Making Acceptance Criteria Executable: A How-To Guide
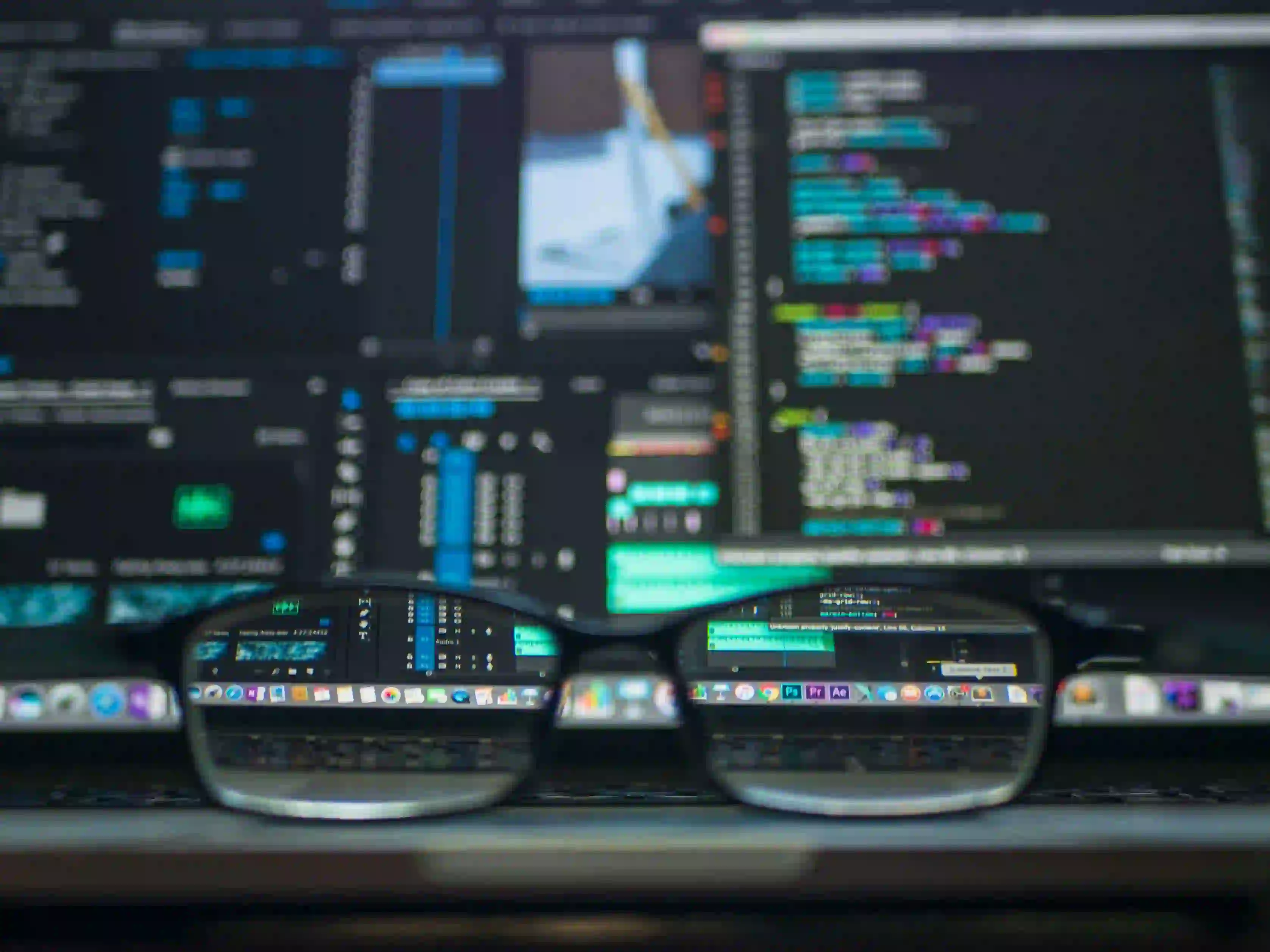
Making Acceptance Criteria Executable: A How-To Guide
Let us Begin
In the realm of software development, acceptance criteria serve as a vital link between business requirements and the technical implementation of features. They provide a clear definition of what the development team needs to achieve and what the stakeholders can expect from the final product. However, acceptance criteria need to be more than just a set of guidelines; they should be executable, meaning that they should be automated and verifiable to ensure that the developed features meet the specified requirements accurately. This article will delve into the concept of making acceptance criteria executable and provide a comprehensive guide on how to achieve this in the context of Java-based development.
Keywords: Acceptance Criteria, Executable, Software Development, Java, Testing
What are Acceptance Criteria?
Acceptance criteria are a set of conditions or specifications that a software product must meet in order to be accepted by a user, customer, or other authorized entity. In Agile development methodologies, acceptance criteria play a crucial role in ensuring that the product meets the needs and expectations of the end-users. They provide clear guidelines for the development team and help in defining and verifying the scope of work.
Examples of Acceptance Criteria
-
Example 1:
- Feature: User Profile Management
- Acceptance Criteria:
- A user should be able to create a new profile with a valid email address and password.
- The profile should display the user's name, contact information, and recent activity.
-
Example 2:
- Feature: E-commerce Checkout Process
- Acceptance Criteria:
- When a user adds an item to the cart and proceeds to checkout, the system should calculate the total price including taxes and shipping.
- The user should be able to select payment methods including credit/debit cards and PayPal.
These examples illustrate how acceptance criteria provide precise conditions that must be met for a feature to be considered successfully implemented.
The Importance of Making Acceptance Criteria Executable
Making acceptance criteria executable holds immense significance in the software development process for several reasons:
-
Accuracy: Executable acceptance criteria allow for precise and automated testing, eliminating human error and subjectivity in the evaluation process.
-
Efficiency: Automation of acceptance criteria testing leads to faster feedback loops, enabling rapid identification and rectification of issues.
-
Client Satisfaction: Executable acceptance criteria ensure that the final product aligns with the client's expectations, thereby enhancing satisfaction and trust.
-
Continuous Integration and Delivery: Executable acceptance criteria seamlessly fit into the CI/CD pipeline, facilitating the rapid and reliable release of new features.
By making acceptance criteria executable, teams can effectively bridge the gap between business requirements and technical implementation, resulting in higher quality software and smoother project execution.
Tools and Techniques for Executing Acceptance Criteria
To achieve the goal of making acceptance criteria executable, various tools and methodologies can be employed. Two prominent approaches include BDD with frameworks like Cucumber and TDD. We will delve into the utilization of Cucumber in the context of Java applications.
Let us Begin to BDD and Cucumber in Java
Behavior-Driven Development (BDD) is a methodology that encourages collaboration between developers, QA, and non-technical or business participants in a software project. It focuses on providing a common understanding of features and their behavior through realistic examples. Cucumber is a widely used BDD tool that supports the implementation of BDD frameworks in various programming languages, including Java.
Example: Setting up Cucumber in a Java Project
To set up Cucumber in a Java project, follow these steps:
-
Step 1: Add the Cucumber dependency in the project's
pom.xml
file:📄snippet.txt<dependency> <groupId>io.cucumber</groupId> <artifactId>cucumber-java</artifactId> <version>{latest_version}</version> <scope>test</scope> </dependency> <dependency> <groupId>io.cucumber</groupId> <artifactId>cucumber-junit</artifactId> <version>{latest_version}</version> <scope>test</scope> </dependency>
-
Step 2: Create a
features
directory in thesrc/test
directory to store feature files. -
Step 3: Create a class to run Cucumber tests, commonly named
CucumberTest
. -
Step 4: Create feature files using the Gherkin language to describe acceptance criteria.
Writing Feature Files
Feature files in Cucumber are written in Gherkin, a plain-text language with a structured syntax that is easy to understand and write. The Gherkin syntax uses specific keywords such as Given
, When
, Then
, And
, and But
to define the behavior of the software in a human-readable format.
Example: Feature File for User Authentication
Feature: User Authentication
Scenario: Valid User Login
Given the user navigates to the login page
When they enter valid credentials (username and password)
Then they should be redirected to the dashboard
The feature file describes the behavior of the system from a user's perspective, aligning with the acceptance criteria for user authentication.
Implementing Step Definitions in Java
Step definitions in Cucumber map the steps defined in the feature files to the actual code that performs the tests. By implementing step definitions in Java, the acceptance criteria are made executable, allowing automated testing based on the defined scenarios.
package stepdefinitions;
import io.cucumber.java.en.Given;
import io.cucumber.java.en.When;
import io.cucumber.java.en.Then;
public class UserAuthenticationSteps {
@Given("the user navigates to the login page")
public void navigateToLoginPage() {
// Code to navigate to the login page
}
@When("they enter valid credentials (username and password)")
public void enterValidCredentials() {
// Code to enter valid credentials
}
@Then("they should be redirected to the dashboard")
public void redirectToDashboard() {
// Assertion code to validate the redirection
}
}
In this example, each step in the feature file is associated with a method in the step definitions class, where the actual interactions with the system and assertions are implemented.
Integrating Executable Acceptance Criteria into the Development Workflow
Integrating executable acceptance criteria into the development and testing processes is vital for ensuring that the defined conditions are met consistently. Continuous Integration and Continuous Deployment (CI/CD) pipelines play a pivotal role in achieving this level of integration.
Continuous Integration and Continuous Deployment (CI/CD)
By integrating executable acceptance criteria with CI/CD pipelines, teams can automate the testing and validation of features before they are deployed to production. This ensures that any code changes meet the specified acceptance criteria and adhere to the defined behavior.
Example: Integrating Cucumber Tests into a CI Pipeline
Using a popular CI tool such as Jenkins, the integration of Cucumber tests into a CI pipeline can be achieved with the following steps:
- Set up a Jenkins job to fetch the code repository and build the project.
- Configure the job to run Cucumber tests as a post-build step.
- Define the conditions for the job to fail if Cucumber tests do not pass, thus preventing the deployment of non-compliant code.
This integration ensures that executable acceptance criteria are an integral part of the development workflow, providing a safety net against the introduction of potential issues.
Challenges and Best Practices
Despite the benefits of making acceptance criteria executable, teams may encounter challenges in implementing and maintaining this practice. Some common challenges include:
- Maintaining Test Suites: As the application evolves, maintaining and updating the test suites to reflect changes in acceptance criteria can be a significant challenge.
- Involving the Whole Team: Ensuring that all team members, including developers, testers, and business analysts, are aligned and actively involved in defining and executing acceptance criteria can be a demanding task.
- Ensuring High-Quality Acceptance Criteria: Creating acceptance criteria that are detailed, clear, and valuable can be a challenge, especially in complex or rapidly changing projects.
To address these challenges, it is essential to adopt best practices such as regular collaboration and communication between team members, automated testing, and a focus on creating concise and unambiguous acceptance criteria.
Case Study: Success Story
XYZ Inc.: Streamlining Feature Delivery with Executable Acceptance Criteria
For XYZ Inc., a leading software development company, the challenge lay in ensuring that the features they delivered were not only as per the client's requirements but also thoroughly tested against precise acceptance criteria.
Challenges Faced:
- Inconsistent understanding of requirements among team members.
- Delayed detection of feature misalignments with business needs.
- Manual testing leading to protracted release cycles.
Implemented Solutions:
- Introduced BDD practices using Cucumber for defining and executing acceptance criteria.
- Conducted regular collaborative sessions involving developers, testers, and business analysts for creating and validating acceptance criteria.
- Integrated Cucumber tests into the CI pipeline to automate acceptance criteria validation.
Benefits Realized:
- Accelerated feedback loops and quicker bug identification.
- Enhanced alignment of developed features with client expectations.
- Significant reduction in release cycle duration.
XYZ Inc.'s success in implementing executable acceptance criteria highlights the potential benefits that can be harnessed through this practice.
Final Thoughts
Making acceptance criteria executable is a pivotal step in ensuring that software solutions precisely meet business needs. By embracing BDD techniques and leveraging tools such as Cucumber, development teams can automate the testing of acceptance criteria, leading to enhanced accuracy, efficiency, and client satisfaction. As the software development landscape continues to evolve, integrating executable acceptance criteria into the development workflow will be imperative for maintaining and improving the quality and agility of software products.
Call to Action: Embrace the practice of making acceptance criteria executable in your software development projects. Explore further resources on BDD, TDD, and Agile methodologies, and start integrating these valuable practices into your development workflow.
Links for Additional Reading:
By adopting these practices and tools, development teams can ensure that their software solutions align closely with business needs, setting the stage for greater success and client satisfaction.
In this step-by-step guide, over the course of this article, we have explored the concept of defining and making acceptance criteria executable in the software development process. We have provided an example of setting up Cucumber in a Java project, writing feature files in Gherkin, and implementing step definitions in Java to make the acceptance criteria executable. We have also discussed the importance of integrating executable acceptance criteria into the development workflow and provided insights into challenges, best practices, and a success story to illustrate the benefits of this approach.