explore the significance of incorporating architecture in DevOps, discuss best practices, and showcase how Java, with its robust features and tools, can facilitate this integration.
Understanding the Importance
Enhancing System Resilience and Scalability
Integrating architecture in DevOps ensures that the software systems are designed with scalability and resilience in mind. By considering architectural principles early in the development lifecycle, teams can make informed decisions about technology stack, infrastructure, and design patterns, leading to more reliable and scalable systems.
Aligning Development with Business Objectives
Architectural decisions heavily influence the long-term maintainability and evolution of software systems. Integrating architecture in DevOps allows teams to align their development efforts with the organization's business objectives, ensuring that the software not only meets immediate needs but also supports future growth and adaptability.
Facilitating Cross-Functional Collaboration
By bringing architects into the DevOps fold, teams can benefit from their expertise in design and system-wide considerations. This collaborative approach ensures that architectural decisions are not made in isolation but in conjunction with development, operations, and other relevant stakeholders, leading to holistic and well-informed design choices.
Best Practices for Integrating Architecture in DevOps
Continuous Architectural Refactoring
Just as code undergoes continuous refactoring in DevOps, architecture should also be subject to ongoing evaluation and improvement. This practice involves re-evaluating architectural decisions, identifying areas for enhancement, and iteratively refining the system's design to meet evolving requirements.
// Example of continuous architectural refactoring in Java
public class UserService {
private UserRepository userRepository;
// Constructor injection for flexibility and testability
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Method demonstrating the use of a well-defined architectural pattern (such as repository pattern) for data access
public User getUserById(long userId) {
return userRepository.findById(userId);
}
}
In the above example, the UserService
class follows the repository pattern, which is a well-defined architectural pattern for data access. This ensures separation of concerns and facilitates maintainability.
Automated Architecture Validation
Integrating automated architecture validation into the CI/CD pipeline can help detect architectural violations early in the development process. Tools such as Checkstyle and SonarQube for Java can be leveraged to enforce architectural conformance and identify potential design flaws, ensuring that the codebase adheres to the defined architectural guidelines.
Empowering Teams with Architecture-as-Code
Just as infrastructure is managed as code in DevOps, architectural components can also be represented as code. By utilizing tools like ArchUnit for Java, teams can write automated tests to validate architectural rules and constraints, treating architecture as first-class citizen in the codebase.
// Example of using ArchUnit for architectural validation in Java
@AnalyzeClasses(packages = "com.example.myapp")
public class MyArchitectureTest {
@ArchTest
public static final ArchRule services_should_only_be_accessed_by_controllers =
classes().that().resideInAPackage("..service..").should().onlyBeAccessed().byAnyPackage("..controller..", "..service..");
}
The above ArchUnit test ensures that services are only accessed by controllers or other services, enforcing a clear architectural boundary.
Leveraging Java for Integrating Architecture in DevOps
Strong Typing and Design Patterns
Java's strong typing and support for design patterns make it well-suited for enforcing architectural principles. By leveraging interfaces, abstract classes, and design patterns such as MVC, DI, and AOP, developers can build scalable and maintainable architectures that align with the organization's requirements.
Ecosystem for Automated Testing
Java's rich ecosystem for automated testing, including JUnit and Mockito, empowers teams to validate architectural decisions through comprehensive unit, integration, and mock testing. This ensures that the architectural components not only meet functional requirements but also adhere to non-functional aspects such as performance, scalability, and maintainability.
Tooling Support for Continuous Integration
Java's extensive tooling support, including Maven and Gradle for build automation, Jenkins and TeamCity for CI/CD, and Docker for containerization, enables seamless integration of architecture-centric processes into the DevOps pipeline. This allows teams to automate architectural validation, promote architectural consistency, and ensure that architectural decisions are reflected in the deployed systems.
My Closing Thoughts on the Matter
Integrating architecture in DevOps is essential for building resilient, scalable, and future-proof software systems. By following best practices such as continuous architectural refactoring, automated validation, and treating architecture as code, teams can ensure that architectural concerns are given the attention they deserve within the DevOps paradigm. Leveraging Java's strong features and tooling support further facilitates the seamless integration of architecture in the DevOps lifecycle, empowering teams to design and deliver robust software solutions that align with the organization's strategic goals.
In conclusion, the marriage of architecture and DevOps in the realm of Java development sets the stage for the creation of software systems that not only meet immediate needs but also withstand the test of time, evolving alongside the organization's objectives and technological advancements.
By incorporating architectural considerations within the DevOps process, teams can foster a culture of collaboration, innovation, and long-term value creation, ultimately bridging the gap between architectural design and operational excellence.
Integrating architecture in DevOps is not just a technical endeavor; it is a strategic investment in the organization's future success.
References:
So, what are your thoughts on this? How do you think integrating architecture in DevOps can further enhance software development practices?
Checkout our other articles