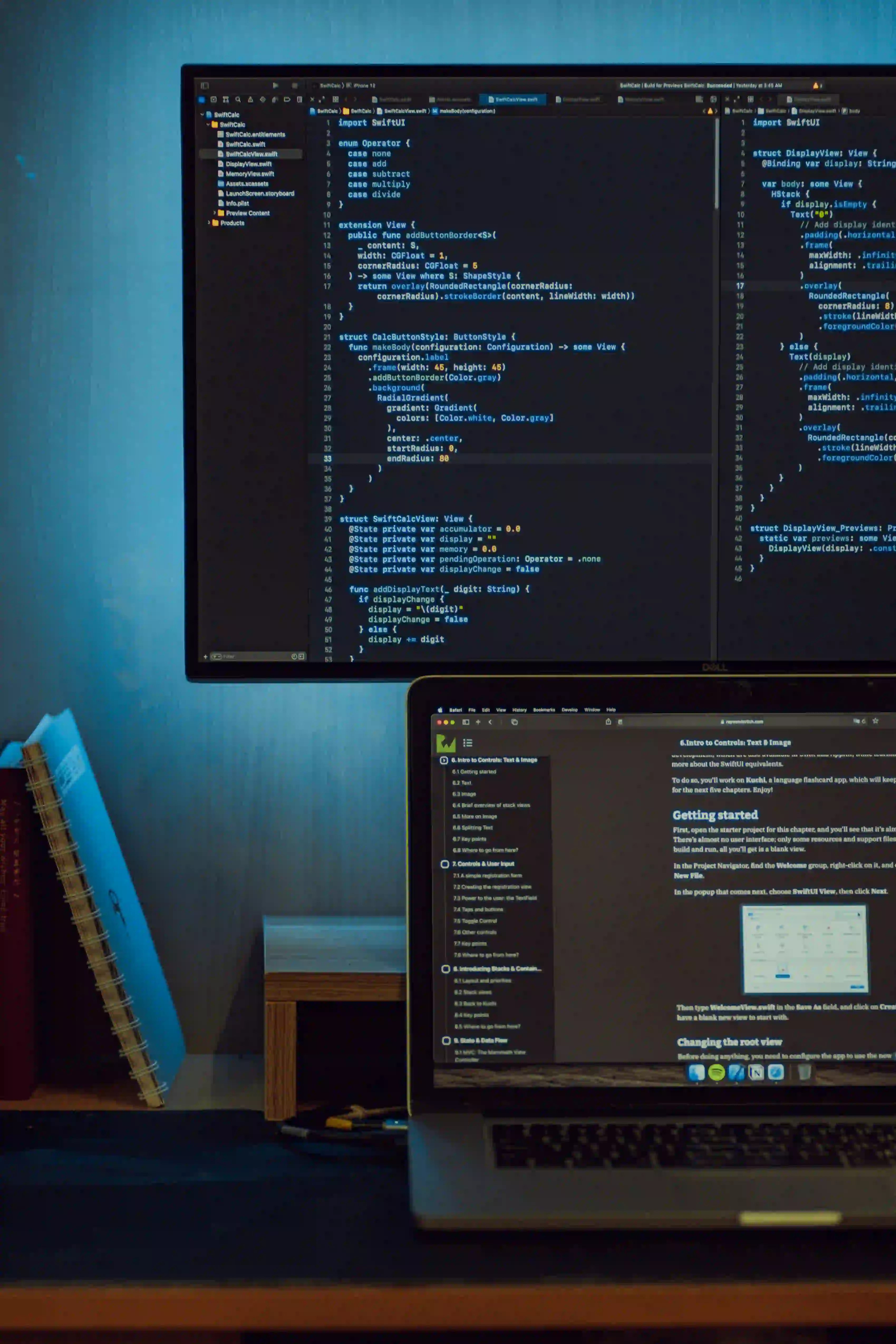
Unlocking Touch Magic: Mastering Gestures in Android Apps
If you're an Android developer, you know just how important touch gestures are in creating intuitive and seamless user experiences. From swiping and pinching to tapping and dragging, gestures are fundamental to modern mobile app design. In this blog post, we'll delve into the world of touch gestures in Android apps, exploring their significance, implementation, and best practices.
The Significance of Touch Gestures
Touch gestures are more than just a fancy way for users to interact with apps – they form the cornerstone of user experience design. Gestures provide a natural and intuitive way for users to navigate and interact with content, making apps more engaging and accessible. Whether it's zooming in on an image, scrolling through a feed, or flipping through pages, gestures enrich the user experience and set a high bar for app usability.
Implementing Touch Gestures in Android
Android provides a comprehensive framework for capturing and handling touch gestures. The GestureDetector
class, part of the Android SDK, is a powerful tool for detecting common gestures such as taps, swipes, and scrolls. Additionally, the GestureOverlayView
allows for the creation of custom gestures that can be recognized within an app.
Let's take a look at a simple implementation of a swipe gesture using GestureDetector
:
public class MainActivity extends AppCompatActivity implements GestureDetector.OnGestureListener {
private GestureDetectorCompat gestureDetector;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
gestureDetector = new GestureDetectorCompat(this, this);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
gestureDetector.onTouchEvent(event);
return super.onTouchEvent(event);
}
@Override
public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX, float velocityY) {
// Handle the swipe gesture
return true;
}
// Other overridden methods from GestureDetector.OnGestureListener
}
In this example, we create a GestureDetector
instance and override the onTouchEvent
method to pass touch events to the detector. We then implement the onFling
method to handle the swipe gesture. This is a basic example, and there are many more gestures and customization options available.
Best Practices for Touch Gesture Implementation
While implementing touch gestures in Android apps, it's crucial to adhere to best practices to ensure a seamless and intuitive user experience. Here are some key principles to keep in mind:
1. Feedback
Provide visual or haptic feedback to indicate when a gesture has been recognized. This reassures users that their actions have been registered, enhancing the overall user experience.
2. Customization
Allow users to customize certain gestures to better suit their preferences. For example, letting users define their own swipe actions for specific app features can greatly enhance usability.
3. Compatibility
Ensure that touch gestures are compatible with a wide range of devices, screen sizes, and orientations. A gesture that works well on a large tablet might need to be adapted for use on a smaller smartphone screen.
4. Accessibility
Always consider the accessibility implications of touch gestures. Provide alternative methods for performing crucial actions for users who may have difficulty with gestures.
5. Performance
Efficiently handle gesture detection and processing to prevent lag or unresponsiveness. Optimizing gesture recognition plays a key role in delivering a smooth user experience.
By keeping these best practices in mind, developers can create apps with touch gestures that feel natural and intuitive, elevating the overall user experience.
The Closing Argument
In the realm of Android app development, mastering touch gestures is essential for creating user-friendly and immersive experiences. By leveraging the Android framework's gesture detection capabilities and adhering to best practices, developers can unlock the full potential of touch magic in their apps. Embracing the art of touch gestures not only enhances usability but also sets the stage for innovative and engaging app interactions.
To further enhance your understanding of touch gestures in Android apps, be sure to check out the official Android Developer documentation and explore the various gesture-related APIs and resources available.
Happy coding and may your apps be touch magic maestros!