Overcoming Hurdles with Mocking Spring Beans in V2
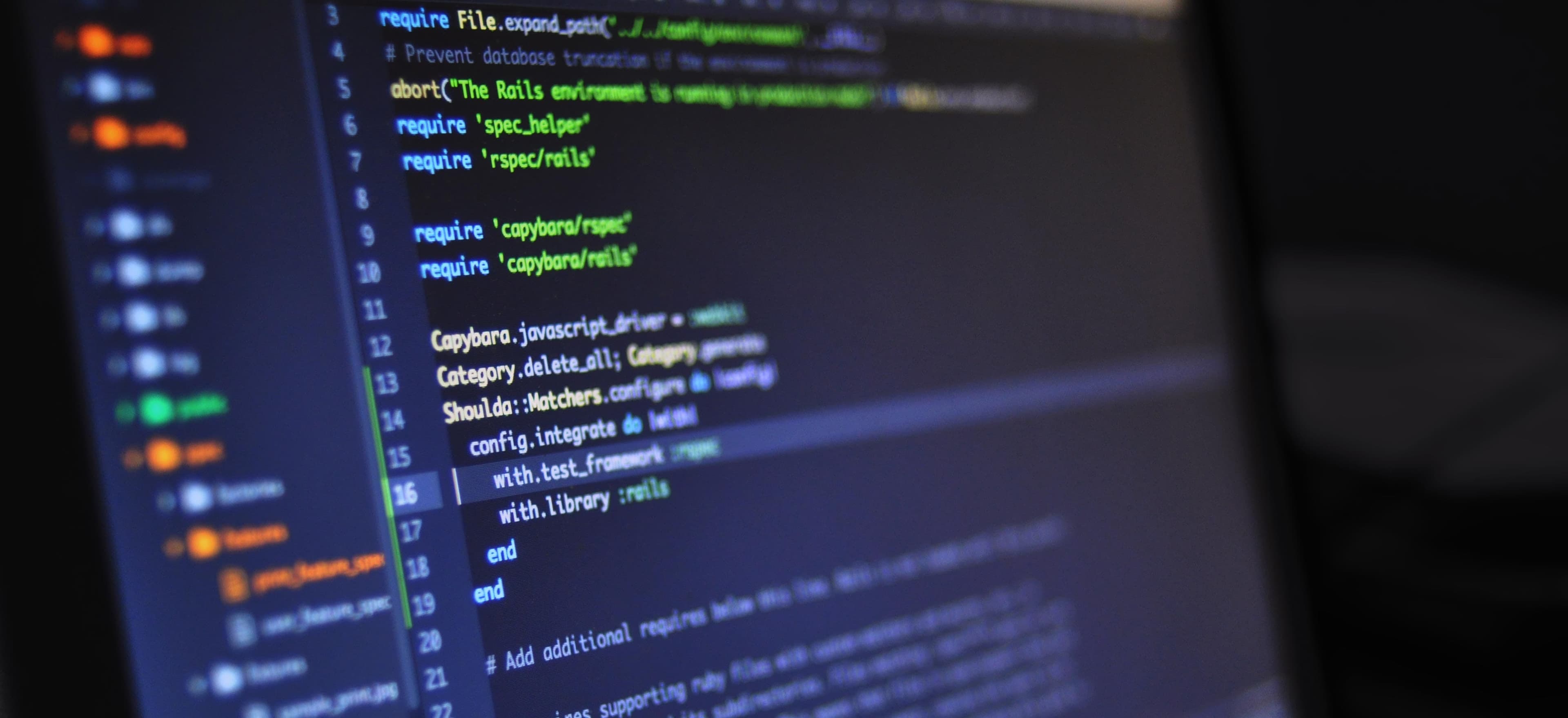
- Published on
Overcoming Hurdles with Mocking Spring Beans in V2
In the world of Java development, Spring Framework stands out as one of the most popular frameworks for building enterprise-level applications. Its dependency injection and inversion of control features make it a go-to choice for many developers. However, when it comes to unit testing Spring-based applications, mocking Spring beans can present a significant challenge.
In this article, we'll delve into the complexities of mocking Spring beans in version 2 of the framework and explore effective strategies to overcome these hurdles. We'll also discuss the importance of mocking, the limitations of traditional mocking frameworks, and how the latest features in Spring can simplify the process.
Why Mock Spring Beans?
Mocking Spring beans is crucial for unit testing. By creating mock implementations of Spring beans, developers can isolate the code under test, simulate the behavior of dependent components, and verify interactions between different modules. This approach enables thorough testing without relying on the actual Spring context, leading to faster and more focused unit tests.
Limitations of Traditional Mocking Frameworks
In earlier versions of Spring, mocking Spring beans often involved using third-party libraries such as Mockito or EasyMock. While these frameworks are powerful and widely used, they have limitations when it comes to mocking certain Spring-specific features, such as annotations and autowiring.
For instance, consider a scenario where a service class depends on a repository bean annotated with @Autowired
. When using a traditional mocking framework, replicating the Spring context and autowiring behavior can be cumbersome and error-prone. This can lead to brittle tests that are tightly coupled to the implementation details of the Spring beans.
Enter Spring 5 and Beyond
With the release of Spring 5 and its subsequent versions, the framework has introduced significant improvements to facilitate mocking of Spring beans. The SpringRunner
and @MockBean
annotations are among the notable additions that streamline the process of mocking beans in unit tests.
SpringRunner and Mockito
The SpringRunner
(or SpringJUnit4ClassRunner
in earlier versions) serves as the test runner that integrates the Spring TestContext Framework with JUnit. When combined with Mockito, it enables the seamless creation of mock implementations for Spring beans.
@RunWith(SpringRunner.class)
public class MyServiceTest {
@MockBean
private MyRepository myRepository;
@Autowired
private MyService myService;
// ...
}
In this example, the @MockBean
annotation instructs the SpringRunner
to mock the MyRepository
bean, which is then autowired into the MyServiceTest
class. This approach simplifies the creation of mock beans and eliminates the need for explicit mock setup, as Mockito handles the mock behavior.
Benefits of @MockBean
The @MockBean
annotation offers several benefits over traditional mocking frameworks. It seamlessly integrates with the Spring context, allowing the mocked beans to participate in the dependency injection mechanism. This means that any beans or components reliant on the mocked bean will receive the mock instance without manual wiring.
Furthermore, @MockBean
provides concise and expressive syntax for defining mock beans within the test class. This leads to clearer and more maintainable tests, as the intent of mocking specific beans is explicitly declared within the test code.
Advanced Mocking Scenarios
While the @MockBean
annotation caters to most mocking requirements, there are advanced scenarios where customizing the mock behavior becomes necessary. In such cases, leveraging the Mockito.when
and Mockito.doReturn
methods can enhance the flexibility of mock bean setup.
@Test
public void testCustomMockBehavior() {
// Customizing mock behavior using Mockito
Mockito.when(myRepository.findById(ArgumentMatchers.anyLong()))
.thenReturn(Optional.of(new MyEntity()));
// Invoke the service method that interacts with the mocked repository
MyEntity result = myService.findById(123L);
// Assertions and verifications
// ...
}
By using Mockito.when
or Mockito.doReturn
, developers can define specific behaviors for the mocked bean's methods, such as returning custom responses or throwing exceptions. This level of control is especially valuable when testing complex scenarios and edge cases.
Future-Proofing Tests with @MockBean
When writing unit tests for Spring-based applications, the use of @MockBean
not only addresses the current mocking challenges but also future-proofs the test suite against potential Spring bean modifications. As the application evolves and new beans are introduced, the existing tests remain resilient, as the @MockBean
approach adapts to changes in the Spring context.
A Final Look
Mocking Spring beans in version 2 of the framework presents a more streamlined and intuitive process, thanks to the enhancements introduced in Spring 5 and beyond. By embracing the @MockBean
annotation and leveraging Mockito in conjunction with the SpringRunner, developers can efficiently create effective unit tests that accurately reflect the real-world behavior of Spring-based components.
In summary, the improved support for mocking Spring beans in Spring V2 empowers developers to write robust and maintainable unit tests, ultimately contributing to the overall quality and reliability of Spring-based applications. With these advancements, the once formidable hurdles of mocking Spring beans have become more manageable, reinforcing Spring's position as a leading framework for enterprise Java development.
For more in-depth insights and best practices regarding Spring Framework, visit the official Spring Documentation.
Now, armed with an understanding of the advancements in mocking Spring beans, it's time to elevate your unit testing game and unlock the full potential of Spring-based applications. Happy coding and testing!