Boost Your Speed: Perfecting Parallel Processing in Containers
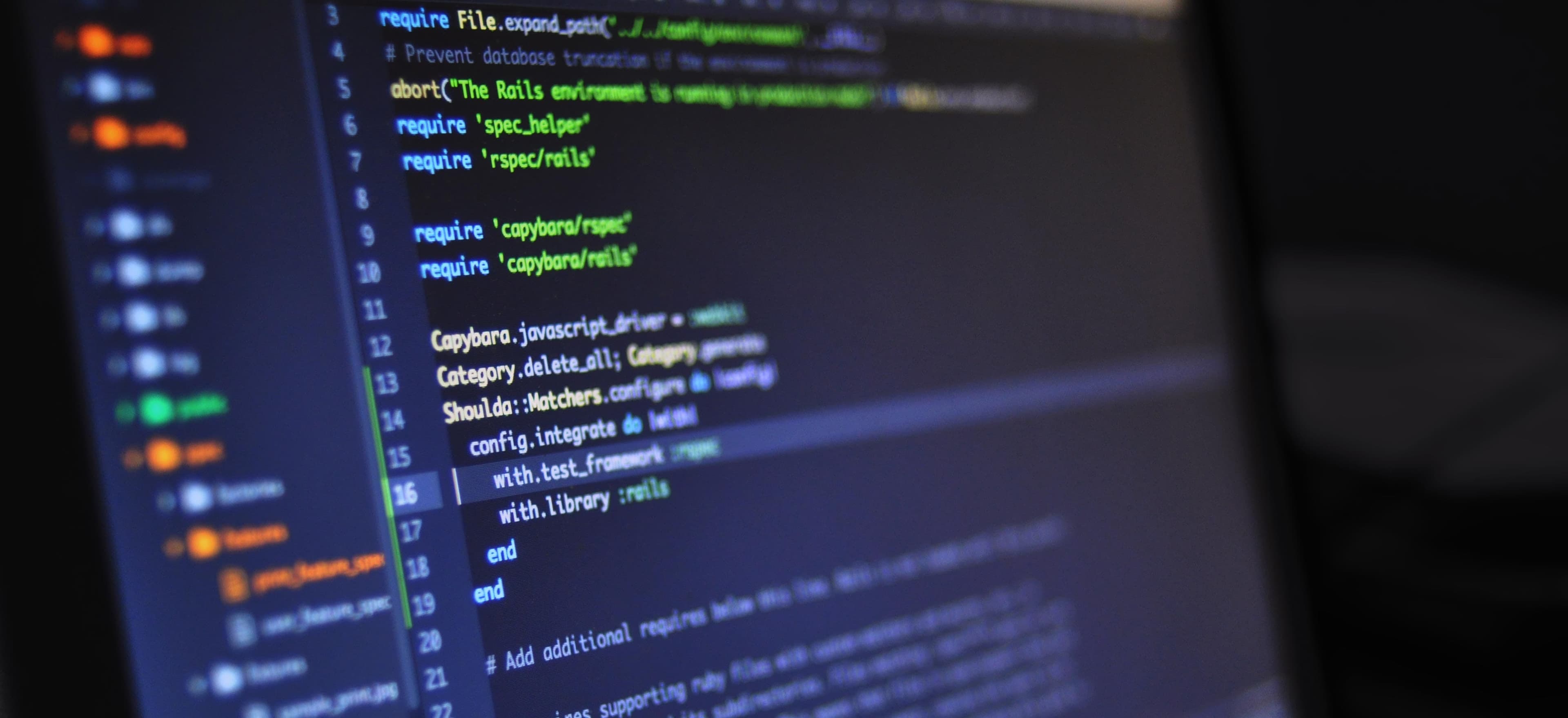
- Published on
Boost Your Speed: Perfecting Parallel Processing in Containers with Java
In the rapidly evolving landscape of software development, speed and efficiency are the currency of the digital age. As developers, we constantly seek ways to shave milliseconds off execution times, and one potent technique at our disposal is parallel processing. When combined with the scalability and isolation benefits of containers, parallel processing becomes a powerhouse. In this comprehensive guide, we'll delve into perfecting parallel processing in containers using Java—a language renowned for its robustness and flexibility.
What is Parallel Processing?
Parallel processing involves dividing a program into independent parts that can run simultaneously, usually with the goal of completing tasks faster than would be possible sequentially. In the context of Java, this often involves utilizing threads or the ForkJoinPool, which efficiently manages worker threads for you.
Why Containers?
Containers, such as those orchestrated by Docker or Kubernetes, encapsulate your application along with its environment, ensuring consistency across development, testing, and production. They shine in scalability, allowing for easy replication of services to handle increased loads.
Embracing Parallel Processing in Java within Containers
Leveraging parallel processing in Java applications that run within containers involves understanding both the Java concurrency framework and container resource management. Let's explore how to marry these two for optimized performance.
Java Concurrency Tools for Parallel Processing
Java offers a rich set of concurrency tools under the java.util.concurrent
package, which can significantly simplify the implementation of parallel processing in your application. The ForkJoinPool
and streams API are particularly well-suited for such tasks.
Example: Utilizing ForkJoinPool for Recursive Tasks
The ForkJoinPool
is an ideal match for problems that can be broken down into smaller tasks and executed in parallel. Below is a simplified example of its use:
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.RecursiveTask;
class FibonacciCalculator extends RecursiveTask<Integer> {
final int n;
FibonacciCalculator(int n) { this.n = n; }
@Override
protected Integer compute() {
if (n <= 1)
return n;
FibonacciCalculator f1 = new FibonacciCalculator(n - 1);
f1.fork(); // asynchronously execute this task in the ForkJoinPool
FibonacciCalculator f2 = new FibonacciCalculator(n - 2);
return f2.compute() + f1.join(); // waits for and retrieves the result of f1
}
}
public class ParallelFibonacci {
public static void main(String[] args) {
ForkJoinPool pool = new ForkJoinPool();
FibonacciCalculator task = new FibonacciCalculator(10);
int result = pool.invoke(task);
System.out.println("Fibonacci number: " + result);
}
}
Why this approach? The ForkJoinPool
efficiently manages a pool of worker threads, dynamically adjusting the number of threads to optimize throughput. This example demonstrates breaking down the recursive calculation of Fibonacci numbers into smaller chunks that are executed in parallel.
Optimizing Container Configuration for Parallel Processing
To ensure that your Java application running in a container can make the most of parallel processing, you need to tune your container's configuration. This includes setting appropriate CPU and memory limits to ensure that the container has enough resources to perform parallel execution effectively.
Here are some tips:
-
Define resource limits and requests in your container orchestration tool (e.g., Kubernetes). This ensures your Java application has the resources it needs without hogging the host system.
-
Leverage multi-stage Docker builds to keep your container images lean, ensuring faster startup times and more efficient scaling.
For more insights into container configuration and management, the official Kubernetes documentation is a goldmine of information: Understanding Kubernetes Resource Management.
Writing Efficient Java Code for Parallel Execution
Writing Java code that executes efficiently in parallel requires attention to detail. Here are a few tips:
-
Avoid shared mutable states: Access to shared mutable variables from multiple threads can lead to contention and performance bottlenecks. Use local variables, thread confinement, and immutable objects where possible.
-
Minimize synchronization: Excessive synchronization can negate the benefits of parallelism. Make use of concurrent data structures and atomic variables offered by
java.util.concurrent
. -
Profile and monitor your application: Tools like VisualVM provide insights into thread behavior, helping you identify and rectify performance issues.
For more advanced profiling, consider using tools like YourKit or JProfiler.
Best Practices for Containerized Java Applications
Beyond just parallel processing, here are some best practices when running Java applications in containers:
-
Use the JVM’s container awareness features: Modern JVMs can detect when they’re running in a container and adjust memory limits accordingly. Ensure your JVM flags are set to leverage this (e.g.,
-XX:+UseContainerSupport
). -
Implement health checks and graceful shutdown: To improve resilience and ensure smooth scaling, implement health checks and handle SIGTERM signals for graceful shutdown.
Embracing the Future
Parallel processing in containers represents a convergence of efficiency and scalability, perfectly suited for the demands of modern software development. By understanding the intricacies of Java's concurrency tools and fine-tuning your container configurations, you can unleash the full potential of your applications.
For further reading and resources on Java concurrency, the official Oracle tutorials are an excellent starting point: Java Concurrency.
As the digital landscape continues to evolve, staying abreast of these techniques will ensure your applications remain performant and competitive. Dive in, experiment, and watch your Java applications soar to new heights of efficiency and scalability.