Fixing Java Client Connectivity Issues with Elasticsearch
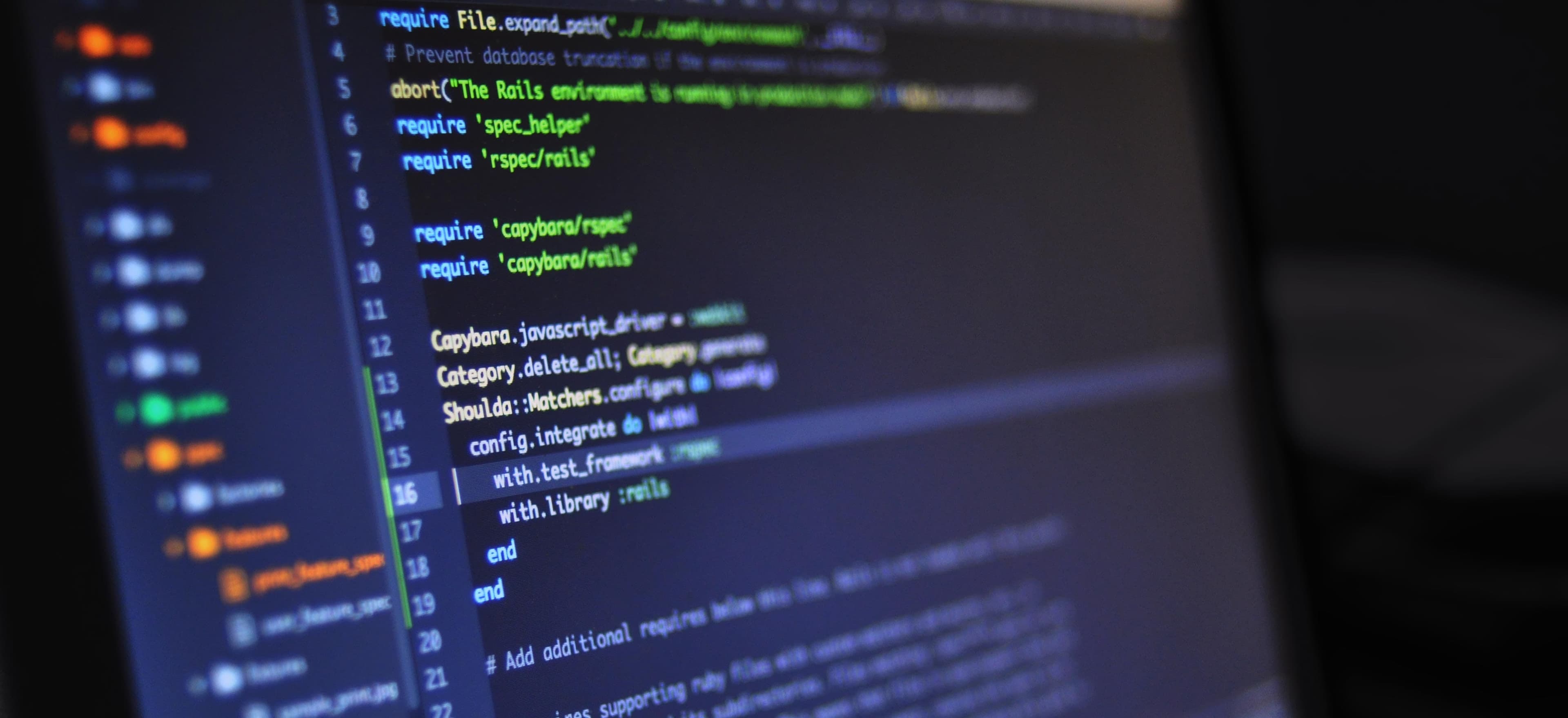
- Published on
Understanding Java Client Connectivity Issues with Elasticsearch
Java has become one of the most popular programming languages for developing enterprise applications, which often require seamless integration with various data storage systems. Among these, Elasticsearch has gained widespread adoption as a powerful and efficient search engine and analytics tool. However, while working with Java clients for Elasticsearch, developers often encounter connectivity issues that can be quite frustrating to debug and resolve. In this article, we'll explore common Java client connectivity issues with Elasticsearch and how to address them effectively.
Connecting with the Elasticsearch Java High-Level REST Client
The Elasticsearch Java High-Level REST Client offers a convenient way to interact with Elasticsearch from a Java application. However, establishing a connection with an Elasticsearch cluster using this client can sometimes pose challenges.
One common issue is encountering connection timeouts during the initialization of the client. This typically happens when the client cannot establish a connection within the specified timeout duration. To address this, it's crucial to review the network connectivity between the Java application and the Elasticsearch cluster. Furthermore, ensuring that the cluster is accessible from the network and checking firewall settings can also be beneficial.
Depicting the Connection Timeout Issue
Consider the following excerpt which demonstrates the initialization of the High-Level REST Client in Java:
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(
new HttpHost("localhost", 9200, "http"),
new HttpHost("localhost", 9201, "http")));
While this code may seem unproblematic, connectivity problems can arise due to network configurations, cluster unavailability, or SSL-related issues.
A potential solution involves modifying the client initialization to include timeout settings:
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(
new HttpHost("localhost", 9200, "http"),
new HttpHost("localhost", 9201, "http"))
.setRequestConfigCallback(
requestConfigBuilder -> requestConfigBuilder
.setConnectTimeout(5000)
.setSocketTimeout(60000)));
In this context, setting the connection and socket timeouts ensures that the client doesn't wait indefinitely, enhancing the handling of connectivity issues.
Resolving SSL Handshake Failures
Another common connectivity issue is encountering SSL handshake failures. When configuring the Java client to connect to an Elasticsearch cluster secured with SSL/TLS, it's essential to handle SSL-related errors gracefully.
To elaborate, a typical SSL-related error might manifest as follows:
javax.net.ssl.SSLHandshakeException: PKIX path building failed
This exception signifies a failure in building the certification path due to missing or untrusted certificates.
In order to circumvent SSL handshake failures, it's paramount to ensure that the Java client trusts the certificates presented by the Elasticsearch cluster. This can be achieved by importing the cluster's SSL certificate into the Java keystore used by the client application.
Let's delve into a sample code snippet that demonstrates importing the SSL certificate into the Java keystore:
String elasticsearchHost = "example.com";
int elasticsearchPort = 9200;
System.setProperty("javax.net.ssl.trustStore", "/path/to/truststore.jks");
System.setProperty("javax.net.ssl.trustStorePassword", "yourpassword");
TrustManager[] trustAllCertificates = new TrustManager[]{
new X509TrustManager() {
public X509Certificate[] getAcceptedIssuers() {
return null;
}
public void checkClientTrusted(X509Certificate[] certs, String authType) {
}
public void checkServerTrusted(X509Certificate[] certs, String authType) {
}
}
};
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, trustAllCertificates, new java.security.SecureRandom());
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(
new HttpHost(elasticsearchHost, elasticsearchPort, "https"))
.setHttpClientConfigCallback(httpClientBuilder ->
httpClientBuilder.setSSLContext(sslContext)));
In this code, the SSL certificate is imported into the truststore, ensuring that the Java client can establish a secure connection with the Elasticsearch cluster.
The Bottom Line
In conclusion, Java clients for Elasticsearch can encounter various connectivity issues, including connection timeouts and SSL handshake failures. By identifying and addressing these issues through appropriate timeout settings and SSL certificate management, developers can mitigate connectivity challenges effectively. It's essential to comprehend the intricacies of these issues and employ robust solutions to ensure seamless connectivity between Java applications and Elasticsearch clusters.